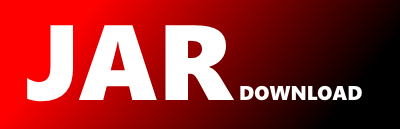
nl.hsac.fitnesse.fixture.slim.JsonHttpTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.fixture.slim;
import nl.hsac.fitnesse.fixture.util.JsonPathHelper;
import org.json.JSONObject;
import java.util.List;
/**
* Fixture to make Http calls and interpret the result as JSON.
*/
public class JsonHttpTest extends HttpTest {
private final JsonPathHelper pathHelper = new JsonPathHelper();
public boolean postValuesAsJsonTo(String serviceUrl) {
return postToImpl(jsonEncodeCurrentValues(), serviceUrl);
}
public boolean putValuesAsJsonTo(String serviceUrl) {
return putToImpl(jsonEncodeCurrentValues(), serviceUrl);
}
protected String jsonEncodeCurrentValues() {
return new JSONObject(getCurrentValues()).toString();
}
@Override
protected String formatValue(String value) {
return getEnvironment().getHtmlForJson(value);
}
public Object jsonPath(String path) {
String jsonPath = getPathExpr(path);
return pathHelper.getJsonPath(getResponse().getResponse(), jsonPath);
}
public int jsonPathCount(String path) {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy