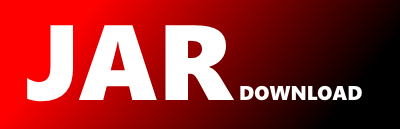
nl.hsac.fitnesse.fixture.slim.MockServerMessageReport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.fixture.slim;
import nl.hsac.fitnesse.fixture.util.XmlHttpResponse;
import org.apache.commons.lang3.StringUtils;
import java.util.Arrays;
import java.util.List;
/**
* Slim table fixture to show messages received (and sent) by MockXmlServer. This fixture does not alter test outcome
* it just shows which messages have (not) been received.
*/
public class MockServerMessageReport extends SlimFixture {
private final String path;
public MockServerMessageReport() {
this(MockXmlServerSetup.DEFAULT_PATH);
}
public MockServerMessageReport(String aPath) {
path = aPath;
}
public List doTable(List> table) {
List> result;
List extends XmlHttpResponse> responses;
try {
responses = MockXmlServerSetup.getResponses(path);
} catch (SlimFixtureException e) {
responses = null;
}
if (responses == null || responses.isEmpty()) {
result = createResult("ignore", "No requests expected or received at: " + path);
} else {
boolean allPass = true;
int failCounter = 0;
int counter = 1;
StringBuilder builder = new StringBuilder("");
for (XmlHttpResponse response : responses) {
boolean rowPass = addRowForResponse(counter, builder, response);
if (!rowPass) {
failCounter++;
}
allPass &= rowPass;
counter++;
}
builder.append("
");
int responseCount = responses.size();
String status;
String header;
if (allPass) {
status = "pass";
header = String.format("Expected and received %s requests", responseCount);
} else {
status = "fail";
header = String.format("%s (of %s) request(s) did not match expectation", failCounter, responseCount);
}
String resultCell = createScenario(!allPass, status, header, builder.toString());
result = createResult("report", resultCell);
}
return result;
}
protected boolean addRowForResponse(int counter, StringBuilder builder, XmlHttpResponse response) {
boolean result;
builder.append("");
if (requestPass(response)) {
result = true;
} else {
result = false;
}
addMessageNoCell(result, builder, counter);
addRequestCell(result, builder, response);
addResponseCell(result, builder, response);
builder.append(" ");
return result;
}
protected void addMessageNoCell(boolean result, StringBuilder builder, int counter) {
builder.append("");
builder.append(counter);
builder.append(" ");
}
protected boolean requestPass(XmlHttpResponse response) {
return StringUtils.isNotEmpty(response.getResponse())
&& StringUtils.isNotEmpty(response.getRequest());
}
protected void addRequestCell(boolean result, StringBuilder builder, XmlHttpResponse response) {
String requestBody = response.getRequest();
builder.append("");
if (requestBody == null) {
builder.append("No request received");
} else {
String reqTitle = getRequestTitle(response);
String req = formatBody(requestBody);
builder.append(createCollapsible(!result, reqTitle, req));
}
builder.append(" ");
}
protected void addResponseCell(boolean result, StringBuilder builder, XmlHttpResponse response) {
String responseBody = response.getResponse();
builder.append("");
if (responseBody == null || "".equals(responseBody)) {
builder.append("Unexpected request, no response set up");
} else {
String respTitle = getResponseTitle(response);
String resp = formatBody(responseBody);
builder.append(createCollapsible(false, respTitle, resp));
}
builder.append(" ");
}
protected String getRequestTitle(XmlHttpResponse response) {
return "request";
}
protected String getResponseTitle(XmlHttpResponse response) {
return "response";
}
protected String formatBody(String xml) {
try {
return getEnvironment().getHtmlForXml(xml);
} catch (Exception e) {
return xml;
}
}
protected List> createResult(String status, String result) {
return Arrays.asList(Arrays.asList(status + ":" + result));
}
protected String createScenario(String status, String header, String detail) {
return createScenario("fail".equals(status), status, header, detail);
}
protected String createScenario(boolean open, String status, String header, String detail) {
String scenarioClosed = " closed";
String detailClosed = " closed-detail";
if (open) {
scenarioClosed = "";
detailClosed = "";
}
return String.format(""
+"%s "
+"%s "
+"
",
scenarioClosed, status, header, detailClosed, detail);
}
protected String createCollapsible(boolean open, String title, String body) {
String closed = " closed";
if (open) {
closed = "";
}
return String.format(""
+"%s
"
+"%s"
+"",
closed, title, body);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy