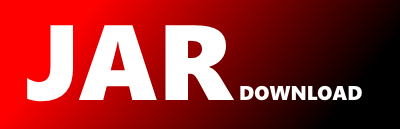
nl.cloudfarming.client.geoviewer.layers.LayerListTopComponent Maven / Gradle / Ivy
The newest version!
/**
* Copyright (C) 2010 Cloudfarming
*
* Licensed under the Eclipse Public License - v 1.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package nl.cloudfarming.client.geoviewer.layers;
import java.io.Serializable;
import java.util.Collection;
import java.util.logging.Logger;
import nl.cloudfarming.client.geoviewer.Layer;
import nl.cloudfarming.client.geoviewer.LayerListApi;
import nl.cloudfarming.client.geoviewer.MapController;
import org.openide.explorer.ExplorerManager;
import org.openide.explorer.ExplorerUtils;
import org.openide.explorer.view.BeanTreeView;
import org.openide.nodes.AbstractNode;
import org.openide.util.Lookup;
import org.openide.util.LookupEvent;
import org.openide.util.LookupListener;
import org.openide.util.NbBundle;
import org.openide.util.Utilities;
import org.openide.windows.TopComponent;
import org.openide.windows.WindowManager;
/**
* Top component which displays something.
*
* @author Timon Veenstra
*
*/
final class LayerListTopComponent extends TopComponent implements LayerListApi, ExplorerManager.Provider, LookupListener {
private static LayerListTopComponent instance;
private transient ExplorerManager explorerManager = new ExplorerManager();
private static final String PREFERRED_ID = "LayerListTopComponent";
private static final String ROOT_NODE_NAME = "root.node.name";
private static final Logger logger = Logger.getLogger(LayerListTopComponent.class.getName());
private LayerListTopComponent() {
initComponents();
setName(NbBundle.getMessage(LayerListTopComponent.class, "CTL_LayerListTopComponent"));
associateLookup(ExplorerUtils.createLookup(explorerManager, getActionMap()));
refresh();
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
beanTreeView = new BeanTreeView();
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(beanTreeView, javax.swing.GroupLayout.DEFAULT_SIZE, 424, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(beanTreeView, javax.swing.GroupLayout.DEFAULT_SIZE, 412, Short.MAX_VALUE)
);
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private org.openide.explorer.view.BeanTreeView beanTreeView;
// End of variables declaration//GEN-END:variables
/**
* Gets default instance. Do not use directly: reserved for *.settings files only,
* i.e. deserialization routines; otherwise you could get a non-deserialized instance.
* To obtain the singleton instance, use {@link findInstance}.
*/
public static synchronized LayerListTopComponent getDefault() {
if (instance == null) {
instance = new LayerListTopComponent();
}
return instance;
}
/**
* Obtain the LayerListTopComponent instance. Never call {@link #getDefault} directly!
*/
public static synchronized LayerListTopComponent findInstance() {
TopComponent win = WindowManager.getDefault().findTopComponent(PREFERRED_ID);
if (win == null) {
logger.warning("Cannot find " + PREFERRED_ID + " component. It will not be located properly in the window system.");
return getDefault();
}
if (win instanceof LayerListTopComponent) {
return (LayerListTopComponent) win;
}
logger.warning("There seem to be multiple components with the '" + PREFERRED_ID +
"' ID. That is a potential source of errors and unexpected behavior.");
return getDefault();
}
@Override
public int getPersistenceType() {
return TopComponent.PERSISTENCE_ALWAYS;
}
private Lookup.Result result = null;
@Override
@SuppressWarnings("unchecked")
public void componentOpened() {
Lookup.Template tpl = new Lookup.Template(Layer.class);
result = Utilities.actionsGlobalContext().lookup(tpl);
result.addLookupListener(this);
result.allItems();
}
@Override
public void componentClosed() {
result.removeLookupListener(this);
result = null;
}
/** replaces this in object stream */
@Override
public Object writeReplace() {
return new ResolvableHelper();
}
@Override
protected String preferredID() {
return PREFERRED_ID;
}
final static class ResolvableHelper implements Serializable {
private static final long serialVersionUID = 1L;
public Object readResolve() {
return LayerListTopComponent.getDefault();
}
}
/**
* Refreshes the layerlist, and therefore re-initialize the tree again.
*/
@Override
public void refresh() {
explorerManager.setRootContext(new AbstractNode(new MapChildren()));
explorerManager.getRootContext().setDisplayName(NbBundle.getMessage(this.getClass(), ROOT_NODE_NAME));
beanTreeView.expandAll();
}
@Override
public ExplorerManager getExplorerManager() {
return explorerManager;
}
@SuppressWarnings("unchecked")
@Override
public void resultChanged(LookupEvent lookupEvent) {
Lookup.Result r = (Lookup.Result) lookupEvent.getSource();
Collection c = r.allInstances();
if (c == null || c.size() <= 0) {
Lookup.Result maps = Lookup.getDefault().lookupResult(MapController.class);
for (MapController mapApi : maps.allInstances()) {
// mapApi.clearSelection();
}
} else {
MapController mapApi = Utilities.actionsGlobalContext().lookup(MapController.class);
assert mapApi != null;
// mapApi.setSelection(c);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy