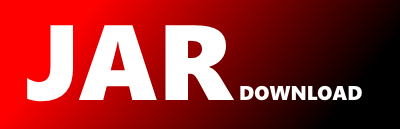
nl.hsac.fitnesse.fixture.util.FirstNonNullHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.fixture.util;
import java.util.Collection;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Stream;
/**
* Helper to find first non-null elements using suppliers or functions, limiting the number of executions.
*/
public class FirstNonNullHelper {
/**
* Gets first supplier's result which is not null.
* Suppliers are called sequentially. Once a non-null result is obtained the remaining suppliers are not called.
*
* @param type of result returned by suppliers.
* @param suppliers all possible suppliers that might be able to supply a value.
* @return first result obtained which was not null, OR null
if all suppliers returned null
.
*/
@SafeVarargs
public static T firstNonNull(Supplier... suppliers) {
return firstNonNull(Supplier::get, suppliers);
}
/**
* Gets first result of function which is not null.
*
* @param type of values.
* @param element to return.
* @param function function to apply to each value.
* @param values all possible values to apply function to.
* @return first result which was not null,
* OR null
if either result for all values was null
or values was null
.
*/
public static R firstNonNull(Function function, T[] values) {
return values == null ? null : firstNonNull(function, Stream.of(values));
}
/**
* Gets first result of function which is not null.
*
* @param type of values.
* @param element to return.
* @param function function to apply to each value.
* @param values all possible values to apply function to.
* @return first result which was not null,
* OR null
if either result for all values was null
or values was null
.
*/
public static R firstNonNull(Function function, Collection values) {
return values == null ? null : firstNonNull(function, values.stream());
}
/**
* Gets first result of function which is not null.
*
* @param type of values.
* @param element to return.
* @param function function to apply to each value.
* @param suppliers all possible suppliers that might be able to supply a value.
* @return first result which was not null,
* OR null
if result for all supplier's values was null
.
*/
public static R firstNonNull(Function function, Supplier>... suppliers) {
Stream> resultStream = Stream.of(suppliers)
.map(s -> (() -> firstNonNull(function, s.get())));
return firstNonNull(Supplier::get, resultStream);
}
/**
* Gets first result of set of function which is not null.
*
* @param type of values.
* @param element to return.
* @param input input to provide to all functions.
* @param function first function to apply (separate to indicate at least one should be provided)
* @param functions all possible functions that might be able to supply a value.
* @return first result which was not null,
* OR null
if result for each function's results was null
.
*/
public static R firstNonNull(T input, Function function, Function... functions) {
return firstNonNull(f -> f.apply(input), Stream.concat(Stream.of(function), Stream.of(functions)));
}
/**
* Gets first element which is not null.
*
* @param type of values.
* @param element to return.
* @param function function to apply to each value.
* @param values all possible values.
* @return first result value which was not null, OR null
if all values were null
.
*/
public static R firstNonNull(Function function, Stream values) {
return values
.map(function)
.filter(Objects::nonNull)
.findFirst()
.orElse(null);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy