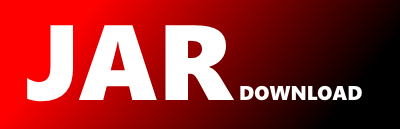
org.hyperledger.aries.api.server.AdminConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aries-client-python Show documentation
Show all versions of aries-client-python Show documentation
Aries Cloud Agent - Python: Java client implementation
/*
* Copyright (c) 2020-2021 - for information on the respective copyright owner
* see the NOTICE file and/or the repository at
* https://github.com/hyperledger-labs/acapy-java-client
*
* SPDX-License-Identifier: Apache-2.0
*/
package org.hyperledger.aries.api.server;
import com.google.gson.Gson;
import com.google.gson.JsonElement;
import com.google.gson.reflect.TypeToken;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
import org.hyperledger.aries.config.GsonConfig;
import java.lang.reflect.Type;
import java.util.Collection;
import java.util.Map;
import java.util.Optional;
@Slf4j
@Data @NoArgsConstructor
public class AdminConfig {
private static final Gson gson = GsonConfig.defaultConfig();
public static final Type COLLECTION_TYPE = new TypeToken>(){}.getType();
private Map config;
/**
* Get a config value
* @param key the config key e.g. debug.auto_accept_invites
* @param type the desired return type
* @param – the type of the desired result object
* @return the value or an empty optional if the key was not found or conversion failed
*/
public Optional getAs(@NonNull String key, @NonNull Type type) {
JsonElement value = config.get(key);
if (value != null) {
try {
return Optional.ofNullable(gson.fromJson(value, type));
} catch (Exception e) {
log.error("Could not convert {} to {}", key, type, e);
}
}
return Optional.empty();
}
/**
* Get a config value
* @param key the config key e.g. debug.auto_accept_invites
* @param type the desired return type
* @param – the type of the desired result object
* @return the value or null if the key was not found or conversion failed
*/
public T getUnwrapped(@NonNull String key, @NonNull Type type) {
JsonElement value = config.get(key);
if (value != null) {
try {
return gson.fromJson(value, type);
} catch (Exception e) {
log.error("Could not convert {} to {}", key, type, e);
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy