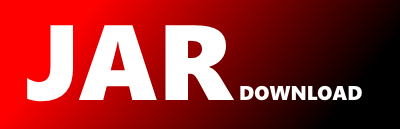
nl.hsac.fitnesse.fixture.util.selenium.by.TechnicalSelectorBy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.fixture.util.selenium.by;
import org.apache.commons.lang3.StringUtils;
import org.openqa.selenium.By;
import java.util.function.Function;
import static nl.hsac.fitnesse.fixture.util.FirstNonNullHelper.firstNonNull;
/**
* By to work using 'technical selectors.
*/
public class TechnicalSelectorBy {
private static final Function ID_BY = byIfStartsWith("id", By::id);
private static final Function CSS_BY = byIfStartsWith("css", By::cssSelector);
private static final Function NAME_BY = byIfStartsWith("name", By::name);
private static final Function LINKTEXT_BY = byIfStartsWith("link", By::linkText);
private static final Function PARTIALLINKTEXT_BY = byIfStartsWith("partialLink", By::partialLinkText);
private static final Function XPATH_BY = byIfStartsWith("xpath", XPathBy::new);
/**
* Whether supplied place is a technical selector.
* @param place place that might be technical selector
* @return true if place starts with one of the technical selector prefixes.
*/
public static boolean isTechnicalSelector(String place) {
return StringUtils.startsWithAny(place,
"id=", "xpath=", "css=", "name=", "link=", "partialLink=");
}
/**
* @param place place that might be technical selector
* @return By if place was a technical selector, null otherwise.
*/
public static By forPlace(String place) {
return firstNonNull(place,
ID_BY,
CSS_BY,
NAME_BY,
LINKTEXT_BY,
PARTIALLINKTEXT_BY,
XPATH_BY);
}
public static Function byIfStartsWith(String prefix, Function constr) {
String prefixEq = prefix + "=";
int prefixLength = prefixEq.length();
return place -> place.startsWith(prefixEq) ? constr.apply(place.substring(prefixLength)) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy