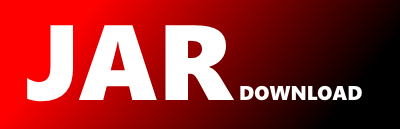
net.yudichev.jiotty.common.lang.throttling.ThresholdThrottlingConsumerModule Maven / Gradle / Ivy
package net.yudichev.jiotty.common.lang.throttling;
import com.google.common.reflect.TypeParameter;
import com.google.common.reflect.TypeToken;
import com.google.inject.Key;
import com.google.inject.TypeLiteral;
import com.google.inject.assistedinject.FactoryModuleBuilder;
import net.yudichev.jiotty.common.inject.*;
import net.yudichev.jiotty.common.lang.TypedBuilder;
import java.util.function.Consumer;
import static com.google.common.base.Preconditions.checkNotNull;
import static net.yudichev.jiotty.common.inject.SpecifiedAnnotation.forNoAnnotation;
public final class ThresholdThrottlingConsumerModule extends BaseLifecycleComponentModule implements ExposedKeyModule> {
private final Key> exposedKey;
private final TypeToken valueType;
private ThresholdThrottlingConsumerModule(TypeToken valueType, SpecifiedAnnotation specifiedAnnotation) {
this.valueType = checkNotNull(valueType);
exposedKey = specifiedAnnotation.specify(asReifiedTypeLiteral(new TypeToken>() {}));
}
@Override
public Key> getExposedKey() {
return exposedKey;
}
public static ValueChoiceBuilder builder() {
return new ValueChoiceBuilder();
}
@Override
protected void configure() {
install(new FactoryModuleBuilder()
.implement(asReifiedTypeLiteral(new TypeToken>() {}), asReifiedTypeLiteral(new TypeToken>() {}))
.build(exposedKey));
expose(exposedKey);
}
private TypeLiteral asReifiedTypeLiteral(TypeToken typeToken) {
return TypeLiterals.asTypeLiteral(typeToken.where(new TypeParameter() {}, valueType));
}
public static final class ValueChoiceBuilder {
public Builder setValueType(Class valueType) {
return setValueType(TypeToken.of(valueType));
}
@SuppressWarnings("MethodMayBeStatic")
private Builder setValueType(TypeToken valueType) {
return new Builder<>(valueType);
}
}
public static final class Builder implements TypedBuilder>>, HasWithAnnotation {
private final TypeToken valueType;
private SpecifiedAnnotation specifiedAnnotation = forNoAnnotation();
private Builder(TypeToken valueType) {
this.valueType = checkNotNull(valueType);
}
@Override
public Builder withAnnotation(SpecifiedAnnotation specifiedAnnotation) {
this.specifiedAnnotation = checkNotNull(specifiedAnnotation);
return this;
}
@Override
public ExposedKeyModule> build() {
return new ThresholdThrottlingConsumerModule<>(valueType, specifiedAnnotation);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy