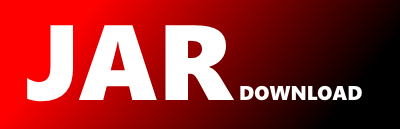
nl.hsac.fitnesse.fixture.util.MapHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.fixture.util;
import nl.hsac.fitnesse.fixture.slim.ListFixture;
import nl.hsac.fitnesse.fixture.slim.SlimFixtureException;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class MapHelper {
private static final Pattern LIST_INDEX_PATTERN = Pattern.compile("(\\S+)\\[(\\d+)\\]");
private HtmlCleaner htmlCleaner = new HtmlCleaner();
/**
* Gets value from map.
* @param map map to get value from.
* @param name name of (possibly nested) property to get value from.
* @return value found, if it could be found, null otherwise.
*/
public Object getValue(Map map, String name) {
String cleanName = htmlCleaner.cleanupValue(name);
return getValueImpl(map, cleanName);
}
protected Object getValueImpl(Map map, String name) {
Object value = null;
if (map.containsKey(name)) {
value = map.get(name);
} else {
String[] parts = name.split("\\.", 2);
if (parts.length > 1) {
Object nested = getValueImpl(map, parts[0]);
if (nested instanceof Map) {
Map nestedMap = (Map) nested;
value = getValueImpl(nestedMap, parts[1]);
}
} else if (isListName(name)) {
value = getListValue(map, name);
} else if (isListIndexExpr(name)) {
value = getIndexedListValue(map, name);
}
}
return value;
}
/**
* Stores value in map.
* @param value value to be passed.
* @param name name to use this value for.
* @param map map to store value in.
*/
public void setValueForIn(Object value, String name, Map map) {
if (isListName(name)) {
String valueStr = null;
if (value != null) {
valueStr = value.toString();
}
setValuesForIn(valueStr, stripListIndicator(name), map);
} else {
if (name.endsWith("\\[]")) {
name = name.replace("\\[]", "[]");
}
String cleanName = htmlCleaner.cleanupValue(name);
Object cleanValue = value;
if (value instanceof String) {
cleanValue = htmlCleaner.cleanupValue((String) value);
}
if (map.containsKey(cleanName)) {
// overwrite current value
map.put(cleanName, cleanValue);
} else {
int lastDot = cleanName.lastIndexOf(".");
if (lastDot > -1) {
String key = cleanName.substring(0, lastDot);
Object nested = getValue(map, key);
if (nested instanceof Map) {
Map nestedMap = (Map) nested;
String lastPart = cleanName.substring(lastDot + 1);
setValueForIn(cleanValue, lastPart, nestedMap);
} else {
throw new SlimFixtureException(false, key + " is not a map, but " + nested.getClass());
}
} else if (isListIndexExpr(name)) {
setIndexedListValue(map, cleanName, cleanValue);
} else {
map.put(cleanName, cleanValue);
}
}
}
}
/**
* Stores list of values in map.
* @param values comma separated list of values.
* @param name name to use this list for.
* @param map map to store values in.
*/
public void setValuesForIn(String values, String name, Map map) {
String cleanName = htmlCleaner.cleanupValue(name);
String[] valueArrays = values.split("\\s*,\\s*");
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy