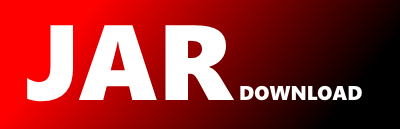
nl.cloudfarming.client.fleet.machine.wizard.MachineWizardAction Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package nl.cloudfarming.client.fleet.machine.wizard;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.IOException;
import java.text.MessageFormat;
import java.util.List;
import javax.swing.AbstractAction;
import nl.cloudfarming.client.fleet.machine.MachineDataService;
import nl.cloudfarming.client.fleet.model.Machine;
import nl.cloudfarming.client.fleet.model.MachineProperty;
import org.netbeans.api.project.Project;
import org.openide.DialogDisplayer;
import org.openide.WizardDescriptor;
import org.openide.nodes.Node.Property;
import org.openide.util.Exceptions;
/**
* This action can be called to start the machine creation wizard.
* Implementations for different machine types should extends this class.
*
* @author Wytse Visser
*/
public abstract class MachineWizardAction extends AbstractAction implements ActionListener {
private Project project;
/**
* Creates a new MachineWizardAction.
*
* @param project The project the action belongs to.
* @param name The name of the action. (e.g. "New Machine")
*/
public MachineWizardAction(Project project, String name) {
this.project = project;
putValue(NAME, name);
}
/**
* Performs this action. Starts a new machine creation wizard and creates
* the machine when the wizard finishes.
*
* @param e The event calling this action.
*/
@Override
public void actionPerformed(ActionEvent e) {
WizardDescriptor wiz = new WizardDescriptor(getWizardFactory().createWizardIterator());
// {0} will be replaced by WizardDescriptor.Panel.getComponent().getName()
// {1} will be replaced by WizardDescriptor.Iterator.name()
wiz.setTitleFormat(new MessageFormat("{0} ({1})"));
wiz.setTitle(getValue(NAME).toString());
if (DialogDisplayer.getDefault().notify(wiz) == WizardDescriptor.FINISH_OPTION) {
try {
createMachine(wiz);
} catch (IOException ex) {
Exceptions.printStackTrace(ex);
}
}
}
protected Project getProject() {
return project;
}
/**
* Returns the value of the given property in the wizard descriptor. Falls
* back to the value in the model if the property is not set in the wizard
* descriptor.
*
* @param propertyName The name of the property to read the value for
* @param wiz The wizard descriptor the property is initally read from
* @param model The model to fall back to
* @return The stored value for the property
*/
protected static Object readProperty(String propertyName, WizardDescriptor wiz, MachineDataService.Model model) {
Object value = wiz.getProperty(propertyName);
if (value == null) {
value = model.getProperty(propertyName);
}
return value;
}
/**
* Creates and returns the MachineWizardImplementation for this action.
*
* @return The MachineWizardImplementation for this action
*/
protected abstract MachineWizardFactory getWizardFactory();
/**
* Create and save a new machine with the values stored in the given
* WizardDescriptor.
*
* @param wiz The WizardDescriptor with the values the user entered using
* the wizard.
* @throws IOException If somehow the machine can't be saved.
*/
protected abstract void createMachine(WizardDescriptor wiz) throws IOException;
/**
* Adds dynamic properties stored in the WizardDescriptor to the machine.
*
* @param machine The machine
* @param wiz The wizard descriptor with the dynamic properties
*/
protected void addDynamicProperties(Machine machine, WizardDescriptor wiz) {
for (List propertyList : MachineDynamicWizardPanel.getDynamicPropertyMap(wiz).values()) {
for (MachineProperty property : propertyList) {
machine.addProperty(property);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy