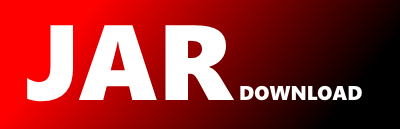
net.wicp.tams.cas.dao.SysResourceMapper Maven / Gradle / Ivy
package net.wicp.tams.cas.dao;
import java.util.List;
import net.wicp.tams.cas.bean.models.SysResource;
import net.wicp.tams.cas.bean.models.SysResourceExample;
import org.apache.ibatis.annotations.Delete;
import org.apache.ibatis.annotations.Insert;
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.ResultMap;
import org.apache.ibatis.annotations.Select;
import org.apache.ibatis.annotations.Update;
public interface SysResourceMapper {
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
long countByExample(SysResourceExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
int deleteByExample(SysResourceExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
@Delete({ "delete from sys_resource", "where id = #{id,jdbcType=BIGINT}" })
int deleteByPrimaryKey(Long id);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
@Insert({ "insert into sys_resource (id, res_code, ", "res_name, res_value, ", "res_type, icon, res_level, ",
"is_edit, parent_id, ", "show_order, remark, ", "is_sync, bound1, ", "bound2, bound3, ", "bound4, bound5, ",
"bound6, bound7, ", "bound8, bound9)", "values (#{id,jdbcType=BIGINT}, #{resCode,jdbcType=VARCHAR}, ",
"#{resName,jdbcType=VARCHAR}, #{resValue,jdbcType=VARCHAR}, ",
"#{resType,jdbcType=VARCHAR}, #{icon,jdbcType=VARCHAR}, #{resLevel,jdbcType=INTEGER}, ",
"#{isEdit,jdbcType=VARCHAR}, #{parentId,jdbcType=BIGINT}, ",
"#{showOrder,jdbcType=BIGINT}, #{remark,jdbcType=VARCHAR}, ",
"#{isSync,jdbcType=VARCHAR}, #{bound1,jdbcType=VARCHAR}, ",
"#{bound2,jdbcType=VARCHAR}, #{bound3,jdbcType=VARCHAR}, ",
"#{bound4,jdbcType=VARCHAR}, #{bound5,jdbcType=VARCHAR}, ",
"#{bound6,jdbcType=VARCHAR}, #{bound7,jdbcType=VARCHAR}, ",
"#{bound8,jdbcType=VARCHAR}, #{bound9,jdbcType=VARCHAR})" })
int insert(SysResource record);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
int insertSelective(SysResource record);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
List selectByExample(SysResourceExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
@Select({ "select", "id, res_code, res_name, res_value, res_type, icon, res_level, is_edit, parent_id, ",
"show_order, remark, is_sync, bound1, bound2, bound3, bound4, bound5, bound6, ", "bound7, bound8, bound9",
"from sys_resource", "where id = #{id,jdbcType=BIGINT}" })
@ResultMap("net.wicp.tams.cas.dao.SysResourceMapper.BaseResultMap")
SysResource selectByPrimaryKey(Long id);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
int updateByExampleSelective(@Param("record") SysResource record, @Param("example") SysResourceExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
int updateByExample(@Param("record") SysResource record, @Param("example") SysResourceExample example);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
int updateByPrimaryKeySelective(SysResource record);
/**
* This method was generated by MyBatis Generator. This method corresponds to the database table sys_resource
*
*/
@Update({ "update sys_resource", "set res_code = #{resCode,jdbcType=VARCHAR},",
"res_name = #{resName,jdbcType=VARCHAR},", "res_value = #{resValue,jdbcType=VARCHAR},",
"res_type = #{resType,jdbcType=VARCHAR},", "icon = #{icon,jdbcType=VARCHAR},",
"res_level = #{resLevel,jdbcType=INTEGER},", "is_edit = #{isEdit,jdbcType=VARCHAR},",
"parent_id = #{parentId,jdbcType=BIGINT},", "show_order = #{showOrder,jdbcType=BIGINT},",
"remark = #{remark,jdbcType=VARCHAR},", "is_sync = #{isSync,jdbcType=VARCHAR},",
"bound1 = #{bound1,jdbcType=VARCHAR},", "bound2 = #{bound2,jdbcType=VARCHAR},",
"bound3 = #{bound3,jdbcType=VARCHAR},", "bound4 = #{bound4,jdbcType=VARCHAR},",
"bound5 = #{bound5,jdbcType=VARCHAR},", "bound6 = #{bound6,jdbcType=VARCHAR},",
"bound7 = #{bound7,jdbcType=VARCHAR},", "bound8 = #{bound8,jdbcType=VARCHAR},",
"bound9 = #{bound9,jdbcType=VARCHAR}", "where id = #{id,jdbcType=BIGINT}" })
int updateByPrimaryKey(SysResource record);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy