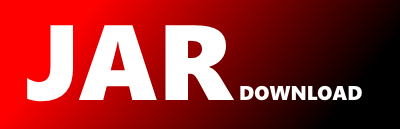
com.google.assistant.embedded.v1alpha2.AssistResponseOrBuilder Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: embedded_assistant.proto
package com.google.assistant.embedded.v1alpha2;
public interface AssistResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.assistant.embedded.v1alpha2.AssistResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* *Output-only* Indicates the type of event.
*
*
* .google.assistant.embedded.v1alpha2.AssistResponse.EventType event_type = 1;
* @return The enum numeric value on the wire for eventType.
*/
int getEventTypeValue();
/**
*
* *Output-only* Indicates the type of event.
*
*
* .google.assistant.embedded.v1alpha2.AssistResponse.EventType event_type = 1;
* @return The eventType.
*/
com.google.assistant.embedded.v1alpha2.AssistResponse.EventType getEventType();
/**
*
* *Output-only* The audio containing the Assistant's response to the query.
*
*
* .google.assistant.embedded.v1alpha2.AudioOut audio_out = 3;
* @return Whether the audioOut field is set.
*/
boolean hasAudioOut();
/**
*
* *Output-only* The audio containing the Assistant's response to the query.
*
*
* .google.assistant.embedded.v1alpha2.AudioOut audio_out = 3;
* @return The audioOut.
*/
com.google.assistant.embedded.v1alpha2.AudioOut getAudioOut();
/**
*
* *Output-only* The audio containing the Assistant's response to the query.
*
*
* .google.assistant.embedded.v1alpha2.AudioOut audio_out = 3;
*/
com.google.assistant.embedded.v1alpha2.AudioOutOrBuilder getAudioOutOrBuilder();
/**
*
* *Output-only* Contains the Assistant's visual response to the query.
*
*
* .google.assistant.embedded.v1alpha2.ScreenOut screen_out = 4;
* @return Whether the screenOut field is set.
*/
boolean hasScreenOut();
/**
*
* *Output-only* Contains the Assistant's visual response to the query.
*
*
* .google.assistant.embedded.v1alpha2.ScreenOut screen_out = 4;
* @return The screenOut.
*/
com.google.assistant.embedded.v1alpha2.ScreenOut getScreenOut();
/**
*
* *Output-only* Contains the Assistant's visual response to the query.
*
*
* .google.assistant.embedded.v1alpha2.ScreenOut screen_out = 4;
*/
com.google.assistant.embedded.v1alpha2.ScreenOutOrBuilder getScreenOutOrBuilder();
/**
*
* *Output-only* Contains the action triggered by the query with the
* appropriate payloads and semantic parsing.
*
*
* .google.assistant.embedded.v1alpha2.DeviceAction device_action = 6;
* @return Whether the deviceAction field is set.
*/
boolean hasDeviceAction();
/**
*
* *Output-only* Contains the action triggered by the query with the
* appropriate payloads and semantic parsing.
*
*
* .google.assistant.embedded.v1alpha2.DeviceAction device_action = 6;
* @return The deviceAction.
*/
com.google.assistant.embedded.v1alpha2.DeviceAction getDeviceAction();
/**
*
* *Output-only* Contains the action triggered by the query with the
* appropriate payloads and semantic parsing.
*
*
* .google.assistant.embedded.v1alpha2.DeviceAction device_action = 6;
*/
com.google.assistant.embedded.v1alpha2.DeviceActionOrBuilder getDeviceActionOrBuilder();
/**
*
* *Output-only* This repeated list contains zero or more speech recognition
* results that correspond to consecutive portions of the audio currently
* being processed, starting with the portion corresponding to the earliest
* audio (and most stable portion) to the portion corresponding to the most
* recent audio. The strings can be concatenated to view the full
* in-progress response. When the speech recognition completes, this list
* will contain one item with `stability` of `1.0`.
*
*
* repeated .google.assistant.embedded.v1alpha2.SpeechRecognitionResult speech_results = 2;
*/
java.util.List
getSpeechResultsList();
/**
*
* *Output-only* This repeated list contains zero or more speech recognition
* results that correspond to consecutive portions of the audio currently
* being processed, starting with the portion corresponding to the earliest
* audio (and most stable portion) to the portion corresponding to the most
* recent audio. The strings can be concatenated to view the full
* in-progress response. When the speech recognition completes, this list
* will contain one item with `stability` of `1.0`.
*
*
* repeated .google.assistant.embedded.v1alpha2.SpeechRecognitionResult speech_results = 2;
*/
com.google.assistant.embedded.v1alpha2.SpeechRecognitionResult getSpeechResults(int index);
/**
*
* *Output-only* This repeated list contains zero or more speech recognition
* results that correspond to consecutive portions of the audio currently
* being processed, starting with the portion corresponding to the earliest
* audio (and most stable portion) to the portion corresponding to the most
* recent audio. The strings can be concatenated to view the full
* in-progress response. When the speech recognition completes, this list
* will contain one item with `stability` of `1.0`.
*
*
* repeated .google.assistant.embedded.v1alpha2.SpeechRecognitionResult speech_results = 2;
*/
int getSpeechResultsCount();
/**
*
* *Output-only* This repeated list contains zero or more speech recognition
* results that correspond to consecutive portions of the audio currently
* being processed, starting with the portion corresponding to the earliest
* audio (and most stable portion) to the portion corresponding to the most
* recent audio. The strings can be concatenated to view the full
* in-progress response. When the speech recognition completes, this list
* will contain one item with `stability` of `1.0`.
*
*
* repeated .google.assistant.embedded.v1alpha2.SpeechRecognitionResult speech_results = 2;
*/
java.util.List extends com.google.assistant.embedded.v1alpha2.SpeechRecognitionResultOrBuilder>
getSpeechResultsOrBuilderList();
/**
*
* *Output-only* This repeated list contains zero or more speech recognition
* results that correspond to consecutive portions of the audio currently
* being processed, starting with the portion corresponding to the earliest
* audio (and most stable portion) to the portion corresponding to the most
* recent audio. The strings can be concatenated to view the full
* in-progress response. When the speech recognition completes, this list
* will contain one item with `stability` of `1.0`.
*
*
* repeated .google.assistant.embedded.v1alpha2.SpeechRecognitionResult speech_results = 2;
*/
com.google.assistant.embedded.v1alpha2.SpeechRecognitionResultOrBuilder getSpeechResultsOrBuilder(
int index);
/**
*
* *Output-only* Contains output related to the user's query.
*
*
* .google.assistant.embedded.v1alpha2.DialogStateOut dialog_state_out = 5;
* @return Whether the dialogStateOut field is set.
*/
boolean hasDialogStateOut();
/**
*
* *Output-only* Contains output related to the user's query.
*
*
* .google.assistant.embedded.v1alpha2.DialogStateOut dialog_state_out = 5;
* @return The dialogStateOut.
*/
com.google.assistant.embedded.v1alpha2.DialogStateOut getDialogStateOut();
/**
*
* *Output-only* Contains output related to the user's query.
*
*
* .google.assistant.embedded.v1alpha2.DialogStateOut dialog_state_out = 5;
*/
com.google.assistant.embedded.v1alpha2.DialogStateOutOrBuilder getDialogStateOutOrBuilder();
/**
*
* *Output-only* Debugging info for developer. Only returned if request set
* `return_debug_info` to true.
*
*
* .google.assistant.embedded.v1alpha2.DebugInfo debug_info = 8;
* @return Whether the debugInfo field is set.
*/
boolean hasDebugInfo();
/**
*
* *Output-only* Debugging info for developer. Only returned if request set
* `return_debug_info` to true.
*
*
* .google.assistant.embedded.v1alpha2.DebugInfo debug_info = 8;
* @return The debugInfo.
*/
com.google.assistant.embedded.v1alpha2.DebugInfo getDebugInfo();
/**
*
* *Output-only* Debugging info for developer. Only returned if request set
* `return_debug_info` to true.
*
*
* .google.assistant.embedded.v1alpha2.DebugInfo debug_info = 8;
*/
com.google.assistant.embedded.v1alpha2.DebugInfoOrBuilder getDebugInfoOrBuilder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy