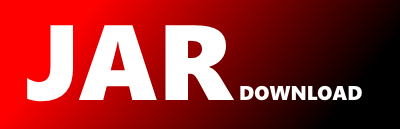
nl.bimbase.bimworks.client.UploadApi Maven / Gradle / Ivy
package nl.bimbase.bimworks.client;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.UUID;
import java.util.concurrent.TimeUnit;
import com.fasterxml.jackson.databind.node.ObjectNode;
import nl.sascom.backplanepublic.common.ClientTask;
import nl.sascom.backplanepublic.common.CommonErrorCode;
import nl.sascom.backplanepublic.common.ExecuteException;
import nl.sascom.backplanepublic.common.Request;
import nl.sascom.backplanepublic.common.Response;
import nl.sascom.backplanepublic.common.StreamAlreadyRegisteredException;
import nl.sascom.backplanepublic.common.utils.HashingUtils;
public class UploadApi {
private final BimWorksClient bimWorksClient;
public UploadApi(BimWorksClient bimWorksClient) {
this.bimWorksClient = bimWorksClient;
}
public UploadModelResponse uploadModel(UUID parentNodeUuid, String filename, long filesize, InputStream inputStream) throws IOException, InterruptedException, BimWorksException, StreamAlreadyRegisteredException, NodeAlreadyExistsException {
return uploadModel(parentNodeUuid, filename, filesize, inputStream, null);
}
public UploadModelResponse uploadModel(UUID parentNodeUuid, String filename, long filesize, InputStream inputStream, UploadOption uploadOption) throws IOException, InterruptedException, BimWorksException, StreamAlreadyRegisteredException, NodeAlreadyExistsException {
Request request = bimWorksClient.createRequest();
request.setTaskName("UploadModelToTree");
request.put("filename", filename);
request.put("filesize", filesize);
String contentType = "application/ifc";
request.put("contentType", contentType);
request.put("node_uuid", parentNodeUuid.toString());
ObjectNode options = Response.createObject();
if (uploadOption != null) {
options.put("force", uploadOption.name());
}
request.set("options", options);
ObjectNode hashNode = Response.createObject();
request.set("hash", hashNode);
BufferedInputStream bufferedInputStream = new BufferedInputStream(inputStream);
bufferedInputStream.mark(32 * 1024);
hashNode.put("partial_sha256", HashingUtils.extractPartialSha256(bufferedInputStream));
bufferedInputStream.reset();
request.setTimeOut(4, TimeUnit.HOURS);
String streamId = bimWorksClient.registerStream(filename, filesize, contentType, bufferedInputStream);
request.attachStream(streamId);
ClientTask task = bimWorksClient.createAsyncTask(request);
try {
task.exec();
Response response = task.await(4, TimeUnit.HOURS);
return new UploadModelResponse((ObjectNode) response.getObjectOutput());
} catch (ExecuteException e) {
if (e.getErrorCode().name().equals("DefaultErrorCode") && e.getErrorCode().getCode() == 29) {
throw new NodeAlreadyExistsException(e.getUserSafeMessage());
}
throw new BimWorksException(e);
}
}
public UploadModelResponse uploadModel(UUID parentNodeUuid, Path path) throws IOException, InterruptedException, BimWorksException, StreamAlreadyRegisteredException, NodeAlreadyExistsException {
return uploadModel(parentNodeUuid, path, null);
}
public UploadModelResponse uploadModel(UUID parentNodeUuid, Path path, UploadOption uploadOption) throws IOException, InterruptedException, BimWorksException, StreamAlreadyRegisteredException, NodeAlreadyExistsException {
Request request = bimWorksClient.createRequest();
request.setTaskName("UploadModelToTree");
request.put("filename", path.getFileName().toString());
request.put("filesize", Files.size(path));
request.put("contentType", "application/ifc");
request.put("node_uuid", parentNodeUuid.toString());
ObjectNode options = Response.createObject();
if (uploadOption != null) {
options.put("force", uploadOption.name());
}
request.set("options", options);
ObjectNode hashNode = Response.createObject();
request.set("hash", hashNode);
try (InputStream fis = Files.newInputStream(path)) {
hashNode.put("partial_sha256", HashingUtils.extractPartialSha256(fis));
}
request.setTimeOut(4, TimeUnit.HOURS);
String streamId = bimWorksClient.registerStream(path);
request.attachStream(streamId);
ClientTask task = bimWorksClient.createAsyncTask(request);
try {
task.exec();
Response response = task.await(4, TimeUnit.HOURS);
return new UploadModelResponse((ObjectNode) response.getObjectOutput());
} catch (ExecuteException e) {
if (e.getErrorCode().name().equals("DefaultErrorCode") && e.getErrorCode().getCode() == 29) {
throw new NodeAlreadyExistsException(e.getUserSafeMessage());
}
throw new BimWorksException(e);
}
}
public UploadModelResponse uploadModel(UUID parentNodeUuid, String filename, String contentType, String url, long filesize) throws IOException, InterruptedException, BimWorksException, StreamAlreadyRegisteredException, NodeAlreadyExistsException {
Request request = bimWorksClient.createRequest();
request.setTaskName("UploadModelToTree");
request.put("filename", filename);
request.put("filesize", filesize);
request.put("contentType", "application/ifc");
request.put("node_uuid", parentNodeUuid.toString());
request.setTimeOut(4, TimeUnit.HOURS);
String streamId = bimWorksClient.registerStream(filename, contentType, url, filesize);
request.attachStream(streamId);
ClientTask task = bimWorksClient.createAsyncTask(request);
try {
task.exec();
Response response = task.await(4, TimeUnit.HOURS);
if (response.getObjectOutput() == null) {
throw new ExecuteException(CommonErrorCode.NO_OUTPUT_IN_RESPONSE);
}
return new UploadModelResponse(response.getObjectOutput());
} catch (ExecuteException e) {
if (e.getErrorCode().name().equals("DefaultErrorCode") && e.getErrorCode().getCode() == 29) {
throw new NodeAlreadyExistsException(e.getUserSafeMessage());
}
throw new BimWorksException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy