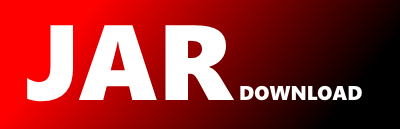
nl.cloudfarming.client.spray.SprayTaskManager Maven / Gradle / Ivy
/**
* Copyright (C) 2008-2012 AgroSense Foundation.
*
* AgroSense is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* There are special exceptions to the terms and conditions of the GPLv3 as it is applied to
* this software, see the FLOSS License Exception
* .
*
* AgroSense is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with AgroSense. If not, see .
*/
package nl.cloudfarming.client.spray;
import java.io.*;
import java.util.EnumSet;
import java.util.Set;
import java.util.logging.Level;
import java.util.logging.Logger;
import nl.cloudfarming.client.model.AgroURI;
import nl.cloudfarming.client.model.ItemIdType;
import nl.cloudfarming.client.task.TaskManager;
import org.netbeans.api.project.Project;
import org.openide.filesystems.FileObject;
import org.openide.util.io.NbObjectInputStream;
import org.openide.util.lookup.ServiceProvider;
/**
*
* @author Maciek Dulkiewicz
*/
@ServiceProvider(service = TaskManager.class)
public class SprayTaskManager extends TaskManager {
private static final Logger LOGGER = Logger.getLogger("nl.cloudfarming.client.spray");
public static final String SPRAY_TASK_DIR_NAME = "SprayTasks";
public void addSprayTask(SprayTask sprayTask) {
save(sprayTask);
getPropertyChangeSupport().firePropertyChange(PROP_TASK_ADDED, null, sprayTask);
}
@Override
public FileObject getStorageFolder(Project project) {
FileObject tasksDir = getTaskDirectory(project);
FileObject sprayTaskDir = tasksDir.getFileObject(SPRAY_TASK_DIR_NAME);
if (sprayTaskDir == null) {
try {
sprayTaskDir = tasksDir.createFolder(SPRAY_TASK_DIR_NAME);
} catch (IOException ex) {
LOGGER.log(Level.WARNING, "Unable to create SprayTask directory {0}", ex.getMessage());
}
}
return sprayTaskDir;
}
@Override
protected SprayTask load(AgroURI uri, Project project) {
if (uri.getItemIdType().equals(ItemIdType.STK)) {
FileObject taskFile = getStorageFolder(project).getFileObject(uri.getItemIdNumber(), SprayTask.EXT);
if (taskFile != null) {
return loadTask(taskFile);
}
}
LOGGER.log(Level.WARNING, "Passed AgroURI is not of type SprayTask {0}", uri);
return null;
}
public SprayTask loadTask(FileObject fileObject) {
LOGGER.log(Level.FINEST, "loading SprayTask object from file {0}", fileObject.getName());
assert SprayTask.EXT.equals(fileObject.getExt()) : "Can only load SprayTasks";
Object object = null;
// use of NbObjectInputStream is needed because SprayTask has members from a different module
// normal ObjectInputStream uses the module classloader and is not able to see those classes
try (ObjectInputStream ois = new NbObjectInputStream(fileObject.getInputStream())) {
object = ois.readObject();
} catch (ClassNotFoundException | IOException ex) {
LOGGER.log(Level.SEVERE, "Could not load file {0} with the exception {1}", new Object[]{fileObject, ex});
}
assert object instanceof SprayTask : "FileObject did not contain the right type (SprayTask) but a " + object.getClass();
SprayTask sprayTask = (SprayTask) object;
return getCachedInstanceFor(sprayTask);
}
@Override
public void save(SprayTask task) {
Project project = TaskManager.findProject(task.getURI());
save(task, project);
}
private void save(SprayTask task, Project project) {
FileObject storageFolder = getStorageFolder(project);
FileObject taskFileObject = storageFolder.getFileObject(task.getItemIdNumber(), SprayTask.EXT);
try {
if (taskFileObject == null) {
String taskFileName = task.getItemIdNumber() + "." + SprayTask.EXT;
try (OutputStream os = storageFolder.createAndOpen(taskFileName)) {
save(task, os);
}
} else {
save(task, taskFileObject);
}
} catch (IOException ex) {
LOGGER.log(Level.SEVERE, "Could not create SprayTask file {0} with the exception {1}", new Object[]{task.getItemIdNumber(), ex});
}
}
private void save(SprayTask task, OutputStream outputStream) {
try (ObjectOutputStream oos = new ObjectOutputStream(outputStream)) {
oos.writeObject(task);
addToCache(task);
} catch (IOException ex) {
LOGGER.log(Level.SEVERE, "Could not serialize SprayTask to file {0} with the exception {1}", new Object[]{task.getItemIdNumber(), ex});
}
}
private void save(SprayTask task, FileObject targetFile) {
LOGGER.log(Level.FINEST, "saving SprayTask object to file {0}", targetFile.getName());
assert SprayTask.EXT.equals(targetFile.getExt()) : "Can only save SprayTasks";
try (ObjectOutputStream oos = new ObjectOutputStream(targetFile.getOutputStream())) {
oos.writeObject(task);
} catch (IOException ex) {
LOGGER.log(Level.SEVERE, "Could not save file {0} with the exception {1}", new Object[]{targetFile, ex});
}
}
@Override
public Set getSupportedTypes() {
return EnumSet.of(ItemIdType.STK);
}
@Override
public void remove(SprayTask entity) {
throw new UnsupportedOperationException("Not supported yet.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy