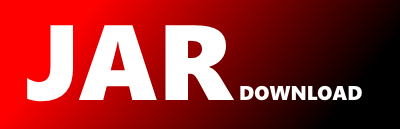
org.hyperledger.aries.webhook.EventParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aries-client-python Show documentation
Show all versions of aries-client-python Show documentation
Aries Cloud Agent - Python: Java client implementation
/*
* Copyright (c) 2020-2021 - for information on the respective copyright owner
* see the NOTICE file and/or the repository at
* https://github.com/hyperledger-labs/acapy-java-client
*
* SPDX-License-Identifier: Apache-2.0
*/
package org.hyperledger.aries.webhook;
import com.google.gson.*;
import com.google.gson.reflect.TypeToken;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.hyperledger.aries.api.present_proof.PresentationExchangeRecord;
import org.hyperledger.aries.api.present_proof.PresentationExchangeRecord.Identifier;
import org.hyperledger.aries.config.GsonConfig;
import org.hyperledger.aries.pojo.PojoProcessor;
import java.lang.reflect.Field;
import java.lang.reflect.Type;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.util.*;
import java.util.Map.Entry;
@Slf4j
public class EventParser {
private static final Type IDENTIFIER_TYPE = new TypeToken>(){}.getType();
private final Gson gson = GsonConfig.defaultConfig();
private final Gson pretty = GsonConfig.prettyPrinter();
public String prettyJson(@NonNull String json) {
JsonElement el = JsonParser.parseString(json);
return pretty.toJson(el);
}
public Optional parseValueSave(@NonNull String json, @NonNull Class valueType) {
Optional t = Optional.empty();
try {
t = Optional.ofNullable(gson.fromJson(json, valueType));
} catch (JsonSyntaxException e) {
log.error("Could not format json body", e);
}
return t;
}
public Optional parsePresentProof(String json) {
Optional presentation = parseValueSave(json, PresentationExchangeRecord.class);
if (presentation.isPresent()) {
JsonElement je = presentation.get()
.getPresentation()
.getAsJsonObject().get("identifiers")
;
List identifiers = gson.fromJson(je, IDENTIFIER_TYPE);
presentation.get().setIdentifiers(identifiers);
}
return presentation;
}
/**
* Converts the present_proof.presentation into an instance of the provided class type:
* @param The class type
* @param json present_proof.presentation
* @param type POJO instance
* @return Instantiated type with all matching properties set
*/
public static T from(@NonNull String json, @NonNull Class type) {
T result = PojoProcessor.getInstance(type);
final Set> revealedAttrs = getRevealedAttributes(json);
final Set> revealedAttrGroups = getRevealedAttrGroups(
json, PojoProcessor.getAttributeGroupName(type));
List fields = PojoProcessor.fields(type);
AccessController.doPrivileged((PrivilegedAction) () -> {
for(Field field: fields) {
String fieldName = PojoProcessor.fieldName(field);
String fieldValue = getValueFor(fieldName, revealedAttrs.isEmpty() ? revealedAttrGroups : revealedAttrs);
try {
field.setAccessible(true);
field.set(result, fieldValue);
} catch (IllegalAccessException | IllegalArgumentException e) {
log.error("Could not set value of field: {} to: {}", fieldName, fieldValue, e);
}
}
return null; // nothing to return
});
return result;
}
/**
* Finds the attribute names in the present_proof.presentation and extracts their corresponding values.
* @param json present_proof.presentation
* @param names Set of attribute names
* @return Map containing the attribute names and their corresponding values
*/
public static Map from(@NonNull String json, @NonNull Set names) {
Map result = new HashMap<>();
final Set> revealedAttrs = getRevealedAttributes(json);
final Set> revealedAttrGroups = getRevealedAttrGroups(json, null);
names.forEach(name -> {
String value = getValueFor(name, revealedAttrs.isEmpty() ? revealedAttrGroups : revealedAttrs);
if (StringUtils.isNotEmpty(value)) {
result.put(name, value);
}
});
return result;
}
private static String getValueFor(String name, Set> revealedAttrs) {
String result = null;
for (Entry e : revealedAttrs) {
String k = e.getKey();
if (k.equals(name) || k.contains(name + "_uuid") && e.getValue() != null) {
final JsonElement raw = e.getValue().getAsJsonObject().get("raw");
if (raw != null) {
result = raw.getAsString();
}
break;
}
}
return result;
}
private static Set> getRevealedAttributes(String json) {
return JsonParser
.parseString(json)
.getAsJsonObject().get("requested_proof")
.getAsJsonObject().get("revealed_attrs").getAsJsonObject()
.entrySet()
;
}
private static Set> getRevealedAttrGroups(@NonNull String json, String groupName) {
Set> result = new LinkedHashSet<>();
final JsonElement attr = JsonParser
.parseString(json)
.getAsJsonObject().get("requested_proof")
.getAsJsonObject().get("revealed_attr_groups")
;
if (attr == null) { // not an attr group
return result;
}
JsonObject attrGroup = attr.getAsJsonObject();
final Set children = attrGroup.keySet();
if (StringUtils.isNotEmpty(groupName)) {
extract(result, attrGroup, groupName);
} else { // brute force
children.forEach(c -> extract(result, attrGroup, c));
}
return result;
}
private static void extract(Set> result, JsonObject attrGroup, String groupName) {
final Set> attrs = attrGroup
.get(groupName).getAsJsonObject().get("values").getAsJsonObject().entrySet();
attrs.forEach(a -> {
if(!result.contains(a)) {
result.add(a);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy