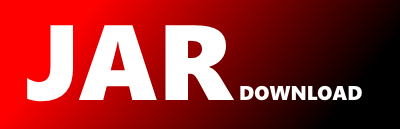
net.zeroinstall.model.impl.RestrictionBaseImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zeroinstall-model Show documentation
Show all versions of zeroinstall-model Show documentation
The Zero Install XSD model transformed by Apache XMLBeans to Java source code.
The newest version!
/*
* XML Type: restriction-base
* Namespace: http://zero-install.sourceforge.net/2004/injector/interface
* Java type: net.zeroinstall.model.RestrictionBase
*
* Automatically generated - do not modify.
*/
package net.zeroinstall.model.impl;
/**
* An XML restriction-base(@http://zero-install.sourceforge.net/2004/injector/interface).
*
* This is a complex type.
*/
public class RestrictionBaseImpl extends net.zeroinstall.model.impl.FeedElementImpl implements net.zeroinstall.model.RestrictionBase
{
private static final long serialVersionUID = 1L;
public RestrictionBaseImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName INTERFACE$0 =
new javax.xml.namespace.QName("", "interface");
private static final javax.xml.namespace.QName OS$2 =
new javax.xml.namespace.QName("", "os");
private static final javax.xml.namespace.QName DISTRIBUTION$4 =
new javax.xml.namespace.QName("", "distribution");
private static final javax.xml.namespace.QName VERSION$6 =
new javax.xml.namespace.QName("", "version");
/**
* Gets the "interface" attribute
*/
public java.lang.String getInterface()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(INTERFACE$0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "interface" attribute
*/
public org.apache.xmlbeans.XmlString xgetInterface()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_attribute_user(INTERFACE$0);
return target;
}
}
/**
* Sets the "interface" attribute
*/
public void setInterface(java.lang.String xinterface)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(INTERFACE$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(INTERFACE$0);
}
target.setStringValue(xinterface);
}
}
/**
* Sets (as xml) the "interface" attribute
*/
public void xsetInterface(org.apache.xmlbeans.XmlString xinterface)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_attribute_user(INTERFACE$0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlString)get_store().add_attribute_user(INTERFACE$0);
}
target.set(xinterface);
}
}
/**
* Gets the "os" attribute
*/
public net.zeroinstall.model.Os.Enum getOs()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(OS$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_default_attribute_value(OS$2);
}
if (target == null)
{
return null;
}
return (net.zeroinstall.model.Os.Enum)target.getEnumValue();
}
}
/**
* Gets (as xml) the "os" attribute
*/
public net.zeroinstall.model.Os xgetOs()
{
synchronized (monitor())
{
check_orphaned();
net.zeroinstall.model.Os target = null;
target = (net.zeroinstall.model.Os)get_store().find_attribute_user(OS$2);
if (target == null)
{
target = (net.zeroinstall.model.Os)get_default_attribute_value(OS$2);
}
return target;
}
}
/**
* True if has "os" attribute
*/
public boolean isSetOs()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(OS$2) != null;
}
}
/**
* Sets the "os" attribute
*/
public void setOs(net.zeroinstall.model.Os.Enum os)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(OS$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(OS$2);
}
target.setEnumValue(os);
}
}
/**
* Sets (as xml) the "os" attribute
*/
public void xsetOs(net.zeroinstall.model.Os os)
{
synchronized (monitor())
{
check_orphaned();
net.zeroinstall.model.Os target = null;
target = (net.zeroinstall.model.Os)get_store().find_attribute_user(OS$2);
if (target == null)
{
target = (net.zeroinstall.model.Os)get_store().add_attribute_user(OS$2);
}
target.set(os);
}
}
/**
* Unsets the "os" attribute
*/
public void unsetOs()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(OS$2);
}
}
/**
* Gets the "distribution" attribute
*/
public java.lang.String getDistribution()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(DISTRIBUTION$4);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "distribution" attribute
*/
public org.apache.xmlbeans.XmlString xgetDistribution()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_attribute_user(DISTRIBUTION$4);
return target;
}
}
/**
* True if has "distribution" attribute
*/
public boolean isSetDistribution()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(DISTRIBUTION$4) != null;
}
}
/**
* Sets the "distribution" attribute
*/
public void setDistribution(java.lang.String distribution)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(DISTRIBUTION$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(DISTRIBUTION$4);
}
target.setStringValue(distribution);
}
}
/**
* Sets (as xml) the "distribution" attribute
*/
public void xsetDistribution(org.apache.xmlbeans.XmlString distribution)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_attribute_user(DISTRIBUTION$4);
if (target == null)
{
target = (org.apache.xmlbeans.XmlString)get_store().add_attribute_user(DISTRIBUTION$4);
}
target.set(distribution);
}
}
/**
* Unsets the "distribution" attribute
*/
public void unsetDistribution()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(DISTRIBUTION$4);
}
}
/**
* Gets the "version" attribute
*/
public java.lang.String getVersion()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(VERSION$6);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "version" attribute
*/
public org.apache.xmlbeans.XmlString xgetVersion()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_attribute_user(VERSION$6);
return target;
}
}
/**
* True if has "version" attribute
*/
public boolean isSetVersion()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(VERSION$6) != null;
}
}
/**
* Sets the "version" attribute
*/
public void setVersion(java.lang.String version)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(VERSION$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(VERSION$6);
}
target.setStringValue(version);
}
}
/**
* Sets (as xml) the "version" attribute
*/
public void xsetVersion(org.apache.xmlbeans.XmlString version)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_attribute_user(VERSION$6);
if (target == null)
{
target = (org.apache.xmlbeans.XmlString)get_store().add_attribute_user(VERSION$6);
}
target.set(version);
}
}
/**
* Unsets the "version" attribute
*/
public void unsetVersion()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(VERSION$6);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy