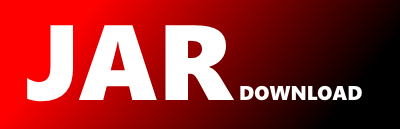
nl.hsac.fitnesse.fixture.util.HttpResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.fixture.util;
import org.apache.http.client.CookieStore;
import org.apache.http.cookie.Cookie;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* Wrapper around HTTP response (and request).
*/
public class HttpResponse {
private final static Map INSTANCES = new ConcurrentHashMap<>();
private Map requestHeaders = new LinkedHashMap<>();
private Map responseHeaders = new LinkedHashMap<>();
private String method;
private String request;
protected String response;
private int statusCode;
private CookieStore cookieStore;
private long responseTime = -1;
/**
* @throws RuntimeException if no valid response is available
*/
public void validResponse() {
if (statusCode == 0) {
throw new NonValidResponseReceivedException("Status code is 0. Probably no response was received.");
}
if (statusCode < 100) {
throw new NonValidResponseReceivedException("Status code is less than 100: " + statusCode);
}
if (statusCode >= 400 && statusCode <= 499) {
throw new NonValidResponseReceivedException("Server reported client error: " + statusCode);
}
if (statusCode >= 500 && statusCode <= 599) {
throw new NonValidResponseReceivedException("Server error returned: " + statusCode);
}
}
/**
* @return the request
*/
public String getRequest() {
return request;
}
/**
* @param aRequest the request to set
*/
public void setRequest(String aRequest) {
request = aRequest;
}
/**
* @return the response
*/
public String getResponse() {
return response;
}
/**
* @param aResponse the response to set
*/
public void setResponse(String aResponse) {
response = aResponse;
}
/**
* @return the statusCode
*/
public int getStatusCode() {
return statusCode;
}
/**
* @param aStatusCode the statusCode to set
*/
public void setStatusCode(int aStatusCode) {
statusCode = aStatusCode;
}
/**
* @return actual headers sent (these will contain the requested headers and some implicit).
*/
public Map getRequestHeaders() {
return requestHeaders;
}
/**
* Adds value to actual headers sent (there may be multiple values per name).
* @param headerName name of header to add.
* @param headerValue value to add.
* @return all current values for name.
*/
public Object addRequestHeader(String headerName, String headerValue) {
return addValue(getRequestHeaders(), headerName, headerValue);
}
/**
* @return headers in response.
*/
public Map getResponseHeaders() {
return responseHeaders;
}
/**
* Adds value to headers received (there may be multiple values per name).
* @param headerName name of header to add.
* @param headerValue value to add.
* @return all current values for name.
*/
public Object addResponseHeader(String headerName, String headerValue) {
return addValue(getResponseHeaders(), headerName, headerValue);
}
protected Object addValue(Map headers, String name, String value) {
Object result;
Object existingValue = headers.get(name);
if (existingValue == null) {
headers.put(name, value);
result = value;
} else if (existingValue instanceof Collection) {
((Collection) existingValue).add(value);
result = existingValue;
} else {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy