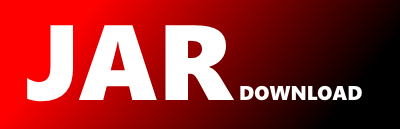
net.yetamine.lang.exceptions.ThrowingCallable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of net.yetamine.lang Show documentation
Show all versions of net.yetamine.lang Show documentation
Small extensions for the core Java language libraries.
The newest version!
/*
* Copyright 2016 Yetamine
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.yetamine.lang.exceptions;
import java.util.Objects;
import java.util.concurrent.Callable;
import java.util.function.Supplier;
/**
* An extension of {@link Callable} that throws a specific exception class,
* which is declared by a type parameter for the interface as well.
*
* @param
* the type of the result
* @param
* the type of the exception that the resource bound to this
* interface may throw
*/
@FunctionalInterface
public interface ThrowingCallable extends Callable {
/**
* @see java.util.concurrent.Callable#call()
*/
V call() throws X;
/**
* Returns an instance that uses a dedicated special handler for taking care
* of any {@link InterruptedException} which may raise when invoking this
* instance.
*
* @param handler
* the handler to use. It must not be {@code null}.
*
* @return the guarding instance
*/
default ThrowingCallable whenInterrupted(ThrowingConsumer super InterruptedException, ? extends X> handler) {
Objects.requireNonNull(handler);
@SuppressWarnings("unchecked") // When using -Xlint:unchecked
final ThrowingCallable result = () -> {
try {
return call();
} catch (Exception e) {
if (e instanceof InterruptedException) {
handler.accept((InterruptedException) e);
}
throw e;
}
};
return result;
}
/**
* Returns the given callable.
*
* @param
* the type of the result
* @param
* the type of the exception that the operation may throw
* @param callable
* the callable to return. It must not be {@code null}.
*
* @return the callable
*/
@SuppressWarnings("unchecked")
static ThrowingCallable from(ThrowingCallable extends V, ? extends X> callable) {
return (ThrowingCallable) callable;
}
/**
* Returns a {@link Supplier} that invokes the given instance and relays all
* unchecked exceptions (and errors) to the caller, while throwing
* {@link UncheckedException} for any checked exception.
*
* @param
* the type of the result
* @param callable
* the callable to use. It must not be {@code null}.
*
* @return the supplier
*/
static Supplier rethrowing(ThrowingCallable extends V, ?> callable) {
Objects.requireNonNull(callable);
return () -> {
try {
return callable.call();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new UncheckedException(e);
}
};
}
/**
* Returns a {@link Supplier} that invokes the given instance and relays all
* errors to the caller, while throwing {@link UncheckedException} for any
* other exception.
*
* @param
* the type of the result
* @param callable
* the callable to use. It must not be {@code null}.
*
* @return the supplier
*/
static Supplier enclosing(ThrowingCallable extends V, ?> callable) {
Objects.requireNonNull(callable);
return () -> {
try {
return callable.call();
} catch (Exception e) {
throw new UncheckedException(e);
}
};
}
/**
* Returns a {@link Supplier} that invokes the given instance and throws
* {@link UncheckedException} encapsulating any exception or error.
*
* @param
* the type of the result
* @param callable
* the callable to use. It must not be {@code null}.
*
* @return the supplier
*/
static Supplier guarding(ThrowingCallable extends V, ?> callable) {
Objects.requireNonNull(callable);
return () -> {
try {
return callable.call();
} catch (Throwable t) {
throw new UncheckedException(t);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy