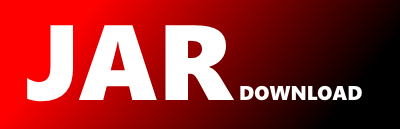
nl.esi.metis.aisparser.AISMessage05 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of EsiAisParser Show documentation
Show all versions of EsiAisParser Show documentation
This package supports the parsing of AIS messages in Java. AIS, the Automatic Identification System, is a system aiming at improving maritime safety by exchanging messages between ships, other vehicles in particular aircraft involved in search-and-rescue (SAR), and (fixed) base stations. To be precise, this package support the ITU-R M.1371-4 AIS standard.
See our extensive javadoc and in particular the class AISParser for more information on how to use this package.
The parser was used in the Poseidon project, and is improved in the Metis project to better handle uncertain information. Both projects were led by the Embedded Systems Institute. In both projects Thales Nederlands was the carrying industrial partner, and multiple Dutch universities participated.
The newest version!
package nl.esi.metis.aisparser;
import cern.colt.bitvector.BitVector;
/** This interface represents an AIS message of type 5: Ship static and voyage related data.
* Such messages are used by Class A shipborne and SAR aircraft AIS stations to report static and voyage-related data.
* @author Pierre van de Laar
* @author Pierre America
*/
public interface AISMessage05 extends AISMessage {
/** Returns an indicator for the AIS version applicable to the current message.
* @return an integer value indicating the AIS version:
* 0 = station compliant with Recommendation ITU-R M.1371-1
* 1 = station compliant with Recommendation ITU-R M.1371-3
* 2-3 = station compliant with future editions
*/
public int getAisVersionIndicator();
/** Returns the IMO number of the transmitting ship.
* See Wikipedia for more information on IMO (International Maritime Organization) numbers.
* @return an integer value representing the IMO number (1-999999999)
* 0 = not available = default
* Not applicable to SAR aircraft
*/
public int getImoNumber();
/** Returns the call sign of the transmitting ship.
* @return a String value, containing at most 7 characters, representing the call sign
* "" = not available
*/
public String getCallSign();
/** Returns the name of the transmitting ship.
* @return a String value, containing maximum 20 characters, representing the name.
* "" = not available.
* For SAR aircraft, it should be set to "SAR AIRCRAFT NNNNNNN" where NNNNNNN equals the aircraft registration number
*/
public String getName();
/** Returns the type of ship and cargo type. This can be converted to a String by utility class {@link UtilsShipType8}.
* @return an integer value representing the type of ship and cargo type:
* 0 = not available or no ship
* 1-99 = as defined in the standard (see {@link UtilsShipType8})
* 100-199 = reserved for regional use
* 200-255 = reserved for future use
* Not applicable to SAR aircraft
*/
public int getTypeOfShipAndCargoType();
/** Returns the dimensions of the ship and the reference point for the reported position.
* These can be analyzed further with utility class {@link UtilsDimensions30}.
* @return a BitVector
value representing the dimensions.
*/
public BitVector getDimension();
/** Returns the type of electronic position fixing device.
* @return an integer value representing of the type of electronic position fixing device:
* 0 = undefined (default)
* 1 = global positioning system (GPS)
* 2 = GNSS (GLONASS)
* 3 = combined GPS/GLONASS
* 4 = Loran-C
* 5 = Chayka
* 6 = integrated navigation system
* 7 = surveyed
* 8 = Galileo
* 9-14 = not used
* 15 = internal GNSS
*/
public int getTypeOfElectronicPositionFixingDevice();
/** Returns the estimated time of arrival (ETA).
* This can be analyzed further using utility class {@link UtilsEta}.
* @return a BitVector
value of the estimated time of arrival (MMDDHHMM UTC)
* Bits 19-16: month; 1-12; 0 = not available
* Bits 15-11: day; 1-31; 0 = not available
* Bits 10-6: hour; 0-23; 24 = not available
* Bits 5-0: minute; 0-59; 60 = not available
* For SAR aircraft, the use of this field may be decided by the responsible administration.
*/
public BitVector getEta();
/** Returns the maximum present static draught.
* @return an integer value expressing the maximum present static draught in 1/10 m
* 255 = draught 25.5 m or greater
* 0 = not available
* in accordance with IMO Resolution A.851
* Not applicable to SAR aircraft, should be set to 0
*/
public int getMaximumPresentStaticDraught();
/** Returns the destination.
* @return a String value, containing maximum 20 characters, representing the destination
* "" = not available
* For SAR aircraft, the use of this field may be decided by the responsible administration.
*/
public String getDestination();
/** Returns the data terminal equipment (DTE) status.
* @return a boolean value describing whether the data terminal equipment is ready:
* false = available
* true = not available
*/
public boolean getDte();
/** Get the spare bit.
* @return the integer value of the spare bit. This should be zero.
*/
public int getSpare();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy