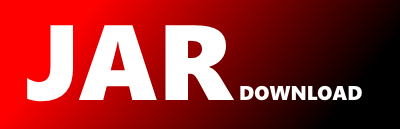
nl.cloudfarming.client.farm.model.AddressPanel Maven / Gradle / Ivy
Show all versions of farm-model Show documentation
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.farm.model;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.event.ActionListener;
import java.util.Arrays;
import javax.swing.JComboBox;
import javax.swing.JTextField;
import javax.swing.event.DocumentListener;
import net.miginfocom.swing.MigLayout;
import nl.cloudfarming.client.model.Address;
import nl.cloudfarming.client.model.Country;
import nl.cloudfarming.client.model.PostalCodeValidator;
import nl.cloudfarming.client.model.PostalCodeValidator.ValidationException;
import nl.cloudfarming.client.util.swing.FormRow;
import nl.cloudfarming.client.util.swing.beanbinding.IntegerConverter;
import nl.cloudfarming.client.util.swing.beanbinding.IntegerValidator;
import org.jdesktop.beansbinding.*;
import org.jdesktop.swingx.autocomplete.AutoCompleteDecorator;
import org.jdesktop.swingx.combobox.ListComboBoxModel;
import org.openide.util.NbBundle;
/**
* Panel for address data
*
* Panel contains the following components in layout
*
* House number label---house number field---addition label---addition
* field
Postal code label---postal code field
Street label---street
* field
City label---city field
country label---country combo
* box
*
*
*
* Use the constructor {@link AddressPanel#AddressPanel(nl.cloudfarming.client.model.Address, int, int)}
* to give the Labels on the left and the Fields on the right a width
* alternative you can use {@link AddressPanel#setLabelWidth(int)} and {@link AddressPanel#setFieldwidth(int)}
*
*
* @author Merijn Zengers
*/
@NbBundle.Messages({"AddressPanel house number invalid message=Should be a number",
"AddressPanel streetLabel text=Street:",
"AddressPanel houseNumberLabel text=House number:",
"AddressPanel cityLabel text=City:",
"AddressPanel postalCodeLabel text=Postal code:",
"AddressPanel countryLabel text=Country:",
"AddressPanel houseNumberAdditionLabel text=Addition:"})
public class AddressPanel extends javax.swing.JPanel {
private ELProperty streetNameProperty = ELProperty.create("${text_ON_ACTION_OR_FOCUS_LOST}");
private BeanProperty addressPropStreetName = BeanProperty.create(Address.PROP_STREET_NAME);
private Binding streetNameBinding;
private ELProperty houseNumberProperty = ELProperty.create("${text_ON_ACTION_OR_FOCUS_LOST}");
private BeanProperty addressPropHouseNumber = BeanProperty.create(Address.PROP_HOUSE_NUMBER);
private Binding houseNumberBinding;
private ELProperty postalCodeProperty = ELProperty.create("${text_ON_ACTION_OR_FOCUS_LOST}");
private BeanProperty addressPropPostalCode = BeanProperty.create(Address.PROP_POSTAL_CODE);
private Binding postalCodeBinding;
private ELProperty cityProperty = ELProperty.create("${text_ON_ACTION_OR_FOCUS_LOST}");
private BeanProperty addressPropCity = BeanProperty.create(Address.PROP_CITY);
private Binding cityBinding;
private BeanProperty countryProperty = BeanProperty.create("selectedItem");
private BeanProperty addressPropCountry = BeanProperty.create(Address.PROP_COUNTRY);
private Binding countryBinding;
private ELProperty additionProperty = ELProperty.create("${text_ON_ACTION_OR_FOCUS_LOST}");
private BeanProperty addressPropAddition = BeanProperty.create(Address.PROP_ADDITION);
private Binding additionBinding;
private Address address;
private ListComboBoxModel countryModel;
private FormRow houseNumberAditionRow;
private FormRow postalCodeRow;
private BindingGroup addressBindingGroup = new BindingGroup();
/**
* {@link JTextField} to enter the addition to the house number of this {@link Address}
* this panel is bound to
*/
private JTextField additionField;
/**
* {@link JTextField} which holds the city of this {@link Address} this
* panel is bound to
*/
private JTextField cityField;
/**
* {@link JComboBox} which contains all the {@link Country} 's of this {@link Address}
* this panel is bound to
*/
private JComboBox countryComboBox;
/**
* {@link JTextField} which holds the house number of this {@link Address}
* this panel is bound to
*/
private JTextField houseNumberField;
/**
* {@link JTextField} which holds the postal code for this {@link Address}
* this panel is bound to
*/
private JTextField postalCodeField;
/**
* {@link JTextField} which holds the street for this {@link Address} this
* panel is bound to
*/
private JTextField streetField;
private static final Color BACKGROUND_COLOR = Color.WHITE;
private static final int HOUSE_NUMBER_WIDTH = 120;
private static final int ADDITION_WIDTH = 40;
/**
* Creates new form AddressPanel Uses default label and field width
*
* @param address
*/
public AddressPanel(Address address) {
this.address = address;
initComponents();
bind();
AutoCompleteDecorator.decorate(this.countryComboBox);
}
/**
* bind form fields to Address properties
*/
private void bind() {
streetNameBinding = Bindings.createAutoBinding(AutoBinding.UpdateStrategy.READ_WRITE,
address, addressPropStreetName, streetField, streetNameProperty);
addressBindingGroup.addBinding(streetNameBinding);
houseNumberBinding = Bindings.createAutoBinding(AutoBinding.UpdateStrategy.READ_WRITE,
address, addressPropHouseNumber, houseNumberField, houseNumberProperty);
houseNumberBinding.setConverter(new IntegerConverter());
houseNumberBinding.setValidator(new IntegerValidator());
addressBindingGroup.addBinding(houseNumberBinding);
postalCodeBinding = Bindings.createAutoBinding(AutoBinding.UpdateStrategy.READ_WRITE,
address, addressPropPostalCode, postalCodeField, postalCodeProperty);
addressBindingGroup.addBinding(postalCodeBinding);
cityBinding = Bindings.createAutoBinding(AutoBinding.UpdateStrategy.READ_WRITE,
address, addressPropCity, cityField, cityProperty);
addressBindingGroup.addBinding(cityBinding);
countryBinding = Bindings.createAutoBinding(AutoBinding.UpdateStrategy.READ_WRITE,
address, addressPropCountry, countryComboBox, countryProperty);
addressBindingGroup.addBinding(countryBinding);
additionBinding = Bindings.createAutoBinding(AutoBinding.UpdateStrategy.READ_WRITE,
address, addressPropAddition, additionField, additionProperty);
addressBindingGroup.addBinding(additionBinding);
addressBindingGroup.bind();
}
/**
* Get the {@link JTextField} which contains the city
*
* @return
*/
public JTextField getCityField() {
return cityField;
}
/**
* Get the {@link JComboBox} which contains the {@link Country}
*
* @return
*/
public JComboBox getCountryComboBox() {
return countryComboBox;
}
/**
* Get the {@link JTextField} which contains the house number
*
* @return
*/
public JTextField getHouseNumberField() {
return houseNumberField;
}
/**
* Get the {@link JTextField} which contains the postal code
*
* @return
*/
public JTextField getPostalCodeField() {
return postalCodeField;
}
/**
* Get the {@link JTextField} which contains the street
*
* @return
*/
public JTextField getStreetField() {
return streetField;
}
/**
* Get the {@link JTextField} which contains the house number addition
*
* @return
*/
public JTextField getAdditionField() {
return additionField;
}
/**
* Add listener on AddresPanel fields
Adds listeners to fields:
* {@link ActionListener} on {@link AddressPanel#countryComboBox}
* {@link DocumentListener} on {@link AddressPanel#postalCodeField}
* {@link DocumentListener} on {@link AddressPanel#houseNumberField}
* {@link DocumentListener} on {@link AddressPanel#additionField}
* {@link DocumentListener} on {@link AddressPanel#streetField}
* {@link DocumentListener} on {@link AddressPanel#cityField}
*
* @param listener
*/
public void addAddressFieldChangeListener(AddressFieldChangeListener listener) {
countryComboBox.addActionListener(listener);
postalCodeField.getDocument().addDocumentListener(listener);
houseNumberField.getDocument().addDocumentListener(listener);
additionField.getDocument().addDocumentListener(listener);
streetField.getDocument().addDocumentListener(listener);
cityField.getDocument().addDocumentListener(listener);
}
/**
* removes listener on AddresPanel fields
removed listeners from
* fields:
* {@link ActionListener} on {@link AddressPanel#countryComboBox}
* {@link DocumentListener} on {@link AddressPanel#postalCodeField}
* {@link DocumentListener} on {@link AddressPanel#houseNumberField}
* {@link DocumentListener} on {@link AddressPanel#additionField}
* {@link DocumentListener} on {@link AddressPanel#streetField}
* {@link DocumentListener} on {@link AddressPanel#cityField}
*
* @param listener
*/
public void removeAddressFieldChangeListener(AddressFieldChangeListener listener) {
countryComboBox.removeActionListener(listener);
postalCodeField.getDocument().removeDocumentListener(listener);
houseNumberField.getDocument().removeDocumentListener(listener);
additionField.getDocument().removeDocumentListener(listener);
streetField.getDocument().removeDocumentListener(listener);
cityField.getDocument().removeDocumentListener(listener);
}
/**
* Validates this form
*
* Will check if the house number is a number if it is filled
Will
* check the postal code for the country that is selected
Only validates
* the postal code when it is filled Sets the warnings on the FormRow
* if validation fails
*
* @return true if valid
*/
public boolean validatePanel() {
boolean valid = true;
if (!getHouseNumberField().getText().isEmpty()) {
try {
Integer.parseInt(getHouseNumberField().getText());
houseNumberAditionRow.setValid();
} catch (NumberFormatException ex) {
houseNumberAditionRow.setInvalid(Bundle.AddressPanel_house_number_invalid_message());
valid = false;
}
} else {
houseNumberAditionRow.setValid();
}
PostalCodeValidator postalCodeValidator = new PostalCodeValidator();
if (!getPostalCodeField().getText().isEmpty()) {
try {
postalCodeValidator.validate((Country) getCountryComboBox().getSelectedItem(), getPostalCodeField().getText());
postalCodeRow.setValid();
} catch (ValidationException ex) {
postalCodeRow.setWarning(ex.getMessage());
}
} else {
postalCodeRow.setValid();
}
return valid;
}
/**
* This method is called from within the constructor to initialize the form.
*/
private void initComponents() {
this.setLayout(new MigLayout("wrap 1, insets 0 0 0 0"));
postalCodeField = new JTextField();
houseNumberField = new JTextField();
houseNumberField.setPreferredSize(new Dimension(HOUSE_NUMBER_WIDTH, 0));
additionField = new JTextField();
additionField.setPreferredSize(new Dimension(ADDITION_WIDTH, 0));
streetField = new JTextField();
cityField = new JTextField();
countryComboBox = new JComboBox();
setBackground(BACKGROUND_COLOR);
FormRow streetRow = new FormRow(Bundle.AddressPanel_streetLabel_text(), Bundle.AddressPanel_streetLabel_text(), streetField);
houseNumberAditionRow = new FormRow(Bundle.AddressPanel_houseNumberLabel_text(), Bundle.AddressPanel_houseNumberLabel_text(), houseNumberField, Bundle.AddressPanel_houseNumberAdditionLabel_text(), Bundle.AddressPanel_houseNumberAdditionLabel_text(), additionField);
postalCodeRow = new FormRow(Bundle.AddressPanel_postalCodeLabel_text(), Bundle.AddressPanel_postalCodeLabel_text(), postalCodeField);
FormRow cityRow = new FormRow(Bundle.AddressPanel_cityLabel_text(), Bundle.AddressPanel_cityLabel_text(), cityField);
FormRow countryRow = new FormRow(Bundle.AddressPanel_countryLabel_text(), Bundle.AddressPanel_countryLabel_text(), countryComboBox);
Country[] countries = Country.values();
Arrays.sort(countries, Country.getNameComparator());
countryModel = new ListComboBoxModel<>(Arrays.asList(countries));
countryComboBox.setModel(countryModel);
this.add(houseNumberAditionRow);
this.add(postalCodeRow);
this.add(streetRow);
this.add(cityRow);
this.add(countryRow);
}
/**
* Interface to use to register listeners on the components on this
* panel
The {@link DocumentListener} is used to register on the {@link JTextField}
* 's
The {@link ActionListener} is used to register on the {@link JComboBox}
*
* implement this interface and register using {@link AddressPanel#addAddressFieldChangeListener(nl.cloudfarming.client.model.AddressPanel.AddressFieldChangeListener)
* }
*/
public interface AddressFieldChangeListener extends DocumentListener, ActionListener {
}
}