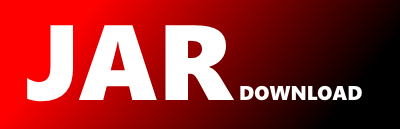
net.welen.jmole.protocols.zabbix.JMoleMetricsProvider Maven / Gradle / Ivy
package net.welen.jmole.protocols.zabbix;
/*
* #%L
* JMole, https://bitbucket.org/awelen/jmole
* %%
* Copyright (C) 2015 - 2020 Anders Welén, [email protected]
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.net.URLEncoder;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.management.AttributeNotFoundException;
import javax.management.InstanceNotFoundException;
import javax.management.MBeanException;
import javax.management.ObjectName;
import javax.management.ReflectionException;
import net.welen.jmole.Configuration;
import net.welen.jmole.JMole;
import net.welen.jmole.threshold.Threshold;
import net.welen.jmole.threshold.ThresholdValues;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import com.quigley.zabbixj.metrics.MetricsException;
import com.quigley.zabbixj.metrics.MetricsKey;
import com.quigley.zabbixj.metrics.MetricsProvider;
public class JMoleMetricsProvider implements MetricsProvider {
private final static Logger LOG = Logger.getLogger(JMoleMetricsProvider.class.getName());
private JMole jmole = null;
public JMoleMetricsProvider(JMole jmole) {
this.jmole = jmole;
}
public Object getValue(MetricsKey metricKey) throws MetricsException {
String key = metricKey.getKey();
LOG.log(Level.FINE, "Key: " + key);
// Zabbix Discovery?
if (key.equals("discovery")) {
try {
return getZabbixDiscovery();
} catch (Throwable t) {
LOG.log(Level.SEVERE, "Couldn't get Zabbix discovery", t);
throw new MetricsException(t);
}
}
// Zabbix ping
if (key.equals("ping")) {
return 1;
}
// Zabbix fetching values?
if (key.equals("fetch")) {
try {
return getZabbixFetch(metricKey);
} catch (Exception e) {
throw new MetricsException(e);
}
}
LOG.log(Level.WARNING, "Unknown key: " + key);
throw new MetricsException("Unknown key: " + key);
}
private String getZabbixFetch(MetricsKey metricKey) throws InstanceNotFoundException, AttributeNotFoundException, ReflectionException, MBeanException, UnsupportedEncodingException {
String path = metricKey.getParameters()[0];
String paths[] = path.split("/");
if (paths.length != 3) {
LOG.log(Level.SEVERE, "Incorrect path: " + path);
throw new MetricsException("Incorrect path: " + path);
}
Object answer = jmole.collectMeasurement(URLDecoder.decode(paths[0], "UTF-8"),
URLDecoder.decode(paths[1], "UTF-8"),
URLDecoder.decode(paths[2], "UTF-8"));
LOG.log(Level.FINE, "Returning \"" + answer.toString() + "\" for: " + metricKey.getKey());
return answer.toString();
}
private String getZabbixDiscovery() throws JSONException, InstanceNotFoundException, ReflectionException, AttributeNotFoundException, MBeanException, UnsupportedEncodingException {
JSONObject jsonObject = new JSONObject();
JSONArray jsonArray = new JSONArray();
// Get all measurements
for (Configuration configuration : jmole.getConfiguration()) {
String category = configuration.getPresentationInformation().getCategory();
for (ObjectName objectName : configuration.getMBeanFinder().getMatchingObjectNames()) {
String name = configuration.getMBeanCollector().getConstructedName(objectName);
for (String attribute : configuration.getMBeanCollector().getAttributes()) {
JSONObject tmpObject = new JSONObject();
tmpObject.put("{#JMOLEPATH}",
URLEncoder.encode(category, "UTF-8") + "/" +
URLEncoder.encode(name, "UTF-8") + "/" +
URLEncoder.encode(attribute, "UTF-8"));
tmpObject.put("{#JMOLECATEGORY}", category);
tmpObject.put("{#JMOLENAME}", name);
tmpObject.put("{#JMOLEATTRIBUTE}", attribute);
// Unit is not used until Zabbix can pick it up in the template
tmpObject.put("{#JMOLEUNIT}", configuration.getPresentationInformation().getUnit());
tmpObject.put("{#JMOLETITLE}", configuration.getMBeanCollector().getConstructedName(objectName));
tmpObject.put("{#JMOLEATTRIBUTEDESCRIPTION}", configuration.getPresentationInformation().translateAttributeLabel(attribute));
Threshold threshold = configuration.getThresholds().get(attribute);
if (threshold != null) {
ThresholdValues individualValues = threshold.getIndividualThresholds().get(configuration.getMBeanCollector().getConstructedName(objectName));
String low;
String high;
// Warnings
if (individualValues == null) {
low = threshold.getWarningLowThreshold();
high = threshold.getWarningHighThreshold();
} else {
low = individualValues.getWarningLowThreshold();
high = individualValues.getWarningHighThreshold();
}
low = Threshold.calculateThreshold(low, configuration.getMBeanCollector(), objectName, attribute);
if (low.length() > 0) {
tmpObject.put("{#JMOLEWARNLOW}", low);
}
high = Threshold.calculateThreshold(high, configuration.getMBeanCollector(), objectName, attribute);
if (high.length() > 0) {
tmpObject.put("{#JMOLEWARNHIGH}", high);
}
// Critical
if (individualValues == null) {
low = threshold.getCriticalLowThreshold();
high = threshold.getCriticalHighThreshold();
} else {
low = individualValues.getCriticalLowThreshold();
high = individualValues.getCriticalHighThreshold();
}
low = Threshold.calculateThreshold(low, configuration.getMBeanCollector(), objectName, attribute);
if (low.length() > 0) {
tmpObject.put("{#JMOLECRITICALLOW}", low);
}
high = Threshold.calculateThreshold(high, configuration.getMBeanCollector(), objectName, attribute);
if (high.length() > 0) {
tmpObject.put("{#JMOLECRITICALHIGH}", high);
}
}
jsonArray.put(tmpObject);
}
}
}
jsonObject.put("data", jsonArray);
String answer = jsonObject.toString();
LOG.log(Level.FINE, "Returning Zabbix discovery: " + answer);
return answer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy