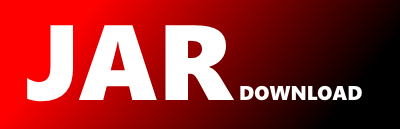
net.wicp.tams.component.components.ComboBox Maven / Gradle / Ivy
package net.wicp.tams.component.components;
import org.apache.tapestry5.BindingConstants;
import org.apache.tapestry5.annotations.Import;
import org.apache.tapestry5.annotations.Parameter;
import org.apache.tapestry5.ioc.annotations.Inject;
import org.apache.tapestry5.ioc.annotations.InjectService;
import org.apache.tapestry5.ioc.annotations.Symbol;
import org.apache.tapestry5.json.JSONArray;
import org.apache.tapestry5.json.JSONObject;
import net.wicp.tams.commons.apiext.StringUtil;
import net.wicp.tams.component.SymbolConstantsCus;
import net.wicp.tams.component.services.ISupportedLocales;
/*****
* 下拉列表
*
* @author Administrator
*
*/
@Import(stack = "easyuistack")
public class ComboBox extends ValidateBox {
protected final static String textFieldVarName="textField";
protected final static String valueFieldVarName="valueField";
/****
* 组件的宽度
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private int width;
@Inject
@Symbol(SymbolConstantsCus.INPUT_WIDTH)
private int inputWidth;
/****
* 组件的高度
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private int height;
/****
* 下拉列表panel的宽度
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private int panelWidth;
/****
* 下拉列表panel的高度
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private int panelHeight;
/*****
* 是否多选(在Combo组件暂时没用到)
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private boolean multiple;
/****
* 当是多选时返回文本的分隔符(在Combo组件暂时没用到)
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private String separator;
/***
* 定义是否可用
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private boolean disabled;
/***
* 定义是否只读
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private boolean readonly;
/****
* 下拉列表是否有像下的箭头
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private boolean hasDownArrow;
/****
* option的label字段,如果是子组件由各子组件提供,不需要再设置此对象。 默认值为:text
*/
@Parameter(required = false, value = "var:textField", defaultPrefix = BindingConstants.LITERAL)
private String textField;
/****
* option的value字段,如果是子组件由各子组件提供,不需要再设置此对象。默认值为:value
*/
@Parameter(required = false, value = "var:valueField", defaultPrefix = BindingConstants.LITERAL)
private String valueField;
/***
* 取数据的地址, 路径不用带contextpath.
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private String url;
/***
* 与url配套使用,在url后面添加查询参数
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private JSONObject urlParam;
/****
* 格式化显示text的函数
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private String formatter;
/****
* 过滤结果集函数
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private String loadFilter;
/****
* 直接给出下拉列表数据,如果有url则此字段无效
*/
@Parameter(required = false, defaultPrefix = BindingConstants.PROP)
private JSONArray data;
/****
* 级联父下拉列表的ID
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private String parent;
/****
* 当用户选择了一个选项时触发
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private String selectHandle;
/****
* 当值改变时触发
*/
@Parameter(required = false, defaultPrefix = BindingConstants.LITERAL)
private String changeHandle;
@InjectService("locales")
protected ISupportedLocales supportedLocales;
@Override
protected void packParms(JSONObject spec) {
super.packParms(spec);
spec.put("class", "combobox");
spec.put("ishtml", false);
if (resources.isBound("formatter"))
spec.put("formatter", formatter);
if (resources.isBound("loadFilter")) {
spec.put("loadFilter", loadFilter);
}
JSONObject params = spec.getJSONObject("params");
if (resources.isBound("width"))
params.put("width", width);
else {
params.put("width", inputWidth);// 默认为120px宽度
}
params.put("fit", false);
if (resources.isBound("height"))
params.put("height", height);
if (resources.isBound("panelWidth"))
params.put("panelWidth", panelWidth);
if (resources.isBound("panelHeight"))
params.put("panelHeight", panelHeight);
if (resources.isBound("multiple"))
params.put("multiple", multiple);
if (resources.isBound("separator"))
params.put("separator", separator);
if (resources.isBound("disabled"))
params.put("disabled", disabled);
if (resources.isBound("readonly"))
params.put("readonly", readonly);
if (resources.isBound("hasDownArrow"))
params.put("hasDownArrow", hasDownArrow);
try {
if(!resources.isBound("textField") || StringUtil.isNull(resources.getRenderVariable(textFieldVarName))){//如果没有绑定或者子系统没有传值过来就设置默认值
resources.storeRenderVariable(textFieldVarName, "text");
}
} catch (Exception e) {
resources.storeRenderVariable(textFieldVarName, "text");
}
params.put("textField", textField==null?"text":textField);
try {
if(!resources.isBound("valueField") || StringUtil.isNull(resources.getRenderVariable(valueFieldVarName))){//如果没有绑定或者子系统没有传值过来就设置默认值
resources.storeRenderVariable(valueFieldVarName, "value");
}
} catch (Exception e) {
resources.storeRenderVariable(valueFieldVarName, "value");
}
params.put("valueField", valueField==null?"value":valueField);
if (resources.isBound("parent")) {// 需要进行级联显示
spec.put("parent", parent);
if (resources.isBound("url"))
spec.put("url", url);
if (resources.isBound("urlParam"))
spec.put("urlParam", urlParam);
}
if (resources.isBound("selectHandle"))
spec.put("selectHandle", selectHandle);
if (resources.isBound("changeHandle"))
spec.put("changeHandle", changeHandle);
if (resources.isBound("data") || data != null) {
params.put("data", data);
} else {
String urlTrue = url;
if (resources.isBound("url")) {
if (resources.isBound("urlparam")) {// 查询参数
StringBuffer buffer = new StringBuffer("?");
for (String key : urlParam.keys()) {
buffer.append(key + "=" + urlParam.getString(key) + "&");
}
urlTrue = url + buffer.substring(0, buffer.length() - 1);
}
}
params.put("url", supportedLocales.buildUrl(urlTrue));
}
}
/***
* 子类可以配置数据
*
* @param data
*/
protected void setData(JSONArray data) {
this.data = data;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy