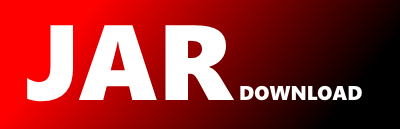
nl.cloudfarming.client.farm.model.CropField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of farm-model Show documentation
Show all versions of farm-model Show documentation
AgroSense Farm model - contains model/domain classes for farm modules
/**
* Copyright (C) 2010-2012 Agrosense [email protected]
*
* Licensed under the Eclipse Public License - v 1.0 (the "License"); you may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package nl.cloudfarming.client.farm.model;
import com.vividsolutions.jts.geom.Envelope;
import com.vividsolutions.jts.geom.Geometry;
import com.vividsolutions.jts.geom.Point;
import java.awt.Color;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.io.IOException;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import javax.swing.Action;
import nl.cloudfarming.client.crop.model.CropInstance;
import nl.cloudfarming.client.field.model.Field;
import nl.cloudfarming.client.geoviewer.DynamicGeometrical;
import nl.cloudfarming.client.model.AbstractEntity;
import nl.cloudfarming.client.model.AgroURI;
import nl.cloudfarming.client.model.ItemIdType;
import org.openide.util.Lookup;
/**
* Model class for Crop fields
*
* A CropField is part of a field where a certain crop is grown. It is always
* part of a {@link CropProductionUnit} which contains the crop that is meant to
* grow on this part of the field.
*
* A CropField is temporal and is only valid between start and end date.
*
* @author Gerben Feenstra
*/
public class CropField extends AbstractEntity implements Serializable, DynamicGeometrical, Transferable{
private static final long serialVersionUID = 8763622679187376702L;
public static final ItemIdType ITEM_ID_TYPE = ItemIdType.CFD;
public static final String EXT = "cfd";
public static final String MIMETYPE = "application/x-agrosense-cropfield";
private Geometry geometry;
public static final String PROP_GEOMETRY = "geometry";
private String name;
public static final String PROP_NAME = "name";
private double areaInHectares;
public static final String PROP_AREA_IN_HECTARES = "areaInHectares";
private Date startDate;
public static final String PROP_START_DATE = "startDate";
private Date endDate;
public static final String PROP_END_DATE = "endDate";
private final AgroURI fieldURI;
public static final String PROP_CROP = "crop";
private AgroURI parentURI;
public static final String PROP_COLOR = "cropFieldColor";
public static final DataFlavor DATA_FLAVOR = new DataFlavor(CropField.class, "CropField");
private transient List mapActions = null;
private transient CropProductionUnit productionUnit;
public CropField(Field field) {
super(field.getURI(), ITEM_ID_TYPE);
assert field != null : "Cannot create a crop field based on a null field";
areaInHectares = field.getAreaInHectares();
endDate = field.getEndDate();
startDate = field.getStartDate();
geometry = field.getGeometry();
name = field.getName();
fieldURI = field.getURI();
}
public CropField(CropField cropField) {
super(cropField.getURI(), ITEM_ID_TYPE);
assert cropField != null:"Cannot create a crop field based on a null crop field";
areaInHectares = cropField.getAreaInHectares();
endDate = cropField.getEndDate();
startDate = cropField.getStartDate();
geometry = cropField.getGeometry();
name = cropField.getName();
fieldURI = cropField.getFieldURI();
parentURI = cropField.getParentURI();
}
@Deprecated
public void setProductionUnit(CropProductionUnit productionUnit) {
setParentURI(productionUnit.getURI());
}
@Deprecated
public CropProductionUnit getProductionUnit(){
return getParent();
}
public CropInstance getCropInstance() {
return getParent().getCropInstance();
}
@Override
public Geometry getBoundingBox() {
return (this.geometry != null) ? geometry.getEnvelope() : null;
}
/**
* Get the value of endDate
*
* @return the value of endDate
*/
public Date getEndDate() {
return endDate;
}
/**
* Set the value of endDate
*
* @param endDate new value of endDate
*/
public void setEndDate(Date endDate) {
Date oldEndDate = this.endDate;
this.endDate = endDate;
getPropertyChangeSupport().firePropertyChange(PROP_END_DATE, oldEndDate, endDate);
}
/**
* Get the value of startDate
*
* @return the value of startDate
*/
public Date getStartDate() {
return startDate;
}
/**
* Set the value of startDate
*
* @param startDate new value of startDate
*/
public void setStartDate(Date startDate) {
Date oldStartDate = this.startDate;
this.startDate = startDate;
getPropertyChangeSupport().firePropertyChange(PROP_START_DATE, oldStartDate, startDate);
}
/**
* Get the value of areaInHectares
*
* @return the value of areaInHectares
*/
public double getAreaInHectares() {
return areaInHectares;
}
/**
* Set the value of areaInHectares
*
* @param areaInHectares new value of areaInHectares
*/
public void setAreaInHectares(double areaInHectares) {
double oldAreaInHectares = this.areaInHectares;
this.areaInHectares = areaInHectares;
getPropertyChangeSupport().firePropertyChange(PROP_AREA_IN_HECTARES, oldAreaInHectares, areaInHectares);
}
/**
* Get the value of geometry
*
* @return the value of geometry
*/
@Override
public Geometry getGeometry() {
return geometry;
}
/**
* Set the value of geometry
*
* @param geometry new value of geometry
*/
@Override
public void setGeometry(Geometry geometry) {
Geometry oldGeometry = this.geometry;
this.geometry = geometry;
getPropertyChangeSupport().firePropertyChange(PROP_GEOMETRY, oldGeometry, geometry);
}
/**
* Get the value of name
*
* @return the value of name
*/
public String getName() {
return name;
}
/**
* Set the value of name
*
* @param name new value of name
*/
public void setName(String name) {
String oldName = this.name;
this.name = name;
getPropertyChangeSupport().firePropertyChange(PROP_NAME, oldName, name);
}
public AgroURI getParentURI() {
return parentURI;
}
public void setParentURI(AgroURI parentURI) {
this.parentURI = parentURI;
// force reload via entity manager and cache:
productionUnit = null;
}
public synchronized CropProductionUnit getParent() {
if (parentURI == null) {
return null;
}
if (productionUnit == null) {
CropProductionUnitManager manager = Lookup.getDefault().lookup(CropProductionUnitManager.class);
productionUnit = manager.find(parentURI);
}
return productionUnit;
}
@Override
public Point getCentroid() {
return geometry == null ? null : geometry.getCentroid();
}
@Override
public Geometry getRenderObject(Envelope envelope) {
return geometry;
}
@Override
public String getTooltipText() {
return getName();
}
@Override
public String toString() {
return this.name;
}
public AgroURI getFieldURI() {
return fieldURI;
}
@Override
public String getIconPath() {
return null;
}
@Override
public String getIconLabel() {
return null;
}
@Override
public Action[] getMapActions() {
return getActions().toArray(new Action[0]);
}
private List getActions() {
if (mapActions == null) {
mapActions = new ArrayList<>();
}
return mapActions;
}
@Override
public void addMapAction(Action action) {
getActions().add(action);
}
@Override
public DataFlavor[] getTransferDataFlavors() {
return new DataFlavor[]{DATA_FLAVOR};
}
@Override
public boolean isDataFlavorSupported(DataFlavor flavor) {
return flavor.equals(DATA_FLAVOR);
}
@Override
public Object getTransferData(DataFlavor flavor) throws UnsupportedFlavorException, IOException {
if (isDataFlavorSupported(flavor)) {
return this;
}
throw new UnsupportedFlavorException(flavor);
}
public Color getColor() {
return getParent().getColor();
}
public void setColorChanged(Color oldColor, Color newColor){
getPropertyChangeSupport().firePropertyChange(PROP_COLOR, oldColor, newColor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy