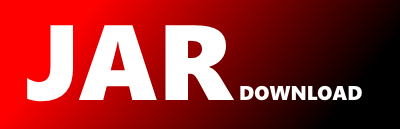
nl.cloudfarming.client.geoviewer.jxmap.layerlist.LayerListNode Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.geoviewer.jxmap.layerlist;
import java.awt.Image;
import java.awt.event.ActionEvent;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.swing.Action;
import nl.cloudfarming.client.geoviewer.*;
import nl.cloudfarming.client.geoviewer.jxmap.MapProperties;
import nl.cloudfarming.client.geoviewer.jxmap.map.CreateGeometryAction;
import nl.cloudfarming.client.geoviewer.jxmap.map.SplitGeometryAction;
import org.openide.actions.ToolsAction;
import org.openide.nodes.FilterNode;
import org.openide.nodes.Node;
import org.openide.util.ImageUtilities;
import org.openide.util.actions.SystemAction;
/**
*
* @author Timon Veenstra
*/
class LayerListNode extends FilterNode implements LayerListRemovableNode {
private final LayerInfo info;
private final SettingsForLayerAction settingsAction;
private boolean visible = true;
@Override
public Image getIcon(int type) {
Image icon = getOriginal().getIcon(type);
if (!visible) {
icon = ImageUtilities.createDisabledImage(icon);
}
return icon;
}
LayerListNode(LayerListController.LayerWrapper layerWrapper) {
super(layerWrapper.getLayerNode(), new FilterLayerListChildren(layerWrapper.getLayerNode()));
this.info = layerWrapper.getLayerInfo();
Layer layer = getLookup().lookup(Layer.class);
settingsAction = new SettingsForLayerAction(info, layer);
visible = info.isVisible();
initListener();
}
private void initListener() {
info.addPropertyChangeListener(LayerInfo.PROPERTY_VISIBLE, new PropertyChangeListener() {
@Override
public void propertyChange(PropertyChangeEvent evt) {
visible = (Boolean) evt.getNewValue();
}
});
}
@Override
public Action[] getActions(boolean context) {
final Layer layer = getLookup().lookup(Layer.class);
List superActions = new ArrayList();
//Remove the ToolsAction from the list
if (super.getActions(context) != null && super.getActions(context).length > 0) {
//New ArrayList because of abstractList return
List actions = new ArrayList(Arrays.asList(super.getActions(context)));
for (int i = 0; i < actions.size(); i++) {
if (actions.get(i) instanceof ToolsAction) {
actions.remove(i);
}
}
superActions.addAll(actions);
}
superActions.addAll(getMapActions());
superActions.addAll(getGeometryActions(layer));
superActions.addAll(getLayerActions());
return superActions.toArray(new Action[superActions.size()]);
}
private List getMapActions() {
List actions = new ArrayList<>();
DynamicGeometrical dynamicGeometrical = getLookup().lookup(DynamicGeometrical.class);
if (dynamicGeometrical != null) {
actions.addAll(Arrays.asList(dynamicGeometrical.getMapActions()));
}
return actions;
}
private List getGeometryActions(Layer layer) {
List actions = new ArrayList<>();
if (layer instanceof SingleObjectLayer) {
actions.add(new CreateGeometryAction((SingleObjectLayer) layer));
if (getLookup().lookup(SplitGeometryHandler.class) != null) {
actions.add(new SplitGeometryAction(this));
}
}
Geographical geographical = getLookup().lookup(Geographical.class);
if (geographical != null) {
actions.add(new SelectFieldInMapAction(geographical));
}
return actions;
}
private List getLayerActions() {
List actions = new ArrayList<>();
if (MapProperties.getLayerNodeRemoveActionEnabledValue()) {
actions.add(SystemAction.get(RemoveLayerAction.class));
}
if (MapProperties.getLayerNodeSettingsActionEnabledValue()) {
actions.add(settingsAction);
}
if (MapProperties.getLayerNodevisibilityActionEnabledValue()) {
actions.add(new VisibilityLayerAction(info) {
@Override
public void actionPerformed(ActionEvent ae) {
super.actionPerformed(ae);
fireIconChange();
}
});
}
return actions;
}
@Override
public Action getPreferredAction() {
Geographical geographical = getLookup().lookup(Geographical.class);
if (geographical != null) {
return new SelectFieldInMapAction(geographical);
} else {
return settingsAction;
}
}
@Override
public Node getRemovableNode() {
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy