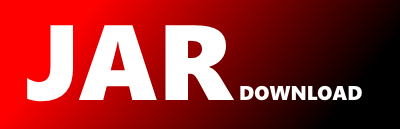
nl.hsac.fitnesse.junit.reportmerge.writer.ChartWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.junit.reportmerge.writer;
import java.io.PrintWriter;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collector;
import java.util.stream.Collectors;
/**
* Helper to use Google Chart.
*/
public class ChartWriter {
protected final PrintWriter pw;
public ChartWriter(PrintWriter pw) {
this.pw = pw;
}
public void writeLoadScriptTag() {
pw.write("");
}
public void writeChartGenerators(List htmls,
BiConsumer> bodyFunction,
String extraJs,
String... chartElements) {
pw.write("");
}
public void writePieChartGenerator(String title,
String chartId,
List values,
Function keyFunction,
Collector groupValueCollector) {
List> sums = sortBy(
values.stream()
.collect(Collectors.groupingBy(
keyFunction,
groupValueCollector))
.entrySet(),
r -> r.getKey());
writePieChartGenerator(title, chartId,
"",
r -> r.getKey(), r -> r.getValue(), sums);
}
public void writePieChartGenerator(String title,
String chartElementId,
String extraOptions,
Function keyFunction,
Function valueFunction,
Iterable groups) {
String dataArray = createDataArray("'Group',''", keyFunction, valueFunction, groups);
pw.write("var ");
pw.write(chartElementId);
pw.write("Data = google.visualization.arrayToDataTable(");
pw.write(dataArray);
pw.write(");");
pw.write("var ");
pw.write(chartElementId);
pw.write("Chart = new google.visualization.PieChart(document.getElementById('");
pw.write(chartElementId);
pw.write("'));");
pw.write(chartElementId);
pw.write("Chart.draw(");
pw.write(chartElementId);
pw.write("Data");
pw.write(",{title:'");
pw.write(title);
pw.write("',sliceVisibilityThreshold:0,is3D:true,pieSliceTextStyle:{color:'black'}");
pw.write(extraOptions);
pw.write("});");
}
public void writeBarChartGenerator(Consumer testResultsWriter,
String title,
String chartElementId,
String extraOptions) {
pw.write("var testResults=");
testResultsWriter.accept(pw);
pw.write(";");
// show graph element when successfully loaded, do this before having it filled so charts API can detect size
writeUnhideElement(chartElementId);
writeBarChartContentGenerator(title, chartElementId, extraOptions);
}
protected void writeLoadAndCallback(List htmls, BiConsumer> bodyFunction) {
pw.write("google.charts.load('current',{'packages':['corechart']});");
pw.write("google.charts.setOnLoadCallback(drawChart);");
pw.write("function drawChart(){");
bodyFunction.accept(this, htmls);
pw.write("};");
}
protected void writeHideElement(String chartElementId) {
pw.write("var ");
pw.write(chartElementId);
pw.write("CurrentStyle = ");
pw.write(chartElementId);
pw.write("Element.getAttribute('style');");
pw.write(chartElementId);
pw.write("Element.setAttribute('style', 'display: none;');");
}
protected void writeVariableForElement(String chartElementId) {
pw.write("var ");
pw.write(chartElementId);
pw.write("Element = document.getElementById('");
pw.write(chartElementId);
pw.write("');");
}
protected void writeUnhideElement(String chartElementId) {
pw.write(chartElementId);
pw.write("Element.setAttribute('style', ");
pw.write(chartElementId);
pw.write("CurrentStyle);");
}
protected void writeBarChartContentGenerator(String title, String chartElementId, String extraOptions) {
pw.write("var ");
pw.write(chartElementId);
pw.write("Array = [['Test','Runtime (ms)']];");
pw.write("var ");
pw.write(chartElementId);
pw.write("Urls = [];");
pw.write("for (var index = 0; index < testResults.length; ++index) {");
pw.write("var testResult = testResults[index];");
pw.write("if (!testResult.overviewPage) {");
pw.write(chartElementId);
pw.write("Array.push([testResult.testName, testResult.time]);");
pw.write(chartElementId);
pw.write("Urls.push(testResult.relativePath);");
pw.write("}}");
pw.write("testResults = null;");
pw.write("var ");
pw.write(chartElementId);
pw.write("Data = google.visualization.arrayToDataTable(");
pw.write(chartElementId);
pw.write("Array);");
pw.write(chartElementId);
pw.write("Array = null;");
pw.write("var ");
pw.write(chartElementId);
pw.write("Chart = new google.visualization.ColumnChart(");
pw.write(chartElementId);
pw.write("Element);");
pw.write(chartElementId);
pw.write("Chart.draw(");
pw.write(chartElementId);
pw.write("Data");
pw.write(",{title:'");
pw.write(title);
pw.write("',bar: {groupWidth: '80%'},legend:{position: 'none'}");
pw.write(extraOptions);
pw.write("});");
pw.write(chartElementId);
pw.write("Data = null;");
writeColumnClickListener(chartElementId);
}
protected void writeColumnClickListener(String chartElementId) {
pw.write("google.visualization.events.addListener(");
pw.write(chartElementId);
pw.write("Chart,'select',function myClickHandler(event) {");
pw.write("var selection = ");
pw.write(chartElementId);
pw.write("Chart.getSelection();");
pw.write("for (var i = 0; i < selection.length; i++) {");
pw.write("var item = selection[i];");
pw.write("if (item.row != null && item.column != null) {");
pw.write("window.location=");
pw.write(chartElementId);
pw.write("Urls[item.row];");
pw.write("}}});");
}
protected String createDataArray(String firstElement, Function keyFunction, Function valueFunction, Iterable groups) {
StringBuilder data = new StringBuilder("[[");
data.append(firstElement);
data.append("],");
groups.forEach(r -> {
data.append("['");
data.append(keyFunction.apply(r));
data.append("',");
data.append(valueFunction.apply(r));
data.append("],");
});
data.append("]");
return data.toString();
}
protected static List sortBy(Collection values, Function function) {
return values.stream().sorted((o1, o2) -> function.apply(o1).compareTo(function.apply(o2)))
.collect(Collectors.toList());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy