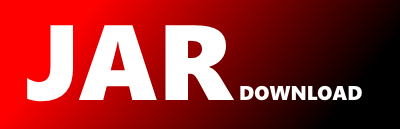
nl.demon.shadowland.freedumbytes.swingx.gui.modal.JModalConfiguration Maven / Gradle / Ivy
package nl.demon.shadowland.freedumbytes.swingx.gui.modal;
import java.awt.*;
import javax.swing.*;
import nl.demon.shadowland.freedumbytes.swingx.gui.utility.*;
/**
* Component that is used to configure the different window-specific related settings.
* Project: JModalWindow - window-specific modality.
* Copyright: Copyright (c) 2001-2006.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* @author Jene Jasper
* @version 2.0
*/
public class JModalConfiguration
{
private int blurStyle;
private Color blurColor;
private Cursor busyCursor;
private boolean busyCursorEnabled;
private Image optionalFrameIcon;
private Icon optionalIFrameIcon;
private boolean windowDragEnabled;
private int windowDragBorderDistance;
private int windowDragScreenSafetyMargin;
private boolean alsoKeepModalToWindowInFront;
private boolean allowIconifyForBlockedInternalFrame;
private boolean enableWaitOnEDT;
private static JModalConfiguration configuration;
/**
* Constructor for the default configuration object.
*/
private JModalConfiguration()
{
blurStyle = JBusyPanel.BLUR_STYLE_ALPHA_CHANNEL;
blurColor = null;
busyCursor = null;
busyCursorEnabled = true;
optionalFrameIcon = null;
optionalIFrameIcon = null;
windowDragEnabled = true;
windowDragBorderDistance = JModalWindow.DEFAULT_DRAG_BORDER_DISTANCE;
windowDragScreenSafetyMargin = Utils.DEFAULT_SCREEN_SAFETY_MARGIN;
alsoKeepModalToWindowInFront = false;
allowIconifyForBlockedInternalFrame = true;
enableWaitOnEDT = false;
}
/**
* Returns the configuration object.
*
* @return JModalConfiguration
*/
private static JModalConfiguration getInstance()
{
if (configuration == null)
{
configuration = new JModalConfiguration();
}
return configuration;
}
/**
* Retrieve the current setting for blurring.
*
* @return int Current blurring style.
*/
public synchronized static int getBlurStyle()
{
return getInstance().blurStyle;
}
/**
* Change the current setting for blurring.
*
* There are three options:
*
*
* - {@link JBusyPanel#BLUR_STYLE_ALPHA_CHANNEL BLUR_STYLE_ALPHA_CHANNEL}: Blur window
* by applying the {@link JBusyPanel#DEFAULT_ALPHA_TRANSPARENCY_FACTOR DEFAULT_ALPHA_TRANSPARENCY_FACTOR}
* to the window's background color [default].
*
- {@link JBusyPanel#BLUR_STYLE_NONE BLUR_STYLE_NONE}: Disabled blurring.
*
- Or set a fixed blur color (see {@link JModalConfiguration#setBlurColor(Color) setBlurColor} ).
*
*
* @param blurStyle int New blurring style.
*/
public synchronized static void setBlurStyle(int blurStyle)
{
getInstance().blurStyle = blurStyle;
}
/**
* Change the current setting for blurring to off.
*/
public synchronized static void setBlurStyleNone()
{
setBlurStyle(JBusyPanel.BLUR_STYLE_NONE);
}
/**
* Change the current setting for blurring to alpha channel.
*/
public synchronized static void setBlurStyleAlphaChannel()
{
setBlurStyle(JBusyPanel.BLUR_STYLE_ALPHA_CHANNEL);
}
/**
* Retrieve the current color for blurring.
*
* @return int Current blurring color.
*/
public synchronized static Color getBlurColor()
{
return getInstance().blurColor;
}
/**
* Determine the color used for blurring.
* If the no blurColor is set (see {@link JModalConfiguration#setBlurColor(Color) setBlurColor})
* then the {@link JBusyPanel#DEFAULT_ALPHA_TRANSPARENCY_FACTOR DEFAULT_ALPHA_TRANSPARENCY_FACTOR}
* is applied to the supplied backgroundColor.
*
* @param backgroundColor Color Optional base color for blurring.
* @return int Current blurring color.
*/
public static Color getBlurColor(Color backgroundColor)
{
if (getBlurColor() == null)
{
return new Color(backgroundColor.getRed(), backgroundColor.getGreen(), backgroundColor.getBlue(), JBusyPanel.DEFAULT_ALPHA_TRANSPARENCY_FACTOR);
}
else
{
return getBlurColor();
}
}
/**
* Set a fixed blurring color. If the blurColor has
* no alpha transparency factor (see {@link JBusyPanel#DEFAULT_ALPHA_NO_TRANSPARENCY_FACTOR DEFAULT_ALPHA_NO_TRANSPARENCY_FACTOR} )
* the {@link JBusyPanel#DEFAULT_ALPHA_TRANSPARENCY_FACTOR DEFAULT_ALPHA_TRANSPARENCY_FACTOR}
* is applied.
*
* If the blurColor is NULL the {@link JBusyPanel#DEFAULT_ALPHA_TRANSPARENCY_FACTOR DEFAULT_ALPHA_TRANSPARENCY_FACTOR}
* is applied to the window's background color in {@link JBusyPanel#BLUR_STYLE_ALPHA_CHANNEL BLUR_STYLE_ALPHA_CHANNEL} mode.
*
* @param blurColor Color New blurring color.
*/
public synchronized static void setBlurColor(Color blurColor)
{
if ((blurColor == null) || (blurColor.getAlpha() != JBusyPanel.DEFAULT_ALPHA_NO_TRANSPARENCY_FACTOR))
{
getInstance().blurColor = blurColor;
}
else
{
getInstance().blurColor = new Color(blurColor.getRed(), blurColor.getGreen(), blurColor.getBlue(), JBusyPanel.DEFAULT_ALPHA_TRANSPARENCY_FACTOR);
}
}
/**
* Retrieve the current busy cursor for a blocked modal component.
*
* The following 3 steps are used to determine the current busy cursor:
*
*
* - The busy cursor set with {@link #setBusyCursor(java.awt.Cursor) setBusyCursor} if it is not null.
*
- The default busy icon filed under the key "swingx.busy.cursor" in the UIManager.
*
- The default one which is a STOP sign.
*
*
* @return Current busy cursor.
*/
public synchronized static Cursor getBusyCursor()
{
if (getInstance().busyCursor == null)
{
return Utils.getBusyCursor();
}
else
{
return getInstance().busyCursor;
}
}
/**
* Change the current busy cursor for a blocked modal component.
*
* If the new value is null
the busy cursor is retrieved from {@link Utils#getBusyCursor() getBusyCursor} in Utils.
*
* @param busyCursor Cursor New busy cursor.
*/
public synchronized static void setBusyCursor(Cursor busyCursor)
{
getInstance().busyCursor = busyCursor;
}
/**
* Should the busy cursor be displayed for a blocked window.
*
* @return boolean Indication if the busy cursor should be used.
*/
public static boolean isBusyCursorEnabled()
{
return getInstance().busyCursorEnabled;
}
/**
* Enable the use of the busy cursor for a blocked window.
*/
public synchronized static void enableBusyCursor()
{
getInstance().busyCursorEnabled = true;
}
/**
* Disable the use of the busy cursor for a blocked window.
*/
public synchronized static void disableBusyCursor()
{
getInstance().busyCursorEnabled = false;
}
/**
* Retrieve the optional frame icon.
*
*
* The following 2 steps are used to determine the optional frame icon:
*
*
* - The frame icon set with {@link #setOptionalFrameIcon(java.awt.Image) setOptionalFrameIcon} if it is not null.
*
- The optional frame icon filed under the key "swingx.frame.icon" in the UIManager.
*
*
* @return Image An optional frame icon.
*/
public synchronized static Image getOptionalFrameIcon()
{
Image optionalFrameIcon = getInstance().optionalFrameIcon;
if (optionalFrameIcon != null)
{
return optionalFrameIcon;
}
String uiOptionalFrameIcon = UIManager.getString("swingx.frame.icon");
if (uiOptionalFrameIcon != null)
{
return Utils.getIcon(uiOptionalFrameIcon).getImage();
}
return null;
}
/**
* Change the optional frame icon.
*
* If the new value is null
the UIManager is used to check for an optional "swingx.frame.icon".
*
* @param optionalFrameIcon Image New optional frame icon.
*/
public synchronized static void setOptionalFrameIcon(Image optionalFrameIcon)
{
getInstance().optionalFrameIcon = optionalFrameIcon;
}
/**
* Retrieve the optional internal frame icon.
*
*
* The following 2 steps are used to determine the optional internal frame icon:
*
*
* - The internal frame icon set with {@link #setOptionalIFrameIcon(javax.swing.Icon) setOptionalIFrameIcon} if it is not null.
*
- The optional internal frame icon filed under the key "swingx.iframe.icon" in the UIManager.
*
*
* @return Image An optional internal frame icon.
*/
public synchronized static Icon getOptionalIFrameIcon()
{
Icon optionalIFrameIcon = getInstance().optionalIFrameIcon;
if (optionalIFrameIcon != null)
{
return optionalIFrameIcon;
}
String uiOptionalIFrameIcon = UIManager.getString("swingx.iframe.icon");
if (uiOptionalIFrameIcon != null)
{
return Utils.getIcon(uiOptionalIFrameIcon);
}
return null;
}
/**
* Change the optional internal frame icon.
*
* If the new value is null
the UIManager is used to check for an optional "swingx.iframe.icon".
*
* @param optionalIFrameIcon Image New optional internal frame icon.
*/
public synchronized static void setOptionalIFrameIcon(Icon optionalIFrameIcon)
{
getInstance().optionalIFrameIcon = optionalIFrameIcon;
}
/**
* Is it allowed to drag windows.
*
* @return boolean Indication if window dragging is allowed.
*/
public static boolean isWindowDraggingEnabled()
{
return getInstance().windowDragEnabled;
}
/**
* Enable the dragging of windows.
*/
public synchronized static void enableWindowDragging()
{
getInstance().windowDragEnabled = true;
}
/**
* Disable the dragging of windows.
*/
public synchronized static void disableWindowDragging()
{
getInstance().windowDragEnabled = false;
}
/**
* Retrieve the current drag border distance from border which activates a window drag cursor.
*
* @return int Current drag border distance.
*/
public synchronized static int getWindowDragBorderDistance()
{
return getInstance().windowDragBorderDistance;
}
/**
* Change the current drag border distance from border which activates a window drag cursor.
*
* The default distance is set to {@link JModalWindow#DEFAULT_DRAG_BORDER_DISTANCE DEFAULT_DRAG_BORDER_DISTANCE}.
*
* @param windowDragBorderDistance int New drag border distance for a window drag cursor.
*/
public synchronized static void setWindowDragBorderDistance(int windowDragBorderDistance)
{
getInstance().windowDragBorderDistance = windowDragBorderDistance;
}
/**
*
Retrieve the current window drag screen safety margin for window positioning
* in case of operating system toolbars and so on.
*
* @return int Current drag screen safety margin.
*/
public synchronized static int getWindowDragScreenSafetyMargin()
{
return getInstance().windowDragScreenSafetyMargin;
}
/**
* Retrieve the current window drag screen safety margin for window positioning
* in case of operating system toolbars and so on.
*
* @param windowDragScreenSafetyMargin int New window drag screen safety margin.
*/
public synchronized static void setWindowDragScreenSafetyMargin(int windowDragScreenSafetyMargin)
{
getInstance().windowDragScreenSafetyMargin = windowDragScreenSafetyMargin;
}
/**
* Should the modalToWindow also stay in the front when its blocking window is reactivated
* as a result of clicking in a blocked window.
*
* @return boolean Indication if the modalToWindow should also stay in the front.
*/
public static boolean keepModalToWindowInFront()
{
return getInstance().alsoKeepModalToWindowInFront;
}
/**
* Enable the placing of the modalToWindow in the front when its blocking window is reactivated
* as a result of clicking in a blocked window.
*/
public synchronized static void enableKeepModalToWindowInFront()
{
getInstance().alsoKeepModalToWindowInFront = true;
}
/**
* Disable the placing of the modalToWindow in the front when its blocking window is reactivated
* as a result of clicking in a blocked window.
*/
public synchronized static void disableKeepModalToWindowInFront()
{
getInstance().alsoKeepModalToWindowInFront = false;
}
/**
* May a blocked internal frame iconify when minimize is selected.
*
* @return boolean Indication if a blocked internal frame may iconify when minimize is selected.
*/
public static boolean allowIconifyForBlockedInternalFrame()
{
return getInstance().allowIconifyForBlockedInternalFrame;
}
/**
* Do not allow a blocked internal frame to iconify when minimize is selected.
*/
public synchronized static void disableIconifyForBlockedInternalFrame()
{
getInstance().allowIconifyForBlockedInternalFrame = false;
}
/**
* Allow a blocked internal frame to iconify when minimize is selected.
*/
public synchronized static void enableIconifyForBlockedInternalFrame()
{
getInstance().allowIconifyForBlockedInternalFrame = true;
}
/**
* Simulate JDialog like halting of the event dispatcher thread.
*
* @return boolean
*/
public static boolean simulateWaitOnEDT()
{
return getInstance().enableWaitOnEDT;
}
/**
* Enable simulating JDialog like halting of the event dispatcher thread.
*
* @throws SecurityException
* if a security manager exists and its {@link
* java.lang.SecurityManager#checkAwtEventQueueAccess}
method denies
* access to the EventQueue.
* @see java.awt.AWTPermission
*/
public synchronized static void enableWaitOnEDT()
{
enableWaitOnEDT(new JModalEventQueue());
}
/**
* Enable simulating JDialog like halting of the event dispatcher thread.
* And in case you need your own custom EventQueue supply one based on the {@link JModalEventQueue JModalEventQueue}
* that is need to enable this feature.
*
* @param customEventQueue JModalEventQueue
* @throws SecurityException
* if a security manager exists and its {@link
* java.lang.SecurityManager#checkAwtEventQueueAccess}
method denies
* access to the EventQueue.
* @see java.awt.AWTPermission
*/
public synchronized static void enableWaitOnEDT(JModalEventQueue customEventQueue)
{
EventQueue currentQueue = Toolkit.getDefaultToolkit().getSystemEventQueue();
if (!(currentQueue instanceof JModalEventQueue))
{
Toolkit.getDefaultToolkit().getSystemEventQueue().push(customEventQueue);
}
getInstance().enableWaitOnEDT = true;
}
/**
* Disable simulating JDialog like halting of the event dispatcher thread.
*/
public synchronized static void disableWaitOnEDT()
{
getInstance().enableWaitOnEDT = false;
}
}