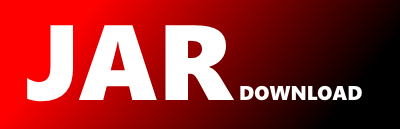
org.hyperledger.aries.api.resolver.DIDDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aries-client-python Show documentation
Show all versions of aries-client-python Show documentation
Aries Cloud Agent - Python: Java client implementation
/*
* Copyright (c) 2020-2021 - for information on the respective copyright owner
* see the NOTICE file and/or the repository at
* https://github.com/hyperledger-labs/acapy-java-client
*
* SPDX-License-Identifier: Apache-2.0
*/
package org.hyperledger.aries.api.resolver;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.google.gson.annotations.SerializedName;
import lombok.*;
import org.apache.commons.lang3.StringUtils;
import java.util.List;
import java.util.Optional;
/**
* W3C Did Document
* https://www.w3.org/TR/did-core/#core-properties
*/
@JsonPropertyOrder({"context", "id"})
@Data @NoArgsConstructor @AllArgsConstructor @Builder
public class DIDDocument {
public static final String ENDPOINT_TYPE_DID_COMM = "did-communication";
public static final String ENDPOINT_TYPE_PROFILE = "profile";
@JsonProperty("@context")
@SerializedName("@context")
private List context = List.of("https://www.w3.org/ns/did/v1");
private String id;
@SerializedName("alsoKnownAs")
private List alsoKnownAs;
private String controller;
@SerializedName("verificationMethod")
private List verificationMethod;
// structures below have the following content:
// String did = indexAt[0], VerificationMethod method = indexAt[1]
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy