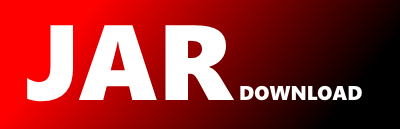
nl.cloudfarming.client.fleet.machine.MachineDataService Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.fleet.machine;
import java.util.*;
import nl.cloudfarming.client.fleet.model.MachinePropertyDefinition;
/**
* This class defines the functionality for returning a list of machine models.
* These models are added to the selection fields in the machine creation
* wizard.
*
* @author Wytse Visser
*/
public interface MachineDataService {
public List getModels();
public class Model {
private String type;
private String brand;
private String modelNumber;
private Map properties = new HashMap<>();
private List additionalSteps;
public Model(String type, String brand, String modelNumber) {
this.type = type;
this.brand = brand;
this.modelNumber = modelNumber;
additionalSteps = new ArrayList<>();
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public String getModelNumber() {
return modelNumber;
}
public void setModelNumber(String modelNumber) {
this.modelNumber = modelNumber;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final Model other = (Model) obj;
if (!Objects.equals(this.type, other.type)) {
return false;
}
if (!Objects.equals(this.brand, other.brand)) {
return false;
}
if (!Objects.equals(this.modelNumber, other.modelNumber)) {
return false;
}
return true;
}
@Override
public int hashCode() {
int hash = 3;
hash = 89 * hash + Objects.hashCode(this.type);
hash = 89 * hash + Objects.hashCode(this.brand);
hash = 89 * hash + Objects.hashCode(this.modelNumber);
return hash;
}
public void putProperty(String key, Object value) {
properties.put(key, value);
}
public Object getProperty(String key) {
return properties.get(key);
}
public void addAdditionalStep(StepInfo step) {
additionalSteps.add(step);
}
public List getAdditionalSteps() {
return additionalSteps;
}
}
public class StepInfo {
private String name;
private List properties = new ArrayList<>();
public StepInfo(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void addProperty(MachinePropertyDefinition property) {
properties.add(property);
}
public List getProperties() {
return properties;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy