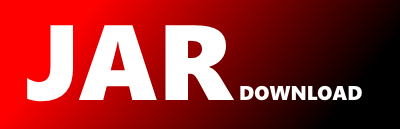
nl.cloudfarming.client.fleet.machine.windows.MachineOverviewPanel Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.fleet.machine.windows;
import java.awt.Dimension;
import java.util.Calendar;
import javax.swing.*;
import net.miginfocom.swing.MigLayout;
import nl.cloudfarming.client.util.swing.FormRow;
import org.jdesktop.swingx.JXDatePicker;
import org.openide.util.NbBundle;
/**
*
* @author Wytse Visser
* @author Merijn zengers
*/
@NbBundle.Messages({
"CTL_OverviewTopComponent=Overview",
"HINT_OverviewTopComponent=This is a machine overview",
"MachineOverviewPanel name label text=Name:",
"MachineOverviewPanel type label text=Type:",
"MachineOverviewPanel brand label text=Brand:",
"MachineOverviewPanel model number label text=Model number:",
"MachineOverviewPanel year label text=Year:",
"MachineOverviewPanel purchase date label text=Purchase date:",
"MachineOverviewPanel purchase price label text=Purchase price:",
"MachineOverviewPanel mileage label text=Mileage:"
})
public class MachineOverviewPanel extends JPanel {
private JTextField nameField;
private JComboBox typeComboBox;
private JComboBox brandCombobox;
private JTextField brandField;
private JComboBox modelNumberCombobox;
private JTextField modelNumberField;
private JSpinner yearSpinner;
private JXDatePicker purchaseDataDatePicker;
private JSpinner purchasePriceField;
private JSpinner mileageField;
private FormRow nameRow;
private FormRow typeRow;
private FormRow brandRow;
private FormRow brandCustomRow;
private FormRow modelNumberRow;
private FormRow modelNumberCustomRow;
/**
* Creates new form OverviewPanel
*/
public MachineOverviewPanel() {
setName(Bundle.CTL_OverviewTopComponent());
setToolTipText(Bundle.HINT_OverviewTopComponent());
initComponents();
}
private void initComponents() {
setLayout(new MigLayout("wrap, hidemode 3"));
nameField = new JTextField();
typeComboBox = new JComboBox();
brandCombobox = new JComboBox();
brandField = new JTextField();
modelNumberCombobox = new JComboBox();
modelNumberField = new JTextField();
yearSpinner = new JSpinner(createYearSpinnerModel());
yearSpinner.setEditor(new JSpinner.NumberEditor(yearSpinner, "0000"));
purchaseDataDatePicker = new JXDatePicker(Calendar.getInstance().getTime());
purchasePriceField = new NonNegativeNumberSpinner(100);
mileageField = new NonNegativeNumberSpinner(100);
nameRow = new FormRow(true, Bundle.MachineOverviewPanel_name_label_text(), Bundle.MachineOverviewPanel_name_label_text(), nameField);
typeRow = new FormRow(true, Bundle.MachineOverviewPanel_type_label_text(), Bundle.MachineOverviewPanel_type_label_text(), typeComboBox);
brandRow = new FormRow(true, Bundle.MachineOverviewPanel_brand_label_text(), Bundle.MachineOverviewPanel_brand_label_text(), brandCombobox);
brandCustomRow = new FormRow(true, Bundle.MachineOverviewPanel_brand_label_text(), Bundle.MachineOverviewPanel_brand_label_text(), brandField);
modelNumberRow = new FormRow(true, Bundle.MachineOverviewPanel_model_number_label_text(), Bundle.MachineOverviewPanel_model_number_label_text(), modelNumberCombobox);
modelNumberCustomRow = new FormRow(true, Bundle.MachineOverviewPanel_model_number_label_text(), Bundle.MachineOverviewPanel_model_number_label_text(), modelNumberField);
FormRow yearRow = new FormRow(false, Bundle.MachineOverviewPanel_year_label_text(), Bundle.MachineOverviewPanel_year_label_text(), yearSpinner);
FormRow purchaseDateRow = new FormRow(false, Bundle.MachineOverviewPanel_purchase_date_label_text(), Bundle.MachineOverviewPanel_purchase_date_label_text(), purchaseDataDatePicker);
FormRow purchasePriceRow = new FormRow(false, Bundle.MachineOverviewPanel_purchase_price_label_text(), Bundle.MachineOverviewPanel_purchase_price_label_text(), purchasePriceField);
FormRow mileageRow = new FormRow(false, Bundle.MachineOverviewPanel_mileage_label_text(), Bundle.MachineOverviewPanel_mileage_label_text(), mileageField);
add(nameRow);
add(typeRow);
add(brandRow);
add(brandCustomRow);
add(modelNumberRow);
add(modelNumberCustomRow);
add(yearRow);
add(purchaseDateRow);
add(purchasePriceRow);
add(mileageRow);
}
/**
* Activates the custom input row for brand
*/
public void activateCustomBrandInput() {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
brandCustomRow.setVisible(true);
revalidate();
}
});
}
/**
* Deactivates the custom input row for brand
*/
public void deactivateCustomBrandInput() {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
brandCustomRow.setVisible(false);
revalidate();
}
});
}
/**
* Activates the custom input field for model number
*/
public void activateCustomModelNumberInput() {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
modelNumberCustomRow.setVisible(true);
revalidate();
}
});
}
/**
* deactivates the custom input field for model number
*/
public void deactivateCustomModelNumberInput() {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
modelNumberCustomRow.setVisible(false);
revalidate();
}
});
}
public JComboBox getBrandCombobox() {
return brandCombobox;
}
public JComboBox getModelNumberCombobox() {
return modelNumberCombobox;
}
public JTextField getModelNumberField() {
return modelNumberField;
}
public String getModelNumberValue() {
Object selectedItem = modelNumberCombobox.getSelectedItem();
if (selectedItem == null || selectedItem.equals("")) {
return null;
}
return modelNumberCombobox.getSelectedItem().toString();
}
void setModelNumbers(Object[] models) {
setComboBoxItems(modelNumberCombobox, models);
}
public JTextField getBrandField() {
return brandField;
}
/**
* Gets the value of the brand combobox
*
* @return
*/
public String getBrandValue() {
Object selectedItem = brandCombobox.getSelectedItem();
if (selectedItem == null) {
return null;
}
return brandCombobox.getSelectedItem().toString();
}
void setBrands(Object[] brands) {
setComboBoxItems(brandCombobox, brands);
}
public JSpinner getMileageField() {
return mileageField;
}
public JTextField getNameField() {
return nameField;
}
public JXDatePicker getPurchaseDataDatePicker() {
return purchaseDataDatePicker;
}
public JSpinner getPurchasePriceField() {
return purchasePriceField;
}
void setTypes(Object[] types) {
setComboBoxItems(typeComboBox, types);
}
public JComboBox getTypeComboBox() {
return typeComboBox;
}
public String getTypeValue() {
Object selectedItem = typeComboBox.getSelectedItem();
if (selectedItem == null || selectedItem.equals("")) {
return null;
}
return typeComboBox.getSelectedItem().toString();
}
public JSpinner getYearSpinner() {
return yearSpinner;
}
public FormRow getBrandRow() {
return brandRow;
}
public FormRow getModelNumberRow() {
return modelNumberRow;
}
public FormRow getNameRow() {
return nameRow;
}
public FormRow getTypeRow() {
return typeRow;
}
public FormRow getBrandCustomRow() {
return brandCustomRow;
}
public FormRow getModelNumberCustomRow() {
return modelNumberCustomRow;
}
private void setComboBoxItems(JComboBox comboBox, Object[] items) {
Object previousSelection = comboBox.getSelectedItem();
comboBox.setModel(new DefaultComboBoxModel(items));
if (items.length == 1) { // Just one item selectable, select it
comboBox.setSelectedIndex(0);
} else if (previousSelection != null) {
comboBox.setSelectedItem(previousSelection);
}
}
private static SpinnerModel createYearSpinnerModel() {
final int currentYear = Calendar.getInstance().get(Calendar.YEAR);
return new SpinnerNumberModel(currentYear, 0, currentYear, 1);
}
private class NonNegativeNumberSpinner extends JSpinner {
private int width;
public NonNegativeNumberSpinner(int width) {
super(new SpinnerNumberModel(0, 0, null, 1));
setEditor(new JSpinner.NumberEditor(this));
this.width = width;
}
@Override
public Dimension getPreferredSize() {
Dimension d = super.getPreferredSize();
return new Dimension(width, d.height);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy