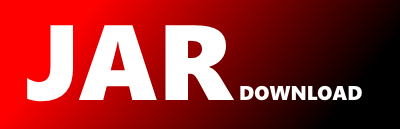
nl.cloudfarming.client.spray.SprayTaskDataObject Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.spray;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import java.io.IOException;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.swing.Icon;
import nl.cloudfarming.client.model.Entity;
import nl.cloudfarming.client.model.EntitySavable;
import nl.cloudfarming.client.spray.tree.SprayTaskNode;
import org.netbeans.api.actions.Savable;
import org.openide.filesystems.FileObject;
import org.openide.loaders.DataObjectExistsException;
import org.openide.loaders.MultiDataObject;
import org.openide.loaders.MultiFileLoader;
import org.openide.nodes.Node;
import org.openide.util.ImageUtilities;
import org.openide.util.Lookup;
import org.openide.util.LookupEvent;
import org.openide.util.LookupListener;
import org.openide.util.lookup.AbstractLookup;
import org.openide.util.lookup.InstanceContent;
import org.openide.util.lookup.ProxyLookup;
public class SprayTaskDataObject extends MultiDataObject implements PropertyChangeListener, LookupListener {
private static final Logger LOGGER = Logger.getLogger(SprayTaskDataObject.class.getCanonicalName());
private InstanceContent ic;
private AbstractLookup intern;
private SprayTask sprayTask;
private SprayTaskManager sprayTaskManager;
public SprayTaskDataObject(FileObject pf, MultiFileLoader loader) throws DataObjectExistsException, IOException {
super(pf, loader);
registerEditor(SprayTask.MIME_TYPE, true);
ic = new InstanceContent();
intern = new AbstractLookup(this.ic);
sprayTaskManager = Lookup.getDefault().lookup(SprayTaskManager.class);
assert sprayTaskManager != null : "No SprayTaskManager class found in the default lookup, unable to load the DataObject";
sprayTask = sprayTaskManager.loadTask(getPrimaryFile());
assert sprayTask != null;
assert sprayTask.getLookup() != null : "SprayTask lookup is null, not properly deserialized";
LOGGER.log(Level.FINE, "SprayTaskDataObject loaded {0}", System.identityHashCode(sprayTask));
ic.add(sprayTask);
sprayTask.addPropertyChangeListener(this);
sprayTask.getLookup().lookupResult(Entity.class).addLookupListener(this);
}
@Override
protected int associateLookup() {
return 1;
}
@Override
protected Node createNodeDelegate() {
return new SprayTaskNode(super.createNodeDelegate(), sprayTask);
}
@Override
public Lookup getLookup() {
return new ProxyLookup(super.getLookup(), sprayTask.getLookup(), intern);
}
@Override
protected void handleDelete() throws IOException {
super.handleDelete();
SprayTaskSavable existingSavable = getLookup().lookup(SprayTaskSavable.class);
if (existingSavable != null) {
existingSavable.destroy();
}
}
@Override
public void propertyChange(PropertyChangeEvent evt) {
Savable existingSavable = getLookup().lookup(Savable.class);
if (existingSavable == null) {
ic.add(new SprayTaskSavable());
}
}
@Override
public void resultChanged(LookupEvent ev) {
propertyChange(null);
}
private static final Icon ICON = ImageUtilities.loadImageIcon("nl/cloudfarming/client/spray/to_do_list_checked_1.png", true);
private class SprayTaskSavable extends EntitySavable {
public SprayTaskSavable() {
super(sprayTask, ICON);
register();
}
@Override
protected String findDisplayName() {
return sprayTask.getName();
}
@Override
protected void handleSave() throws IOException {
getDataObject().sprayTaskManager.save(sprayTask);
destroy();
}
@Override
public void destroy() {
getDataObject().ic.remove(SprayTaskSavable.this);
unregister();
}
@Override
public void dispose() {
getDataObject().dispose();
}
@Override
public boolean equals(Object obj) {
if (obj instanceof SprayTaskSavable) {
SprayTaskSavable savable = (SprayTaskSavable) obj;
return getDataObject() == savable.getDataObject();
}
return false;
}
private SprayTaskDataObject getDataObject() {
return SprayTaskDataObject.this;
}
@Override
public int hashCode() {
return getDataObject().hashCode();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy