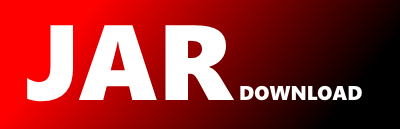
nl.cloudfarming.client.geoviewer.jxmap.map.MapWidgetFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geoviewer-jxmap Show documentation
Show all versions of geoviewer-jxmap Show documentation
AgroSense geoviewer JXMap implementation. Contains a map/geoviewer TopComponent based on the JXMap classes from swingx.
/**
* Copyright (C) 2010-2012 Agrosense [email protected]
*
* Licensed under the Eclipse Public License - v 1.0 (the "License"); you may
* not use this file except in compliance with the License. You may obtain a
* copy of the License at
*
* http://www.eclipse.org/legal/epl-v10.html
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package nl.cloudfarming.client.geoviewer.jxmap.map;
import java.awt.Point;
import java.lang.reflect.TypeVariable;
import java.util.logging.Logger;
import javax.swing.JPopupMenu;
import nl.cloudfarming.client.geoviewer.Geographical;
import nl.cloudfarming.client.geoviewer.Geometrical;
import nl.cloudfarming.client.geoviewer.Layer;
import nl.cloudfarming.client.geoviewer.render.GeometricalWidget;
import nl.cloudfarming.client.geoviewer.jxmap.render.JXMapGeoTranslator;
import nl.cloudfarming.client.geoviewer.render.GeographicalWidget;
import nl.cloudfarming.client.geoviewer.render.GridCoverage2DWidget;
import org.geotools.coverage.grid.GridCoverage2D;
import org.netbeans.api.visual.action.ActionFactory;
import org.netbeans.api.visual.action.PopupMenuProvider;
import org.netbeans.api.visual.widget.Widget;
import org.openide.nodes.Node;
import org.openide.util.Utilities;
/**
* factory class for the creation of map widgets
*
*
* @author Timon Veenstra
*/
public class MapWidgetFactory {
private static final Logger LOGGER = Logger.getLogger("nl.cloudfarming.client.geoviewer.jxmap.map.MapWidgetFactory");
/**
* Create a widget from a node and a scene
*
* @param node node to create a widgets for
* @param scene scene to create the widget on
* @return the created widget
*/
static Widget createWidgetFromNode(final Node node, MapScene scene) {
Geometrical geometrical = node.getLookup().lookup(Geometrical.class);
Layer layer = Layer.Finder.findLayer(node);
if (layer == null) {
LOGGER.warning("FIXME:Attempt to create a widget from a node without a layer in its hierarchy.");
return null;
}
if (scene.getObjects().contains(node)) {
LOGGER.warning("FIXME:Attempt to create a widget from a node which is already present in the scene");
return null;
}
if (geometrical == null) {
Geographical geographical = node.getLookup().lookup(Geographical.class);
if (geographical == null) {
LOGGER.finest("Attempt to create a widget from a node without a geographical or geometrical, could be a parent node");
return null;
} else {
// for (TypeVariable tv : geographical.getClass().getTypeParameters()) {
// if (tv.getGenericDeclaration().getClass().equals(GridCoverage2D.class)) {
// LOGGER.finest("Creating GridCoverage2DWidget");
// GridCoverage2DWidget widget = new GridCoverage2DWidget(layer.getName(), geographical, layer.getPalette(), geographical.getBoundingBox(), new JXMapGeoTranslator(scene.getMapViewer()), scene);
// return widget;
// }
// }
//FIXME does not always have to result in a GridCoverage2DWidget, unsure how to make this more generic. Only support GridCoverage2DWidget by now
LOGGER.finest("Creating GridCoverage2DWidget");
GridCoverage2DWidget widget = new GridCoverage2DWidget(layer.getName(), geographical, layer.getPalette(), geographical.getBoundingBox(), new JXMapGeoTranslator(scene.getMapViewer()), scene);
return widget;
}
}
GeometricalWidget widget = new GeometricalWidget(scene, new JXMapGeoTranslator(scene.getMapViewer()), geometrical, layer.getPalette(), null);
widget.getActions().addAction(scene.createObjectHoverAction());
widget.getActions().addAction(scene.createWidgetHoverAction());
widget.getActions().addAction(scene.createSelectAction());
widget.getActions().addAction(ActionFactory.createPopupMenuAction(new PopupMenuProvider() {
@Override
public JPopupMenu getPopupMenu(Widget widget, Point localLocation) {
JPopupMenu menu = Utilities.actionsToPopup(node.getActions(true), node.getLookup());
return menu;
}
}));
return widget;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy