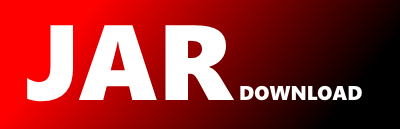
nl.cloudfarming.client.farm.model.Farm Maven / Gradle / Ivy
/**
* Copyright (C) 2011 Agrosense
*
* Licensed under the Eclipse Public License - v 1.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package nl.cloudfarming.client.farm.model;
import java.beans.PropertyChangeListener;
import java.beans.PropertyChangeSupport;
import java.io.Serializable;
/**
* Farm bean class representing a farm.
*
*
* @author Timon Veenstra
*/
public class Farm implements Serializable{
private String name;
public static final String PROP_NAME = "name";
private transient PropertyChangeSupport propertyChangeSupport = new PropertyChangeSupport(this);
PropertyChangeSupport getPropertyChangeSupport(){
if (propertyChangeSupport == null){
propertyChangeSupport = new PropertyChangeSupport(this);
}
return propertyChangeSupport;
}
/**
* Get the value of name
*
* @return the value of name
*/
public String getName() {
return name;
}
/**
* Set the value of name
*
* @param name new value of name
*/
public void setName(String name) {
String oldName = this.name;
this.name = name;
getPropertyChangeSupport().firePropertyChange(PROP_NAME, oldName, name);
}
/**
* Add PropertyChangeListener.
*
* @param listener
*/
public void addPropertyChangeListener(PropertyChangeListener listener) {
getPropertyChangeSupport().addPropertyChangeListener(listener);
}
/**
* Remove PropertyChangeListener.
*
* @param listener
*/
public void removePropertyChangeListener(PropertyChangeListener listener) {
getPropertyChangeSupport().removePropertyChangeListener(listener);
}
private String registrationNumber;
public static final String PROP_REGISTRATIONNUMBER = "registrationNumber";
/**
* Get the value of registrationNumber
*
* @return the value of registrationNumber
*/
public String getRegistrationNumber() {
return registrationNumber;
}
/**
* Set the value of registrationNumber
*
* @param registrationNumber new value of registrationNumber
*/
public void setRegistrationNumber(String registrationNumber) {
String oldRegistrationNumber = this.registrationNumber;
this.registrationNumber = registrationNumber;
getPropertyChangeSupport().firePropertyChange(PROP_REGISTRATIONNUMBER, oldRegistrationNumber, registrationNumber);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy