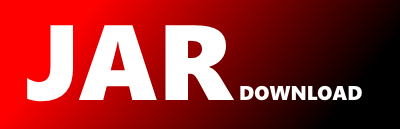
nl.cloudfarming.client.fleet.machine.wizard.MachineWizardOverviewPanel Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify it under
* the terms of the GNU General Public License as published by the Free Software
* Foundation, either version 3 of the License, or (at your option) any later
* version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it
* is applied to this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License along with
* AgroSense. If not, see .
*/
package nl.cloudfarming.client.fleet.machine.wizard;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import nl.cloudfarming.client.fleet.machine.MachineDataService;
import nl.cloudfarming.client.fleet.model.Machine;
import nl.cloudfarming.client.fleet.model.MachineProperty;
import org.openide.WizardDescriptor;
import org.openide.util.NbBundle;
/**
*
* @author Wytse Visser
*/
@NbBundle.Messages({"MachineWizardOverviewPanel name label text=Name",
"MachineWizardOverviewPanel type label text=Type",
"MachineWizardOverviewPanel brand label text=Brand",
"MachineWizardOverviewPanel model number label text=Model number",
"MachineWizardOverviewPanel year label text=Year",
"MachineWizardOverviewPanel purchase date label text=Purchase date",
"MachineWizardOverviewPanel purchase price label text=Purchase price",
"MachineWizardOverviewPanel mileage label text=Mileage",})
public final class MachineWizardOverviewPanel extends MachineWizardPanel {
private MachineVisualOverviewPanel visualPanel;
private Set propertyNames;
@Override
protected MachineVisualOverviewPanel getVisual() {
if (visualPanel == null) {
visualPanel = new MachineVisualOverviewPanel();
}
return visualPanel;
}
@Override
public void readSettings(WizardDescriptor wiz) {
getVisual().removeAll();
Machine machine = (Machine) wiz.getProperty(MachineWizardIterator.PROP_MACHINE);
setTitle(machine.getName());
getVisual().addProperty(Bundle.MachineWizardOverviewPanel_name_label_text(), machine.getName());
MachineDataService.Model model = (MachineDataService.Model) wiz.getProperty(MachineWizardIterator.PROP_MODEL);
if (model != null) {
getVisual().addProperty(Bundle.MachineWizardOverviewPanel_type_label_text(), model.getType());
getVisual().addProperty(Bundle.MachineWizardOverviewPanel_brand_label_text(), model.getBrand());
getVisual().addProperty(Bundle.MachineWizardOverviewPanel_model_number_label_text(), model.getModelNumber());
}
getVisual().addProperty(Bundle.MachineWizardOverviewPanel_year_label_text(), machine.getYear());
getVisual().addProperty(Bundle.MachineWizardOverviewPanel_purchase_date_label_text(), machine.getPurchaseDate());
getVisual().addProperty(Bundle.MachineWizardOverviewPanel_purchase_price_label_text(), machine.getPurchasePrice());
getVisual().addProperty(Bundle.MachineWizardOverviewPanel_mileage_label_text(), machine.getMileage());
for (PropertyEntry entry : getPropertyNames()) {
String humanReadableName = entry.getHumanReadableName();
Object value = wiz.getProperty(entry.getName());
getVisual().addProperty(humanReadableName, value);
}
addDynamicProperties(wiz);
}
public void addProperty(String name, String humanReadableName) {
getPropertyNames().add(new PropertyEntry(name, humanReadableName));
}
private void addDynamicProperties(WizardDescriptor wiz) {
for (List propertyLists : MachineDynamicWizardPanel.getDynamicPropertyMap(wiz).values()) {
for (MachineProperty propertyValueHolder : propertyLists) {
getVisual().addProperty(propertyValueHolder.getProperty());
}
}
}
@Override
public void storeSettings(WizardDescriptor wiz) {
// Can't change anything on this panel, nothing to store
}
private Set getPropertyNames() {
if (propertyNames == null) {
propertyNames = new HashSet<>();
}
return propertyNames;
}
private class PropertyEntry {
private String name;
private String humanReadableName;
public PropertyEntry(String name, String humanReadableName) {
this.name = name;
this.humanReadableName = humanReadableName;
}
String getName() {
return name;
}
String getHumanReadableName() {
return humanReadableName;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy