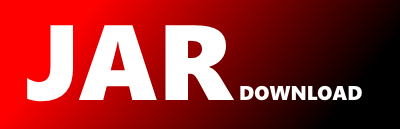
nl.hsac.fitnesse.slimcoverage.SlimScenarioUsage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hsac-fitnesse-plugin Show documentation
Show all versions of hsac-fitnesse-plugin Show documentation
Plugin to add features to a FitNesse installation
package nl.hsac.fitnesse.slimcoverage;
import org.apache.commons.lang3.StringUtils;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class SlimScenarioUsage {
private final Map usagePerPage = new LinkedHashMap();
public SlimScenarioUsagePer getUsageByPage(String pageName) {
if (!usagePerPage.containsKey(pageName)) {
usagePerPage.put(pageName, new SlimScenarioUsagePer(pageName));
}
return usagePerPage.get(pageName);
}
public List getUsage() {
return new ArrayList(usagePerPage.values());
}
public SlimScenarioUsagePer getScenarioUsage() {
SlimScenarioUsagePer result = new SlimScenarioUsagePer("Total per scenario");
for (SlimScenarioUsagePer value : usagePerPage.values()) {
for (Map.Entry entry : value.getUsage().entrySet()) {
result.addUsage(entry.getKey(), entry.getValue());
}
}
return result;
}
public Collection getUnusedScenarios() {
List result = new ArrayList();
for (Map.Entry usage : getScenarioUsage().getUsage().entrySet()) {
if (usage.getValue() < 1) {
result.add(usage.getKey());
}
}
return result;
}
public Collection getUsedScenarios() {
List result = new ArrayList();
for (Map.Entry usage : getScenarioUsage().getUsage().entrySet()) {
if (usage.getValue() > 0) {
result.add(usage.getKey());
}
}
return result;
}
public Collection getOverriddenScenarios() {
Set result = new HashSet();
for (Map.Entry> usage : getOverriddenScenariosPerPage().entrySet()) {
result.addAll(usage.getValue());
}
return result;
}
public Map> getOverriddenScenariosPerPage() {
Map> result = new LinkedHashMap>();
for (Map.Entry value : usagePerPage.entrySet()) {
if (!value.getValue().getOverriddenScenarios().isEmpty()) {
result.put(value.getKey(), value.getValue().getOverriddenScenarios());
}
}
return result;
}
public Map> getPagesUsingScenario() {
Map> result = new LinkedHashMap>();
for (Map.Entry value : usagePerPage.entrySet()) {
String page = value.getKey();
for (Map.Entry entry : value.getValue().getUsage().entrySet()) {
if (entry.getValue() > 0) {
String scenario = entry.getKey();
Collection pagesUsingScenario = getOrCreateCollection(result, scenario);
pagesUsingScenario.add(page);
}
}
}
return result;
}
public Map> getScenariosBySmallestScope() {
Map> result = new LinkedHashMap>();
Map> pagesPerScenario = getPagesUsingScenario();
for (Map.Entry> ppsEntry : pagesPerScenario.entrySet()) {
String scenario = ppsEntry.getKey();
Collection pages = ppsEntry.getValue();
String scope = getLongestSharedPath(pages);
Collection scenariosForScope = getOrCreateCollection(result, scope);
scenariosForScope.add(scenario);
}
return result;
}
private String getLongestSharedPath(Collection pages) {
String result;
if (pages.size() == 1) {
result = pages.iterator().next();
} else {
List pageNames = new ArrayList(pages);
String longestPrefix = StringUtils.getCommonPrefix(pageNames.toArray(new String[pageNames.size()]));
if (longestPrefix.endsWith(".")) {
result = longestPrefix.substring(0, longestPrefix.lastIndexOf("."));
} else {
if (pageNames.contains(longestPrefix)) {
result = longestPrefix;
} else {
int lastDot = longestPrefix.lastIndexOf(".");
result = longestPrefix.substring(0, lastDot);
}
}
}
return result;
}
protected Collection getOrCreateCollection(Map> map, String scope) {
Collection value = map.get(scope);
if (value == null) {
value = new ArrayList();
map.put(scope, value);
}
return value;
}
public String toString() {
return "ScenarioUsage: " + usagePerPage;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy