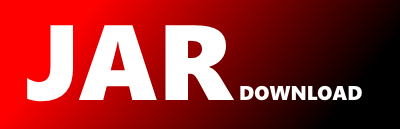
nl.esi.metis.aisparser.AISMessage21 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of EsiAisParser Show documentation
Show all versions of EsiAisParser Show documentation
This package supports the parsing of AIS messages in Java. AIS, the Automatic Identification System, is a system aiming at improving maritime safety by exchanging messages between ships, other vehicles in particular aircraft involved in search-and-rescue (SAR), and (fixed) base stations. To be precise, this package support the ITU-R M.1371-4 AIS standard.
See our extensive javadoc and in particular the class AISParser for more information on how to use this package.
The parser was used in the Poseidon project, and is improved in the Metis project to better handle uncertain information. Both projects were led by the Embedded Systems Institute. In both projects Thales Nederlands was the carrying industrial partner, and multiple Dutch universities participated.
The newest version!
/* ESI AIS Parser
*
* Copyright 2011/2012 by Pierre van de Laar & Pierre America (Embedded Systems Institute)
* Copyright 2008 by Brian C. Lane
* All Rights Reserved
*
*/
package nl.esi.metis.aisparser;
import cern.colt.bitvector.BitVector;
/** This interface represents an AIS message of type 21: Aids-to-Navigation Report (AtoN).
*
* @author Pierre van de Laar
* @author Pierre America
* @author Brian C. Lane
*/
public interface AISMessage21 extends AISMessage, PositionInfo {
/** Returns the type of Aid-to-Navigation.
* @return an integer value representing the type of AtoN:
* 0 = not available
* Refer to the definition in Table 71 of the standard.
*/
public int getTypeOfAtoN();
/** Returns the name of the Aid-to-Navigation.
* The name of the AtoN may be extended by the parameter {@linkplain #getNameOfAtoNExtension() "Name of Aid-to-Navigation Extension"} below.
* @return a String value, containing maximum 20 characters, representing the name of the AtoN.
* "" = not available
*/
public String getNameOfAtoN();
/** Returns the dimensions of the Aid-to-Navigation and the reference point for the reported position.
* These can be analyzed further with utility class {@link UtilsDimensions30}.
* Note that for fixed Aids-to-Navigation, virtual AtoN, and for off-shore structures,
* the orientation established by the bow-to-stern dimension is supposed to point to true north.
* For floating aids, the dimensions are supposed to be approximated by a circle, with the reference point in the middle.
* @return a BitVector
value representing the dimensions.
*/
public BitVector getDimension();
/** Returns the type of electronic position fixing device.
* @return an integer value representing of the type of electronic position fixing device:
* 0 = undefined (default)
* 1 = global positioning system (GPS)
* 2 = GNSS (GLONASS)
* 3 = combined GPS/GLONASS
* 4 = Loran-C
* 5 = Chayka
* 6 = integrated navigation system
* 7 = surveyed
* 8 = Galileo
* 9-14 = not used
* 15 = internal GNSS
*/
public int getTypeOfElectronicPositionFixingDevice();
/** Returns the time stamp contained in the message.
* This can be analyzed further using utility class {@link UtilsTimeStamp}.
* @return an integer value representing the UTC second when the report was generated by the electronic position fixing system (EPFS) (0-59)
* 60 if time stamp is not available
* 61 if positioning system is in manual input mode
* 62 if electronic position fixing system operates in estimated (dead reckoning) mode
* 63 if the positioning system is inoperative
*/
public int getTimeStamp();
/** Returns the off-position indicator.
* Note: This flag should only be considered valid by the receiving station
* if the AtoN is a floating aid ({@linkplain #getTypeOfAtoN AtoN type} ≥ 20) and {@linkplain #getTimeStamp time stamp} ≤ 59.
* @return a boolean value representing the off-position indicator:
* false = on position
* true = off position
*/
public boolean getOffPositionIndicator();
/** Returns the status of the AtoN.
* @return an integer value representing the AtoN status.
* This is reserved for the indication of the AtoN status and should be zero now.
*/
public int getStatusAtoN();
/** Returns the RAIM flag, which describes the receiver autonomous integrity monitoring status of the electronic position fixing device.
* @return a boolean value:
* false = RAIM not in use
* true = RAIM in use
*/
public boolean getRaimFlag();
/** Returns the virtual AtoN flag.
* @return a boolean value representing the virtual AtoN flag:
* false = real AtoN at indicated position
* true = virtual AtoN, does not physically exist
*/
public boolean getVirtualAtoNFlag();
/** Returns the assigned mode flag.
* @return a boolean value representing the assigned mode flag:
* false = Station operating in autonomous and continuous mode
* true = Station operating in assigned mode
*/
public boolean getAssignedModeFlag();
/** Returns the first spare bit.
* @return the value of the first spare bit. This should be zero.
*/
public int getSpare1();
/** Returns the name of AtoN extension.
* This can be combined with the {@linkplain #getNameOfAtoN() name of the Aid-to-Navigation} field.
* @return a String value of up to 14 characters, representing the name of AtoN extension
*/
public String getNameOfAtoNExtension();
/** Returns the second set of spare bits.
* @return the value of the second set of spare bits.
* This should be zero, although the number of bits can vary depending on the length of the name of AtoN extension.
*/
public int getSpare2();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy