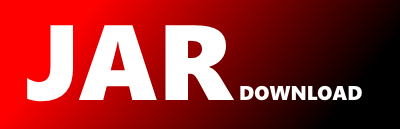
nl.clockwork.ebms.Eithers Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ebms-core Show documentation
Show all versions of ebms-core Show documentation
This library implements the ebXML Message Specification version 2.0, which defines a communications-protocol neutral method for exchanging electronic business messages through the exchange of XML based messages.
/**
* Copyright 2011 Clockwork
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package nl.clockwork.ebms;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Spliterator;
import java.util.Spliterators.AbstractSpliterator;
import java.util.function.BiFunction;
import java.util.function.BinaryOperator;
import java.util.function.Consumer;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import io.vavr.collection.Seq;
import io.vavr.control.Either;
import lombok.val;
public class Eithers
{
private static class EitherSpliterator extends AbstractSpliterator>
{
private Spliterator> spliterator;
private Predicate> predicate;
private boolean stopCondition = false;
public EitherSpliterator(Spliterator> spliterator, Predicate> predicate)
{
super(spliterator.estimateSize(),0);
this.spliterator = spliterator;
this.predicate = predicate;
}
@Override
public synchronized boolean tryAdvance(Consumer super Either> consumer)
{
boolean hadNext = spliterator.tryAdvance(either ->
{
if (!predicate.test(either))
stopCondition = true;
consumer.accept(either);
});
return hadNext && !stopCondition;
}
}
public static Either> sequenceRight(Stream> stream)
{
val identity = Either.>right(new ArrayList());
BiFunction>,Either,Either>> accumulator = (e,item) ->
{
if (e.isLeft())
return e;
if (item.isLeft())
return Either.>left(item.getLeft());
val list = e.get();
list.add(item.get());
return Either.>right(list);
};
BinaryOperator>> combiner = (e1,e2) ->
{
if (e1.isLeft())
return e1;
if (e2.isLeft())
return e2;
val list = Stream.concat(e1.get().stream(),e2.get().stream()).collect(Collectors.toList());
return Either.>right(list);
};
//return stream.reduce(identity,accumulator,combiner);
val spliterator = new EitherSpliterator(stream.spliterator(),e -> e.isRight());
return StreamSupport.stream(spliterator,false).reduce(identity,accumulator,combiner);
}
public static void main(String[] args) throws Exception
{
{
List> input = Arrays.asList(
Either.right(1),
Either.right(2));
//Either> result = reduce(r.stream());
val result = sequenceRight(input.stream().peek(i -> System.out.println(i)));
System.out.println(result.getOrElseThrow(e -> e));
}
System.out.println();
{
List> input = Arrays.asList(
Either.right(1),
Either.right(2));
val result = Either.sequenceRight(input);
System.out.println(result.getOrElseThrow(e -> e).asJava());
}
System.out.println();
try
{
List> input = Arrays.asList(
Either.right(1),
Either.left(new Exception("An error occurred!")),
Either.right(2));
val result = sequenceRight(input.stream().peek(i -> System.out.println(i)));
System.out.println(result.getOrElseThrow(e -> e));
}
catch (Exception e)
{
e.printStackTrace();
}
System.out.println();
try
{
List> input = Arrays.asList(
Either.right(1),
Either.left(new Exception("An error occurred!")),
Either.right(2));
Either> sequenceRight = Either.sequenceRight(input);
System.out.println(sequenceRight.getOrElseThrow(e -> e));
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy