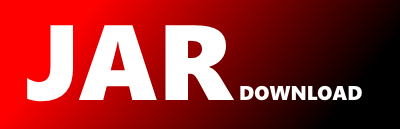
nl.cloudfarming.client.fleet.machine.MachineDataObject Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.fleet.machine;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.io.IOException;
import javax.swing.Icon;
import nl.cloudfarming.client.fleet.model.Machine;
import nl.cloudfarming.client.fleet.model.MachineManager;
import nl.cloudfarming.client.geoviewer.SimpleLayer;
import nl.cloudfarming.client.model.EntitySavable;
import org.netbeans.api.actions.Savable;
import org.openide.filesystems.FileObject;
import org.openide.loaders.DataObjectExistsException;
import org.openide.loaders.MultiDataObject;
import org.openide.loaders.MultiFileLoader;
import org.openide.util.ImageUtilities;
import org.openide.util.Lookup;
import org.openide.util.lookup.AbstractLookup;
import org.openide.util.lookup.InstanceContent;
import org.openide.util.lookup.ProxyLookup;
/**
*
* @author Merijn Zengers
*/
public class MachineDataObject extends MultiDataObject implements PropertyChangeListener {
private final InstanceContent ic = new InstanceContent();
private final Lookup lkp = new AbstractLookup(ic);
private final Machine machine;
private final MachineManager machineManager;
private final static String ICON = "nl/cloudfarming/client/fleet/machine/fleet.png";
public MachineDataObject(FileObject fo, MultiFileLoader loader, MachineManager machineManager) throws DataObjectExistsException {
super(fo, loader);
this.machineManager = machineManager;
this.machine = machineManager.load(fo);
ic.add(machine);
ic.add(machine.getGeometrical());
ic.add(new SimpleLayer(machine.getName()));
machine.addPropertyChangeListener(this);
}
@Override
public Lookup getLookup() {
return new ProxyLookup(super.getLookup(), lkp);
}
private static final Icon IMAGE_ICON = ImageUtilities.loadImageIcon(ICON, true);
@Override
public void propertyChange(PropertyChangeEvent pce) {
if (lkp.lookup(Savable.class) == null) {
this.ic.add(new MachineSavable());
}
}
private class MachineSavable extends EntitySavable {
public MachineSavable() {
super(machine, IMAGE_ICON);
register();
}
@Override
public void handleSave() throws IOException {
dataObject().machineManager.save(machine);
destroy();
}
/**
* Cancel the save operation Removes this Savable from the lookup and
* unregisters
*/
@Override
public void destroy() {
dataObject().ic.remove(MachineSavable.this);
unregister();
}
@Override
protected String findDisplayName() {
return machine.getName();
}
MachineDataObject dataObject() {
return MachineDataObject.this;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof MachineSavable) {
return dataObject() == ((MachineSavable) obj).dataObject();
}
return false;
}
@Override
public int hashCode() {
return dataObject().hashCode();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy