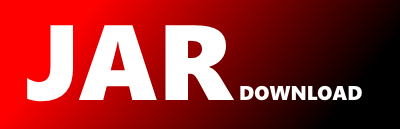
net.yetamine.lang.creational.Factory Maven / Gradle / Ivy
Show all versions of net.yetamine.lang Show documentation
/*
* Copyright 2016 Yetamine
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.yetamine.lang.creational;
import java.util.Objects;
import java.util.function.Function;
/**
* An interface for factories and builders.
*
* @param
* the type of the product
*/
@FunctionalInterface
public interface Factory {
/**
* Provides a new instance of the product.
*
*
* Technically, the result does not have to be a brand new instance if the
* result is immutable and may be shared. However, the result must be such
* an instance that it can be used by multiple clients without their mutual
* interference.
*
* @return a new instance of the product
*/
T build();
/**
* Makes a factory which uses a template object as the prototype for making
* more instances.
*
* @param
* the type of the template
* @param
* the type of the product
* @param template
* the template for the builder. It must not be {@code null}.
* @param builder
* the builder which takes a template object and returns an
* independent copy of it. It must not be {@code null}.
*
* @return a factory using a template object
*/
static Factory prototype(U template, Function super U, ? extends T> builder) {
Objects.requireNonNull(template);
Objects.requireNonNull(builder);
return () -> builder.apply(template);
}
}