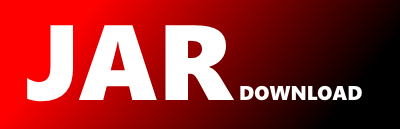
net.weweave.commerce.DomainList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of key Show documentation
Show all versions of key Show documentation
License Key Validator for weweave Commerce.
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy