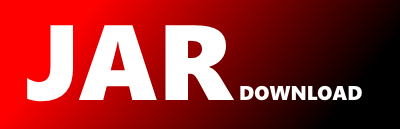
nl.cloudfarming.client.farm.model.FarmDetailsController Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of farm-model Show documentation
Show all versions of farm-model Show documentation
AgroSense Farm model - contains model/domain classes for farm modules
/**
* Copyright (C) 2010-2012 Agrosense [email protected]
*
* Licensed under the Eclipse Public License - v 1.0 (the "License"); you may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package nl.cloudfarming.client.farm.model;
import java.awt.event.ActionEvent;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.ImageIcon;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import nl.cloudfarming.client.model.AddressPanel;
import nl.cloudfarming.client.model.IssuingAgency;
import nl.cloudfarming.client.util.swing.ASBodyPanel;
import org.openide.util.ImageUtilities;
import org.openide.util.NbBundle;
/**
*
* @author Merijn Zengers
*/
@NbBundle.Messages({
"FarmDetailsController invalid name=Invalid name",
"FarmDetailsController invalid issuing agency=Invalid issuing agency",
"FarmDetailsController invalid registration number=Invalid registration number",
})
public abstract class FarmDetailsController {
protected final T farmDetailPanel;
private final ImageIcon TITLE_ICON = new ImageIcon(ImageUtilities.loadImage("nl/cloudfarming/client/farm/model/house32.png"));
private ASBodyPanel bodyPanel;
private static final Logger LOGGER = Logger.getLogger("nl.cloudfarming.client.farm.model");
/**
* Creates a FarmDetailsController
* @param farmDetailPanel
*/
public FarmDetailsController(T farmDetailPanel) {
this.farmDetailPanel = farmDetailPanel;
FarmDetailsChangedListener detailsDocumentListener = new FarmDetailsChangedListener();
farmDetailPanel.farmNameTextField.getDocument().addDocumentListener(new NameChangedListener());
farmDetailPanel.farmNameTextField.getDocument().addDocumentListener(detailsDocumentListener);
farmDetailPanel.farmRegistrationNumberTextField.getDocument().addDocumentListener(detailsDocumentListener);
//Register this as document listener on The addres panel
farmDetailPanel.addressPanel.addAddressFieldChangeListener(detailsDocumentListener);
}
/**
* Validate the form
* Sets the FormRows to valid invalid
* @return true is valid
*/
public boolean validate() {
String name = farmDetailPanel.getFarmName();
boolean valid = true;
if (name == null || name.isEmpty()) {
valid = false;
farmDetailPanel.getFarmNameRow().setInvalid(Bundle.FarmDetailsController_invalid_name());
} else {
farmDetailPanel.getFarmNameRow().setValid();
}
IssuingAgency issuingAgency = farmDetailPanel.getFarmIssuingAgency();
String registrationNumber = farmDetailPanel.getFarmRegistrationNumber();
if (issuingAgency == null) {
valid = false;
farmDetailPanel.getFarmIssuingAgencyRow().setInvalid(Bundle.FarmDetailsController_invalid_issuing_agency());
} else {
farmDetailPanel.getFarmIssuingAgencyRow().setValid();
}
if (registrationNumber == null || registrationNumber.isEmpty() && farmDetailPanel.isFarmRegistrationNumberFieldEditable()) {
valid = false;
farmDetailPanel.getRegistrationNumberRow().setInvalid(Bundle.FarmDetailsController_invalid_registration_number());
} else // check agency specific pattern:
if (issuingAgency != null && !issuingAgency.isValidEnterpriseId(registrationNumber) && farmDetailPanel.isFarmRegistrationNumberFieldEditable()) {
farmDetailPanel.getRegistrationNumberRow().setWarning(issuingAgency.getPatternMessage());
} else {
farmDetailPanel.getRegistrationNumberRow().setValid();
}
if (!farmDetailPanel.getAddressPanel().validatePanel()) {
valid = false;
}
return valid;
}
/**
* Get the {@link ASBodyPanel} containing the panel provided in the constructor
* @return
*/
public ASBodyPanel getPanel() {
if (bodyPanel == null) {
bodyPanel = new ASBodyPanel(farmDetailPanel);
bodyPanel.setIcon(TITLE_ICON);
}
return bodyPanel;
}
public T getFarmDetailPanel() {
return farmDetailPanel;
}
public abstract void farmDetailFieldsUpdated();
private class FarmDetailsChangedListener implements AddressPanel.AddressFieldChangeListener {
@Override
public void insertUpdate(DocumentEvent e) {
changed();
}
@Override
public void removeUpdate(DocumentEvent e) {
changed();
}
@Override
public void changedUpdate(DocumentEvent e) {
changed();
}
void changed() {
FarmDetailsController.this.farmDetailFieldsUpdated();
}
@Override
public void actionPerformed(ActionEvent ae) {
changed();
}
}
private class NameChangedListener implements DocumentListener {
@Override
public void insertUpdate(DocumentEvent e) {
updateTitle(e.getDocument());
}
@Override
public void removeUpdate(DocumentEvent e) {
updateTitle(e.getDocument());
}
@Override
public void changedUpdate(DocumentEvent e) {
updateTitle(e.getDocument());
}
void updateTitle(Document d) {
try {
bodyPanel.setTitle(d.getText(0, d.getLength()));
} catch (BadLocationException ex) {
LOGGER.log(Level.SEVERE, "Could not update the title due to an exception");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy