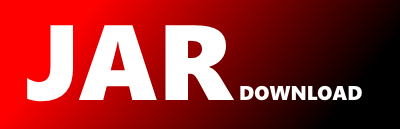
net.yetamine.lang.exceptions.ThrowingConsumer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of net.yetamine.lang Show documentation
Show all versions of net.yetamine.lang Show documentation
Small extensions for the core Java language libraries.
The newest version!
/*
* Copyright 2017 Yetamine
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.yetamine.lang.exceptions;
import java.util.Objects;
import java.util.function.Consumer;
/**
* A variant of {@link Consumer} that throws a specific exception class, which
* is declared by the type parameter.
*
* @param
* the type of the argument
* @param
* the type of the exception that the operation may throw
*/
@FunctionalInterface
public interface ThrowingConsumer {
/**
* Executes the operation.
*
* @param arg
* the argument of the operation
*
* @throws X
* if the operation fails
*/
void accept(T arg) throws X;
/**
* Returns a {@link Consumer} that invokes this instance to process its
* argument and uses the given handler to handle any exceptions.
*
*
* This method provides a bridge from methods throwing checked exceptions; a
* typical use might look like:
*
*
* collection.forEach(throwingConsumer.handled(UncheckedException::raise))
*
*
* @param handler
* the exception handler. It must not be {@code null}.
*
* @return the consumer
*/
default Consumer guarded(ThrowingConsumer super Throwable, ? extends RuntimeException> handler) {
return arg -> {
try {
accept(arg);
} catch (Throwable t) {
handler.accept(t);
}
};
}
/**
* Returns the given consumer.
*
* @param
* the type of the argument
* @param
* the type of the exception that the operation may throw
* @param consumer
* the consumer to return. It must not be {@code null}.
*
* @return the consumer
*/
@SuppressWarnings("unchecked")
static ThrowingConsumer from(ThrowingConsumer super T, ? extends X> consumer) {
return (ThrowingConsumer) consumer;
}
/**
* Returns a {@link Consumer} that invokes the given instance to process its
* argument and relays all unchecked exceptions (and errors) to the caller,
* while throwing {@link UncheckedException} for any checked exception.
*
* @param
* the type of the argument
* @param consumer
* the consumer to use. It must not be {@code null}.
*
* @return the consumer
*/
static Consumer rethrowing(ThrowingConsumer super T, ?> consumer) {
Objects.requireNonNull(consumer);
return arg -> {
try {
consumer.accept(arg);
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new UncheckedException(e);
}
};
}
/**
* Returns a {@link Consumer} that invokes the given instance to process its
* argument and relays all errors to the caller, while throwing
* {@link UncheckedException} for any other exception.
*
* @param
* the type of the argument
* @param consumer
* the consumer to use. It must not be {@code null}.
*
* @return the consumer
*/
static Consumer enclosing(ThrowingConsumer super T, ?> consumer) {
Objects.requireNonNull(consumer);
return arg -> {
try {
consumer.accept(arg);
} catch (Exception e) {
throw new UncheckedException(e);
}
};
}
/**
* Returns a {@link Consumer} that invokes the given instance to process its
* argument and throws {@link UncheckedException} encapsulating any
* exception or error.
*
* @param
* the type of the argument
* @param consumer
* the consumer to use. It must not be {@code null}.
*
* @return the consumer
*/
static Consumer guarding(ThrowingConsumer super T, ?> consumer) {
Objects.requireNonNull(consumer);
return arg -> {
try {
consumer.accept(arg);
} catch (Throwable t) {
throw new UncheckedException(t);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy