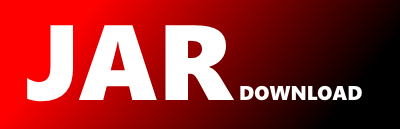
org.hyperledger.aries.BaseClient Maven / Gradle / Ivy
/*
* Copyright (c) 2020-2021 - for information on the respective copyright owner
* see the NOTICE file and/or the repository at
* https://github.com/hyperledger-labs/acapy-java-client
*
* SPDX-License-Identifier: Apache-2.0
*/
package org.hyperledger.aries;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.JsonParser;
import com.google.gson.reflect.TypeToken;
import lombok.extern.slf4j.Slf4j;
import okhttp3.*;
import org.apache.commons.lang3.StringUtils;
import org.hyperledger.acy_py.generated.model.DID;
import org.hyperledger.acy_py.generated.model.V20CredExRecordDetail;
import org.hyperledger.acy_py.generated.model.V20PresExRecord;
import org.hyperledger.aries.api.connection.ConnectionRecord;
import org.hyperledger.aries.api.credentials.Credential;
import org.hyperledger.aries.api.endorser.EndorseTransactionRecord;
import org.hyperledger.aries.api.exception.AriesException;
import org.hyperledger.aries.api.issue_credential_v1.V1CredentialExchange;
import org.hyperledger.aries.api.jsonld.ErrorResponse;
import org.hyperledger.aries.api.multitenancy.WalletRecord;
import org.hyperledger.aries.api.present_proof.PresentationExchangeRecord;
import org.hyperledger.aries.api.present_proof.PresentationRequestCredentials;
import org.hyperledger.aries.config.GsonConfig;
import javax.annotation.Nullable;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.concurrent.TimeUnit;
@Slf4j
abstract class BaseClient {
static final MediaType JSON_TYPE = MediaType.get("application/json; charset=utf-8");
static final Type CONNECTION_TYPE = new TypeToken>(){}.getType();
static final Type CREDENTIAL_TYPE = new TypeToken>(){}.getType();
static final Type ISSUE_CREDENTIAL_TYPE = new TypeToken>(){}.getType();
static final Type ISSUE_CREDENTIAL_V2_TYPE = new TypeToken>(){}.getType();
static final Type PRESENTATION_REQUEST_CREDENTIALS = new TypeToken>(){}.getType();
static final Type PROOF_TYPE = new TypeToken>(){}.getType();
static final Type PROOF_TYPE_V2 = new TypeToken>(){}.getType();
static final Type TRX_RECORD_TYPE = new TypeToken>(){}.getType();
static final Type WALLET_DID_TYPE = new TypeToken>(){}.getType();
static final Type WALLET_RECORD_TYPE = new TypeToken>(){}.getType();
static final Type MAP_TYPE = new TypeToken
© 2015 - 2025 Weber Informatics LLC | Privacy Policy