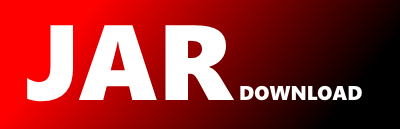
nl.cloudfarming.client.fleet.machine.wizard.MachineWizardStartPanel Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.fleet.machine.wizard;
import java.awt.Component;
import java.util.HashSet;
import java.util.Set;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
import nl.cloudfarming.client.fleet.machine.windows.MachineOverviewController;
import nl.cloudfarming.client.fleet.machine.windows.MachineOverviewPanel;
import nl.cloudfarming.client.fleet.model.Machine;
import nl.cloudfarming.client.fleet.model.MachineProvider;
import nl.cloudfarming.client.util.swing.ASBodyPanel;
import org.netbeans.api.project.Project;
import org.openide.WizardDescriptor;
import org.openide.WizardValidationException;
import org.openide.util.HelpCtx;
import org.openide.util.ImageUtilities;
import org.openide.util.Lookup;
import org.openide.util.NbBundle;
/**
* The panel for the initial step in the machine creation wizard.
*
* @author Wytse Visser
*/
@NbBundle.Messages({"MachineWizardStartPanel general properties step name=General details",
"MachineWizardStartPanel invalid input message=There are some errors please correct them"})
public class MachineWizardStartPanel extends MachineOverviewController implements WizardDescriptor.ValidatingPanel, WizardDescriptor.FinishablePanel {
private final Set listeners = new HashSet<>(1);
private MachineWizardFactory factory;
private Machine machineDataHolder;
private ASBodyPanel overviewDecorator;
public MachineWizardStartPanel(MachineWizardFactory factory, Machine machineDataHolder) {
super(new MachineOverviewPanel(), machineDataHolder, Lookup.getDefault().lookupAll(factory.getDataServiceClass()));
this.factory = factory;
this.machineDataHolder = machineDataHolder;
}
@Override
public boolean isValid() {
return true;
}
@Override
public void validate() throws WizardValidationException{
if(!isPanelValid()){
super.validatePanel();
throw new WizardValidationException(overviewDecorator, Bundle.MachineWizardStartPanel_invalid_input_message(), Bundle.MachineWizardStartPanel_invalid_input_message());
}
}
@Override
public void addChangeListener(ChangeListener l) {
synchronized (listeners) {
listeners.add(l);
}
}
@Override
public void removeChangeListener(ChangeListener l) {
synchronized (listeners) {
listeners.remove(l);
}
}
protected final void fireChangeEvent() {
Set ls;
synchronized (listeners) {
ls = new HashSet<>(listeners);
}
ChangeEvent ev = new ChangeEvent(this);
for (ChangeListener l : ls) {
l.stateChanged(ev);
}
}
@Override
public void readSettings(WizardDescriptor wiz) {
}
@Override
public void storeSettings(WizardDescriptor wiz) {
wiz.putProperty(MachineWizardIterator.PROP_MACHINE, machineDataHolder);
wiz.putProperty(MachineWizardIterator.PROP_MODEL, getSelectedModel());
}
@Override
public MachineProvider getMachineProvider() {
return Lookup.getDefault().lookup(factory.getMachineProviderClass());
}
@Override
public Project getProject() {
return factory.getProject();
}
@Override
public void fieldsUpdated() {
fireChangeEvent();
overviewDecorator.setTitle(getOverviewPanel().getNameField().getText());
}
@Override
public boolean isFinishPanel() {
return isPanelValid();
}
@Override
public Component getComponent() {
if (overviewDecorator == null) {
MachineOverviewPanel overviewPanel = getOverviewPanel();
overviewDecorator = new ASBodyPanel(overviewPanel);
overviewDecorator.setName(Bundle.MachineWizardStartPanel_general_properties_step_name());
overviewDecorator.setIcon(ImageUtilities.loadImageIcon("nl/cloudfarming/client/fleet/vehicle/steering_wheel_332.png", true));
}
return overviewDecorator;
}
@Override
public HelpCtx getHelp() {
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy