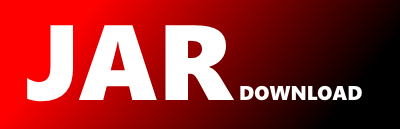
org.hyperledger.aries.api.jsonld.VerifiableCredential Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aries-client-python Show documentation
Show all versions of aries-client-python Show documentation
Aries Cloud Agent - Python: Java client implementation
/*
* Copyright (c) 2020-2021 - for information on the respective copyright owner
* see the NOTICE file and/or the repository at
* https://github.com/hyperledger-labs/acapy-java-client
*
* SPDX-License-Identifier: Apache-2.0
*/
package org.hyperledger.aries.api.jsonld;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.google.gson.annotations.SerializedName;
import lombok.*;
import lombok.experimental.SuperBuilder;
import org.hyperledger.aries.config.CredDefId;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.List;
/**
* @see VerifiableCredential
*/
@Data @SuperBuilder @NoArgsConstructor
@JsonPropertyOrder({ "@context", "type" })
@JsonInclude(Include.NON_NULL)
public class VerifiableCredential {
@Builder.Default
@NonNull @Nonnull
@SerializedName("@context")
@JsonProperty("@context")
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy