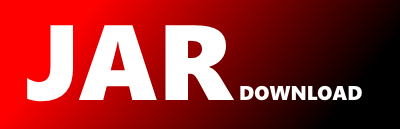
nl.esi.metis.aisparser.AISMessagePositionReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of EsiAisParser Show documentation
Show all versions of EsiAisParser Show documentation
This package supports the parsing of AIS messages in Java. AIS, the Automatic Identification System, is a system aiming at improving maritime safety by exchanging messages between ships, other vehicles in particular aircraft involved in search-and-rescue (SAR), and (fixed) base stations. To be precise, this package support the ITU-R M.1371-4 AIS standard.
See our extensive javadoc and in particular the class AISParser for more information on how to use this package.
The parser was used in the Poseidon project, and is improved in the Metis project to better handle uncertain information. Both projects were led by the Embedded Systems Institute. In both projects Thales Nederlands was the carrying industrial partner, and multiple Dutch universities participated.
The newest version!
/* ESI AIS Parser
*
* Copyright 2011/2012 by Pierre van de Laar & Pierre America (Embedded Systems Institute)
* Copyright 2008 by Brian C. Lane
* All Rights Reserved
*
*/
package nl.esi.metis.aisparser;
/** This is the common interface for all types of AIS messages that provide position reports.
* Mobile stations (i.e., ships and other vehicles) should periodically transmit such position reports.
* @author Pierre van de Laar
* @author Pierre America
*/
public interface AISMessagePositionReport extends AISMessage, PositionInfo {
/** Returns the navigational status.
* This can be further analyzed using utility class {@link UtilsNavStatus}.
* @return an integer in the range of 0 to 15:
* 0 = under way using engine
* 1 = at anchor
* 2 = not under command
* 3 = restricted maneuverability
* 4 = constrained by her draught
* 5 = moored
* 6 = aground
* 7 = engaged in fishing
* 8 = under way sailing
* 9 = reserved for future amendment of navigational status for ships carrying DG, HS, or MP, or IMO hazard or pollutant category C, high speed craft (HSC)
* 10 = reserved for future amendment of navigational status for ships carrying dangerous goods (DG), harmful substances (HS) or marine pollutants (MP),
* or IMO hazard or pollutant category A, wing in grand (WIG)
* 11-13 = reserved for future use
* 14 = AIS-SART (active)
* 15 = not defined = default (also used by AIS-SART under test)
*/
public int getNavigationalStatus();
/** Returns the rate of turn.
* This value can be analyzed further with utility class {@link UtilsRateOfTurn8}.
* @return an integer value in the range of -128 to 127:
* 0 to +126 = turning right at up to 708° per minute or higher
* 0 to -126 = turning left at up to 708° per minute or higher
* Values between 0 and 708° per minute coded by ROTAIS = 4.733 SQRT(ROTsensor) degrees per minute,
* where ROTsensor is the Rate of Turn as input by an external Rate of Turn Indicator (TI).
* ROTAIS is rounded to the nearest integer value.
* +127 = turning right at more than 5° per 30 seconds (No TI available)
* -127 = turning left at more than 5° per 30 seconds (No TI available)
* -128 (80 hex) indicates no turn information available (default).
*/
public int getRateOfTurn();
/** Returns the speed over ground.
* @return an integer value in the range of 0 to 1023,
* representing the speed over ground in 1/10 knot steps (0-102.2 knots)
* 1023 = not available
* 1022 = 102.2 knots or higher
*/
public int getSpeedOverGround();
/** Returns the course over ground.
* This value can be analyzed further with utility class {@link UtilsAngle12}.
* @return an integer value representing the course over ground in 1/10° for values in the range of 0 to 3599.
* 3600 (E10h) = not available.
* 3601 or higher should not be used
*/
public int getCourseOverGround();
/** Returns the true heading.
* @return an integer value representing the true heading in degrees (0-359).
* 511 indicates not available
*/
public int getTrueHeading();
/** Returns the time stamp contained in the message.
* This can be analyzed further using utility class {@link UtilsTimeStamp}.
* @return an integer value representing the UTC second when the report was generated by the electronic position fixing system (EPFS) (0-59)
* 60 if time stamp is not available
* 61 if positioning system is in manual input mode
* 62 if electronic position fixing system operates in estimated (dead reckoning) mode
* 63 if the positioning system is inoperative
*/
public int getTimeStamp();
/** Returns the special maneuver indicator.
* @return an integer value with the following meaning:
* 0 = not available
* 1 = not engaged in special maneuver
* 2 = engaged in special maneuver (i.e.: regional passing arrangement on Inland Waterway)
*/
public int getSpecialManoeuvre();
/** Returns the spare bits.
* @return the integer value of the spare bits, which should be zero.
*/
public int getSpare();
/** Returns the RAIM flag, which describes the receiver autonomous integrity monitoring status of the electronic position fixing device.
* @return a boolean value:
* false = RAIM not in use
* true = RAIM in use
*/
public boolean getRaimFlag();
/** Returns the communication state.
* @return the value of the communication state. Depending on the message type, this may be {@link Itdma} or {@link Sotdma}.
*/
public CommunicationState getCommunicationState();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy