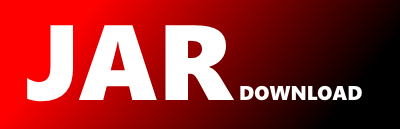
nl.hsac.fitnesse.fixture.util.JsonHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.fixture.util;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import net.minidev.json.JSONArray;
import nl.hsac.fitnesse.fixture.Environment;
import org.apache.commons.lang3.StringUtils;
import org.json.JSONException;
import org.json.JSONObject;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* Helper dealing with JSON objects.
*/
public class JsonHelper {
private final static Gson GSON = new Gson();
private final static Type MAP_TYPE = new TypeToken>(){}.getType();
/**
* Interprets supplied String as Json and converts it into a Map.
* @param jsonString string to interpret as Json object.
* @return property -> value.
*/
public Map jsonStringToMap(String jsonString) {
if (StringUtils.isEmpty(jsonString)) {
return null;
}
try {
return GSON.fromJson(jsonString, MAP_TYPE);
} catch (JSONException e) {
throw new RuntimeException("Unable to convert string to map: " + jsonString, e);
}
}
private Map jsonObjectToMap(JSONObject jsonObject) throws JSONException {
Map result = new LinkedHashMap<>();
for (Object key : jsonObject.keySet()) {
String stringKey = String.valueOf(key);
Object value = convertJsonObject(jsonObject.get(stringKey));
result.put(stringKey, value);
}
return result;
}
private Object convertJsonObject(Object value) {
Object result = value;
if (value instanceof JSONObject) {
result = jsonObjectToMap((JSONObject) value);
} else if (value instanceof org.json.JSONArray) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy