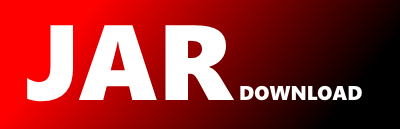
nl.hsac.fitnesse.fixture.slim.CompareFixture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-fixtures Show documentation
Show all versions of hsac-fitnesse-fixtures Show documentation
Fixtures to assist in testing via FitNesse
package nl.hsac.fitnesse.fixture.slim;
import com.sksamuel.diffpatch.DiffMatchPatch;
import nl.hsac.fitnesse.fixture.util.Formatter;
import org.apache.commons.text.StringEscapeUtils;
import java.util.LinkedList;
/**
* Fixture to determine and visualize differences between strings.
*/
public class CompareFixture extends SlimFixture {
private final DiffMatchPatch diffMatchPatch = new DiffMatchPatch();
/**
* Determines difference between two strings.
* @param first first string to compare.
* @param second second string to compare.
* @return HTML of difference between the two.
*/
public String differenceBetweenAnd(String first, String second) {
Formatter whitespaceFormatter = new Formatter() {
@Override
public String format(String value) {
return ensureWhitespaceVisible(value);
}
};
return getDifferencesHtml(first, second, whitespaceFormatter);
}
/**
* Determines difference between two strings, visualizing various forms of whitespace.
* @param first first string to compare.
* @param second second string to compare.
* @return HTML of difference between the two.
*/
public String differenceBetweenExplicitWhitespaceAnd(String first, String second) {
Formatter whitespaceFormatter = new Formatter() {
@Override
public String format(String value) {
return explicitWhitespace(value);
}
};
return getDifferencesHtml(first, second, whitespaceFormatter);
}
protected String getDifferencesHtml(String first, String second, Formatter whitespaceFormatter) {
if (first == null) {
if (second == null) {
return null;
} else {
first = "";
}
} else if (second == null) {
second = "";
}
LinkedList diffs = getDiffs(first, second);
String rootTag = first.startsWith("") && first.endsWith("
")
? "pre"
: "div";
String diffPrettyHtml = diffToHtml(rootTag, diffs, whitespaceFormatter);
if (first.startsWith("") && first.endsWith("
")) {
diffPrettyHtml = diffPrettyHtml.replaceFirst("^", "").replaceFirst("
$", "
");
}
return diffPrettyHtml;
}
/**
* Determines number of differences (substrings that are not equal) between two strings.
* @param first first string to compare.
* @param second second string to compare.
* @return number of different substrings.
*/
public int countDifferencesBetweenAnd(String first, String second) {
if (first == null) {
if (second == null) {
return 0;
} else {
first = "";
}
} else if (second == null) {
second = "";
}
LinkedList"+ cleanFirst + "
").equals(cleanDiff)) {
cleanDiff = "" + first + "
";
} else if (cleanFirst != null && cleanFirst.equals(cleanDiff)) {
cleanDiff = first;
}
}
return cleanDiff;
}
/**
* Determines number of differences (substrings that are not equal) between two strings,
* ignoring differences in whitespace.
* @param first first string to compare.
* @param second second string to compare.
* @return number of different substrings.
*/
public int countDifferencesBetweenIgnoreWhitespaceAnd(String first, String second) {
String cleanFirst = allWhitespaceToSingleSpace(first);
String cleanSecond = allWhitespaceToSingleSpace(second);
return countDifferencesBetweenAnd(cleanFirst, cleanSecond);
}
protected String allWhitespaceToSingleSpace(String value) {
return value != null
? value
// unicode non breaking space to normal space
.replace("\u00A0", " ")
// all sequences of whitespace replaced by single space
.replaceAll("\\s+", " ")
: null;
}
protected String diffToHtml(String rootTag, LinkedList"); } protected String explicitWhitespace(String text) { return text.replace(" ", "·") .replace("\r", "↵") .replace("\n", "¶
") .replace("\t", "→") .replace(" ", "•"); } }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy