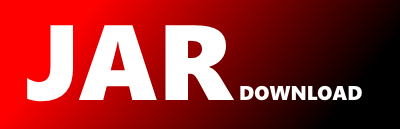
nl.cloudfarming.client.fleet.machine.wizard.MachineDynamicWizardPanel Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package nl.cloudfarming.client.fleet.machine.wizard;
import java.beans.PropertyEditor;
import java.beans.PropertyEditorSupport;
import java.lang.reflect.InvocationTargetException;
import java.util.*;
import java.util.logging.Logger;
import nl.cloudfarming.client.fleet.machine.MachineDataService.StepInfo;
import nl.cloudfarming.client.fleet.model.Machine;
import nl.cloudfarming.client.fleet.model.MachinePropertyDefinition;
import nl.cloudfarming.client.fleet.model.MachineProperty;
import org.openide.WizardDescriptor;
import org.openide.nodes.Node;
import org.openide.nodes.Node.Property;
/**
* An optional extra panel which can be added to the machine wizard.
*
* @author Wytse Visser
*/
class MachineDynamicWizardPanel extends MachineWizardPanel {
private StepInfo stepInfo;
private boolean propertiesAdded = false;
private MachineDynamicVisualPanel visualPanel;
private List properties;
public static final String PROP_DYNAMIC_PROPMAP = "dynamic properties map";
private static final Logger LOGGER = Logger.getLogger("nl.cloudfarming.client.fleet.vehicle.wizard");
/**
* Creates the MachineDynamicWizardPanel. The content of this panel is based
* on the given StepInfo object.
*
* @param stepInfo The StepInfo with the name and properties for this panel.
*/
public MachineDynamicWizardPanel(StepInfo stepInfo) {
this.stepInfo = stepInfo;
}
@Override
protected MachineDynamicVisualPanel getVisual() {
if (visualPanel == null) {
visualPanel = new MachineDynamicVisualPanel();
visualPanel.setName(stepInfo.getName());
}
return visualPanel;
}
@Override
public void readSettings(WizardDescriptor wiz) {
setTitle(((Machine) wiz.getProperty(MachineWizardIterator.PROP_MACHINE)).getName());
if (!propertiesAdded) {
properties = new ArrayList<>();
for (MachinePropertyDefinition machineProperty : stepInfo.getProperties()) {
try {
MachineProperty propertyValueHolder = new MachineProperty(machineProperty.getDefaultValue(), machineProperty);
Property property = propertyValueHolder.getProperty();
properties.add(propertyValueHolder);
getVisual().addPropertyField(property);
} catch (IllegalAccessException | InvocationTargetException ex) {
LOGGER.warning("Could not add property to visual panel");
}
}
propertiesAdded = true;
}
}
@Override
public void storeSettings(WizardDescriptor wiz) {
Map> map = getDynamicPropertyMap(wiz);
map.put(this, properties);
wiz.putProperty(PROP_DYNAMIC_PROPMAP, map);
}
/**
* Returns the map with dynamic properties of the given WizardDescriptor.
* Creates a new one if none exists.
*
* @param wiz The WizardDescriptor to get the map from
* @return The dynamic property map
*/
public static Map> getDynamicPropertyMap(WizardDescriptor wiz) {
Map> map;
if (wiz.getProperty(PROP_DYNAMIC_PROPMAP) == null) {
map = new HashMap<>();
} else {
map = (Map>) wiz.getProperty(PROP_DYNAMIC_PROPMAP);
}
return map;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
final MachineDynamicWizardPanel other = (MachineDynamicWizardPanel) obj;
if (!Objects.equals(getVisual().getName(), other.getVisual().getName())) {
return false;
}
return true;
}
@Override
public int hashCode() {
int hash = 7;
return hash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy