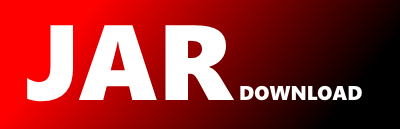
nl.hsac.fitnesse.junit.reportmerge.TestReportFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.junit.reportmerge;
import nl.hsac.fitnesse.fixture.util.FileUtil;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.nio.file.Path;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import static java.util.Collections.emptyMap;
import static nl.hsac.fitnesse.junit.reportmerge.TestReportHtml.ERROR_STATUS;
import static nl.hsac.fitnesse.junit.reportmerge.TestReportHtml.FAIL_STATUS;
import static nl.hsac.fitnesse.junit.reportmerge.TestReportHtml.IGNORE_STATUS;
import static nl.hsac.fitnesse.junit.reportmerge.TestReportHtml.NO_TEST_STATUS;
import static nl.hsac.fitnesse.junit.reportmerge.TestReportHtml.PASS_STATUS;
/**
* Factory to create TestReportHtml instances.
*/
public class TestReportFactory {
protected static final String OVERVIEW_TABLE_START = "Name Right Wrong Exceptions Runtime (in milliseconds) ";
protected static final Pattern TIME_PATTERN = Pattern.compile("(.+?) .+? .+? .+? (\\d+?) ");
protected final File rootDir;
protected final Map> testTimes = new HashMap<>();
protected final Map> testIndexes = new HashMap<>();
public TestReportFactory(File rootDir) {
this.rootDir = rootDir;
}
public TestReportHtml create(Path reportPath) {
File reportFile = reportPath.toFile();
String content = getFileContent(reportFile);
boolean isOverview = content.contains(OVERVIEW_TABLE_START);
String status = getStatus(content);
TestReportHtml reportHtml = new TestReportHtml(rootDir, reportPath, isOverview, status);
if (reportHtml.isOverviewPage()) {
Map runTimes = getRunTimes(reportHtml, content);
testTimes.put(reportHtml.getRunName(), runTimes);
computeRunIndexes(reportHtml.getRunName(), runTimes);
}
return reportHtml;
}
public long getTime(String run) {
return getTestTimes(run).values().stream().reduce(0L, Long::sum);
}
public long getTime(String run, String page) {
Long time = getTestTimes(run).get(page);
if (time == null) {
time = -1L;
}
return time;
}
protected Map getTestTimes(String run) {
return testTimes.computeIfAbsent(run, x -> emptyMap());
}
protected void computeRunIndexes(String run, Map runTimes) {
int index = 0;
Map runIndexes = getTestIndexes(run);
for (String page : runTimes.keySet()) {
runIndexes.put(page, index++);
}
}
public int getIndex(String run) {
return Integer.MAX_VALUE;
}
public int getIndex(String run, String page) {
return getTestIndexes(run)
.computeIfAbsent(page, p -> {
// absent means: single test report (i.e. no overview page present)
int offset = 2;
if (page.endsWith(".SuiteSetUp")) {
// single test -> setup before actual test
offset = 3;
} else if (page.endsWith(".SuiteTearDown")) {
// single test -> teardown after actual test
offset = 1;
}
return getIndex(run) - offset;
});
}
protected Map getTestIndexes(String run) {
return testIndexes.computeIfAbsent(run, x -> new HashMap<>());
}
protected String getStatus(String content) {
String result;
if (content.contains("class=\"error\">")) {
result = ERROR_STATUS;
} else if (content.contains("class=\"fail\">")) {
result = FAIL_STATUS;
} else if (content.contains("class=\"pass\">")) {
result = PASS_STATUS;
} else if (content.contains("class=\"ignore\">")) {
result = IGNORE_STATUS;
} else {
result = NO_TEST_STATUS;
}
return result;
}
protected Map getRunTimes(TestReportHtml reportHtml, String content) {
Map result = new LinkedHashMap<>();
Matcher m = TIME_PATTERN.matcher(content);
while (m.find()) {
String name = m.group(1);
String time = m.group(2);
Long msTime = Long.valueOf(time);
result.put(name, msTime);
}
return result;
}
protected static String getFileContent(File file) {
try (FileInputStream s = new FileInputStream(file)) {
return FileUtil.streamToString(s, file.getName()).trim();
} catch (IOException e) {
return "";
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy