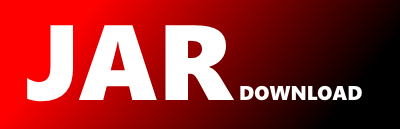
nl.cloudfarming.client.farm.model.FarmDetailsPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of farm-model Show documentation
Show all versions of farm-model Show documentation
AgroSense Farm model - contains model/domain classes for farm modules
/**
* Copyright (C) 2010-2012 Agrosense [email protected]
*
* Licensed under the Eclipse Public License - v 1.0 (the "License"); you may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package nl.cloudfarming.client.farm.model;
import java.awt.Dimension;
import javax.swing.JComponent;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
import net.miginfocom.swing.MigLayout;
import nl.cloudfarming.client.model.Address;
import nl.cloudfarming.client.model.AddressPanel;
import nl.cloudfarming.client.model.IssuingAgency;
import nl.cloudfarming.client.util.swing.FormRow;
import org.openide.util.NbBundle;
import org.openide.util.NbBundle.Messages;
/**
*
* @author Timon Veenstra & Gerben Feenstra
*/
@Messages({
"FarmDetailsPanel.icon.toolTipText=Farm Details",
"FarmDetailsPanel.icon.text=",
"FarmDetailsPanel.nameLabel.toolTipText=Company name",
"FarmDetailsPanel.nameLabel.text=Company name:",
"FarmDetailsPanel.issuingAgencyLabel.toolTipText=Issuing agency",
"FarmDetailsPanel.issuingAgencyLabel.text=Issuing agency:",
"FarmDetailsPanel.registrationNumberLabel.toolTipText=Registration number",
"FarmDetailsPanel.registrationNumberLabel.text=Registration number:"
})
public abstract class FarmDetailsPanel extends JPanel {
protected static final int FIELD_WIDTH = 350;
private static final String ICON_LABEL_TEXT = NbBundle.getMessage(FarmDetailsPanel.class, "FarmDetailsPanel.icon.text");
private static final String ICON_LABEL_TOOLTIP_TEXT = NbBundle.getMessage(FarmDetailsPanel.class, "FarmDetailsPanel.icon.toolTipText");
private static final String NAME_LABEL_TEXT = NbBundle.getMessage(FarmDetailsPanel.class, "FarmDetailsPanel.nameLabel.text");
private static final String NAME_LABEL_TOOLTIP_TEXT = NbBundle.getMessage(FarmDetailsPanel.class, "FarmDetailsPanel.nameLabel.toolTipText");
private static final String ISSUING_AGENCY_LABEL_TEXT = NbBundle.getMessage(FarmDetailsPanel.class, "FarmDetailsPanel.issuingAgencyLabel.text");
private static final String ISSUING_AGENCY_LABEL_TOOLTIP_TEXT = NbBundle.getMessage(FarmDetailsPanel.class, "FarmDetailsPanel.issuingAgencyLabel.toolTipText");
private static final String REGISTRATION_LABEL_TEXT = NbBundle.getMessage(FarmDetailsPanel.class, "FarmDetailsPanel.registrationNumberLabel.text");
private static final String REGISTRATION_LABEL_TOOLTIP_TEXT = NbBundle.getMessage(FarmDetailsPanel.class, "FarmDetailsPanel.registrationNumberLabel.toolTipText");
private Address address;
protected JTextField farmNameTextField = new JTextField();
// workaround for hard to read disabled comboboxes;
// let subclasses pass in the component, so we can use e.g. a non-editable textfield instead
// (making a combobox non-editable only disables entering text and still allows selecting another item)
protected JComponent farmIssuingAgencyComponent;
protected JTextField farmRegistrationNumberTextField = new JTextField();
protected AddressPanel addressPanel;
private FormRow farmNameRow;
private FormRow farmIssuingAgencyRow;
private FormRow registrationNumberRow;
/**
* Creates new form FarmDetailsPanel
*
* @param
*/
public FarmDetailsPanel(JComponent farmIssuingAgencyComponent, Address address) {
this.address = address;
this.farmIssuingAgencyComponent = farmIssuingAgencyComponent;
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
*/
private void initComponents() {
this.setLayout(new MigLayout("wrap"));
JLabel farmIconLabel = new JLabel();
farmIconLabel.setText(ICON_LABEL_TEXT);
farmIconLabel.setToolTipText(ICON_LABEL_TOOLTIP_TEXT);
farmNameTextField.setPreferredSize(new Dimension(FIELD_WIDTH, 0));
farmNameRow = new FormRow(NAME_LABEL_TEXT, NAME_LABEL_TOOLTIP_TEXT, farmNameTextField);
farmIssuingAgencyComponent.setPreferredSize(new Dimension(FIELD_WIDTH, 0));
farmIssuingAgencyRow = new FormRow(ISSUING_AGENCY_LABEL_TEXT, ISSUING_AGENCY_LABEL_TOOLTIP_TEXT, farmIssuingAgencyComponent);
farmRegistrationNumberTextField.setPreferredSize(new Dimension(FIELD_WIDTH, 0));
registrationNumberRow = new FormRow(REGISTRATION_LABEL_TEXT, REGISTRATION_LABEL_TOOLTIP_TEXT, farmRegistrationNumberTextField);
addressPanel = new AddressPanel(address);
this.add(farmNameRow);
this.add(farmIssuingAgencyRow);
this.add(registrationNumberRow);
this.add(addressPanel, "span");
}
/**
* Get the name of the farm
*
* @return
*/
public String getFarmName() {
return farmNameTextField.getText().trim();
}
/**
* Set the name of the farm
*
* @param name
*/
public void setFarmName(String name) {
farmNameTextField.setText(name);
}
/**
* Get the farm name text field
*/
JTextField getFarmNameTextField(){
return farmNameTextField;
}
public abstract IssuingAgency getFarmIssuingAgency();
public abstract void setFarmIssuingAgency(IssuingAgency issuingAgency);
/**
* Get the Registration number
* @return
*/
public String getFarmRegistrationNumber() {
return farmRegistrationNumberTextField.getText().trim();
}
/**
* Set the registration number
* @param registrationNumber S
*/
public void setFarmRegistrationNumber(String registrationNumber) {
farmRegistrationNumberTextField.setText(registrationNumber);
}
/**
* Check if the registration number field is editable
* @return
*/
public boolean isFarmRegistrationNumberFieldEditable(){
return farmRegistrationNumberTextField.isEditable();
}
/**
* Get the {@link AddressPanel} from this form
*
* @return
*/
public AddressPanel getAddressPanel() {
return addressPanel;
}
/**
* get the address from this panel
*/
public Address getAddress(){
return address;
}
public FormRow getFarmIssuingAgencyRow() {
return farmIssuingAgencyRow;
}
public FormRow getFarmNameRow() {
return farmNameRow;
}
public FormRow getRegistrationNumberRow() {
return registrationNumberRow;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy