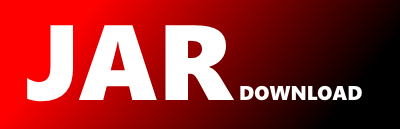
nl.cloudfarming.client.geoviewer.render.PolygonRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of geoviewer-render Show documentation
Show all versions of geoviewer-render Show documentation
AgroSense geoviewer render - contains classes for rendering of various geo objects
The newest version!
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.geoviewer.render;
import com.vividsolutions.jts.awt.PolygonShape;
import com.vividsolutions.jts.geom.Coordinate;
import com.vividsolutions.jts.geom.Geometry;
import com.vividsolutions.jts.geom.Polygon;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.Paint;
import java.awt.RadialGradientPaint;
import java.awt.Rectangle;
import java.awt.Shape;
import java.awt.geom.Ellipse2D;
import java.awt.geom.Point2D;
import java.util.ArrayList;
import java.util.Collection;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.jdesktop.swingx.mapviewer.GeoPosition;
import org.netbeans.api.visual.model.ObjectState;
/**
* Render a polygon, including holes.
*
* @author johan
*/
public class PolygonRenderer extends GeometryRenderer {
private static final Logger LOGGER = Logger.getLogger(PolygonRenderer.class.getName());
public static final int ALPHA_SELECTED = (int) (255 * .5);//50%
private static final double DEFAULT_DIFFERENCE = 0.0002; //what does this number mean?
@Override
public void render(Polygon geometry, Geometry boundingBox, Rectangle canvas, GeoTranslator translator, ObjectState state, Graphics2D g) {
Coordinate[] shell = translate(geometry.getExteriorRing().getCoordinates(), boundingBox, canvas, translator);
Collection holes = new ArrayList(geometry.getNumInteriorRing());
for (int i = 0; i < geometry.getNumInteriorRing(); i++) {
holes.add(translate(geometry.getInteriorRingN(i).getCoordinates(), boundingBox, canvas, translator));
}
PolygonShape polygonShape = new PolygonShape(shell, holes);
Color org = g.getColor();
if (state.isSelected()) {
//
// gradient fill for the selected polygon, makes selection more visible when zoomed out.
//
Point2D edge = new Point2D.Double(polygonShape.getBounds2D().getMinX(), polygonShape.getBounds2D().getMinY());
Point2D centroid = new Point2D.Double(polygonShape.getBounds2D().getCenterX(), polygonShape.getBounds2D().getCenterY());
LOGGER.log(Level.FINEST, "object selected, edge={0}, centroid={1}", new Object[]{edge, centroid});
// corner case for tiny geometries and/or zooming out very far (IllegalArgumentException: Radius must be greater than zero)
if (!edge.equals(centroid)) {
Paint p = new RadialGradientPaint(centroid, (float)centroid.distance(edge), new float[]{0.0f,0.7f}, new Color[]{Color.red,org});
g.setPaint(p);
}
}
//
// fill the polygon
//
g.fill(polygonShape);
g.setPaint(org);
if (state.isSelected()) {
//
// calculate zoom level and resize factor
//
// - take 2 awt point with a 10 pixel distance.
// - convert those pixels to geo positions
// - calculate distance between the geo positions
// (it's not really the distance but rather the difference in coordinates)
// - the ratio between the distance in pixels and the distance in geo positions
// determines the zoom level. By inverting this number with a max of 1 we get a
// resize factor.
//
//
Point2D left = new java.awt.Point(0, 0);
GeoPosition leftPosition = translator.pixelToGeo(canvas, boundingBox, left);
Point2D slightlyMoreToTheRight = new java.awt.Point(10, 0);
GeoPosition rightPosition = translator.pixelToGeo(canvas, boundingBox, slightlyMoreToTheRight);
final double difference = rightPosition.getLongitude() - leftPosition.getLongitude();
double zoomLevel = difference / DEFAULT_DIFFERENCE;
final double iconResizeFactor = 1.0 / Math.max(zoomLevel /*- 2*/, 1);
g.setStroke(
new ShapeStroke(
new Shape[]{
/* new Star2D(0.0, 0.0, 2.0*(float)iconResizeFactor, 5.0*(float)iconResizeFactor, 5),*/
new Ellipse2D.Float(0, 0, 4*(float)iconResizeFactor, 4*(float)iconResizeFactor)
},
10.0f*(float)iconResizeFactor));
g.setColor(Color.black);
g.draw(polygonShape);
g.setColor(org);
}
}
private Coordinate[] translate(Coordinate[] coordinates, Geometry boundingBox, Rectangle canvas, GeoTranslator translator) {
Coordinate[] translated = new Coordinate[coordinates.length];
for (int i = 0; i < coordinates.length; i++) {
Point2D point2D = translator.geoToPixel(canvas, boundingBox, coordinates[i]);
double x = point2D.getX() - canvas.getBounds().getX();
double y = point2D.getY() - canvas.getBounds().getY();
translated[i] = new Coordinate(x, y);
}
return translated;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy